
Still won't work
Dependencies: mbed wave_player 4DGL-uLCD-SE MMA8452
hardware.cpp
00001 // This header has all the (extern) declarations of the globals. 00002 // "extern" means "this is instantiated somewhere, but here's what the name 00003 // means. 00004 #include "globals.h" 00005 00006 #include "hardware.h" 00007 00008 // We need to actually instantiate all of the globals (i.e. declare them once 00009 // without the extern keyword). That's what this file does! 00010 00011 // Hardware initialization: Instantiate all the things! 00012 uLCD_4DGL uLCD(p9,p10,p11); // LCD Screen (tx, rx, reset) 00013 //SDFileSystem sd(p5, p6, p7, p8, "sd"); // SD Card(mosi, miso, sck, cs) 00014 Serial pc(USBTX,USBRX); // USB Console (tx, rx) 00015 MMA8452 acc(p28, p27, 100000); // Accelerometer (sda, sdc, rate) 00016 DigitalIn button1(p21); // Pushbuttons (pin) 00017 DigitalIn button2(p22); 00018 DigitalIn button3(p23); 00019 AnalogOut DACout(p18); // Speaker (pin) 00020 PwmOut speaker(p26); 00021 wave_player waver(&DACout); 00022 00023 // Some hardware also needs to have functions called before it will set up 00024 // properly. Do that here. 00025 int hardware_init() 00026 { 00027 // Crank up the speed 00028 uLCD.baudrate(3000000); 00029 pc.baud(115200); 00030 00031 //Initialize pushbuttons 00032 button1.mode(PullUp); 00033 button2.mode(PullUp); 00034 button3.mode(PullUp); 00035 00036 return ERROR_NONE; 00037 } 00038 00039 GameInputs read_inputs() 00040 { 00041 GameInputs in; 00042 in.b1 = !button1; 00043 in.b2 = !button2; 00044 in.b3 = !button3; 00045 acc.readXYZGravity(&in.ax,&in.ay,&in.az); 00046 return in; 00047 }
Generated on Fri Aug 19 2022 16:39:02 by
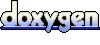