A simple WIP that logs data from a Grove sensor, and can send and receive information over USB and SMS.
Dependencies: DHT DS_1337 SDFileSystem USBDevice mbed
measurementhandler.h
00001 #ifndef MEASUREMENTHANDLER_H 00002 #define MEASUREMENTHANDLER_H 00003 00004 #include "AbstractHandler.h" 00005 #include "GroveDht22.h" 00006 #include "config.h" 00007 #ifdef ENABLE_GPRS_TESTING 00008 #include "GprsHandler.h" 00009 #endif 00010 00011 class SdHandler; 00012 class UsbComms; 00013 00014 00015 /*! 00016 * \brief The MeasurementHandler class forms the link between data generation and data output, and stores settings. 00017 * 00018 * Receives requests from \a GroveDht22 when a new measurement has been taken or error has occurred. This handler then 00019 * sends that information on to \a UsbComms for printing that information to terminal and \a SdHandler for printing 00020 * that information to the CSV data file. 00021 * 00022 * This handler also determines if the necessary conditions have been met to send an SMS. This is based on last measurement, 00023 * the set alert threshold, and time since last alert was sent. An SMS is sent using \a GprsHandler. 00024 * 00025 * Other requests from inputs asking for current measurement states (such as the latest measurement) may come from \a UsbComms 00026 * or \a GprsHandler. The string inspection and matching is handled here, and responses sent to the data outputs. 00027 * 00028 * 00029 * Flashes LED4 constantly to inform that normal operation is occurring. 00030 */ 00031 class MeasurementHandler : public AbstractHandler 00032 { 00033 public: 00034 #ifdef ENABLE_GPRS_TESTING 00035 MeasurementHandler(SdHandler *_sd, UsbComms *_usb, GprsHandler *_gprs, MyTimers *_timer); 00036 #else 00037 MeasurementHandler(SdHandler *_sd, UsbComms *_usb, MyTimers *_timer); 00038 #endif 00039 00040 void run(); 00041 00042 void setRequest(int request, void *data = 0); 00043 00044 Dht22Result lastResult() const { return m_lastResult; } 00045 00046 enum request_t { 00047 measreq_MeasReqNone, ///< No request (for tracking what the last request was, this is initial value for that) 00048 measreq_DhtResult, ///< Dht22 returned with a result 00049 measreq_DhtError, ///< Dht22 returned with an error 00050 #ifdef ENABLE_GPRS_TESTING 00051 measreq_Status, ///< We got an SMS asking for the status (time, last error, last result) 00052 #endif 00053 }; 00054 00055 private: 00056 SdHandler *m_sd; ///< Reference to write to SD card 00057 UsbComms *m_usb; ///< Reference to write to USB serial port 00058 00059 #ifdef ENABLE_GPRS_TESTING 00060 GprsHandler *m_gprs; ///< Reference to write to GPRS (SMS) 00061 #endif 00062 00063 Dht22Result m_lastResult; ///< Copy of the last result that came from Dht22 00064 int m_lastError; ///< Copy of the last error that came from Dht22 00065 00066 #ifdef ENABLE_GPRS_TESTING 00067 char m_lastSender[GPRS_RECIPIENTS_MAXLEN]; ///< The last sender of an SMS 00068 #endif 00069 00070 bool m_flashOn; ///< LED is currently on when true 00071 00072 enum mode_t{ 00073 meas_Start, ///< Set up the state machine 00074 meas_CheckRequest, ///< Check request register 00075 00076 #ifdef ENABLE_GPRS_TESTING 00077 meas_PostStateSMS, ///< Send an SMS of the last result and state 00078 #endif 00079 meas_PostResult, ///< Write the last Grove result to SD and USB 00080 meas_PostError, ///< Write the last Grove error to USB (and in future, SD syslog) 00081 00082 meas_FlashTimer, ///< Flash an LED on and off so user knows device is still running 00083 00084 meas_WaitError ///< Lots of fails, wait for a while 00085 }; 00086 mode_t mode; 00087 00088 request_t m_lastRequest; ///< tracks if result or error was the last request 00089 00090 uint8_t m_requestRegister; ///< contains the current pending requests as bitwise flags 00091 00092 }; 00093 00094 #endif // MEASUREMENTHANDLER_H
Generated on Tue Jul 12 2022 23:07:44 by
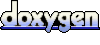