A simple WIP that logs data from a Grove sensor, and can send and receive information over USB and SMS.
Dependencies: DHT DS_1337 SDFileSystem USBDevice mbed
main.cpp
00001 /*! 00002 * Project: Humidity SMS Alert 00003 * Author: Joseph Radford 00004 * Date start: January 2016 00005 * Date mod: 00006 * Target: Arch GPRS V2 00007 * Peripherals: SD Card 00008 * SIM Card 00009 * Grove Humidity and Temperature Sensor Pro (DHT22 on a breakout board) 00010 * 00011 * 00012 * Takes intermittent readings from the humidity temperature sensor. These are written to a timestamped CSV file 00013 * on the SD card and printed over serial if there is a connection to a terminal window (Screen on Linux, Putty on Windows, etc) 00014 * 00015 * When conditions have been met, an SMS alert is sent notifying the recipient of the last sensor readings. 00016 * 00017 * States can be requested, and configurations changed, by sending requests over USB serial or SMS. 00018 * 00019 * Inputs: 00020 * \a GroveDht22 00021 * 00022 * Outputs: 00023 * \a SdHandler 00024 * 00025 * 00026 * Inputs and outputs: 00027 * \a GprsHandler 00028 * \a UsbComms 00029 * 00030 * Linker: 00031 * \a MeasurementHandler 00032 * 00033 * 10/04/2016 v0.0.1 - Writes timestamped humidity data to an SD card 00034 * 00035 * Issues: 00036 * Stops communicating over USB after ~10 mins. 00037 * Will not work if USB not present. 00038 * Will not work if SD card is not present 00039 * GPRS yet to be implemented 00040 * RTC is set to 01/01/2000 00:00:00 at startup. No way of setting it yet. 00041 */ 00042 00043 #include "config.h" 00044 00045 #include "mbed.h" 00046 #include "math.h" 00047 00048 // Clocks and timers 00049 #include "DS1337.h" 00050 #include "rtc.h" 00051 #include "timers.h" 00052 00053 // Handlers 00054 #include "Handlers/GroveDht22.h" 00055 #include "Handlers/UsbComms.h" 00056 #include "Handlers/SdHandler.h" 00057 #include "Handlers/measurementhandler.h" 00058 00059 #ifdef ENABLE_GPRS_TESTING 00060 #include "Handlers/GprsHandler.h" 00061 #endif 00062 00063 /* Declare hardware classes */ 00064 00065 DigitalOut myled1(LED2); ///< startup, and USB handler when writing occurring (right most) 00066 DigitalOut myled2(LED3); ///< SD handler - on when buffer pending, off when written. If error, will be on as buffer won't clear 00067 DigitalOut myled3(LED4); ///< GPRS handler information 00068 DigitalOut myled4(LED1); ///< measurement handler flashes, periodically to inform running (left most) 00069 00070 //DigitalOut myenable(P0_6); 00071 00072 DS1337 * RTC_DS1337; ///< RTC interface class 00073 00074 I2C i2c(P0_5, P0_4); ///< I2C comms - this is required by the RTC - do NOT change the name of the instance 00075 00076 00077 /* Declare helpers */ 00078 MyTimers *mytimer; ///< declare timers class - required for other classes to use timers (do not change name) 00079 00080 00081 /* Declare handlers */ 00082 GroveDht22 *grove; ///< grove humidity sensor handler class 00083 UsbComms *usbcomms; ///< reading and writing to usb 00084 SdHandler *sdhandler; ///< Writing data to a file on SD 00085 MeasurementHandler *measure; ///< Handle measurements, and route data to the user and user to configuration 00086 00087 #ifdef ENABLE_GPRS_TESTING 00088 GprsHandler * gprs; ///< Reading and writing to the SIM900 00089 #endif 00090 00091 #ifdef ENABLE_GPRS_TESTING 00092 #define NUM_HANDLERS 5 00093 #else 00094 #define NUM_HANDLERS 4 00095 #endif 00096 00097 #define PROGRAM_TITLE "Arch GPRS V2 Alert and Request" 00098 #define PROGRAM_INFO "v0.0.1, released 09/04/2016" 00099 00100 /*! 00101 * \brief main pulses LED1, creates all classes, pulses LED1, then runs through all handlers forever 00102 * \return 00103 */ 00104 int main() 00105 { 00106 /* start up sequence, so we know we reached main */ 00107 myled1 = 1; 00108 wait(0.2); 00109 myled1 = 0; 00110 wait(0.2); 00111 myled1 = 1; 00112 wait(0.2); 00113 myled1 = 0; 00114 wait(1); 00115 myled1 = 1; 00116 wait(0.2); 00117 myled1 = 0; 00118 wait(0.2); 00119 myled1 = 1; 00120 wait(0.2); 00121 myled1 = 0; 00122 wait(1); 00123 00124 /* Declare all classes */ 00125 00126 // create MyTimers object, which can be used for waiting in handlers 00127 mytimer = new MyTimers(); 00128 00129 // RTC interface class 00130 RTC_DS1337 = new DS1337(); 00131 attach_rtc(&my_rtc_read, &my_rtc_write, &my_rtc_init, &my_rtc_enabled); 00132 00133 // set the time to the start of the year 2000, by default 00134 // this will be removed when time can be set, so that time is not reset each time the program starts. 00135 tm timeinfo; 00136 timeinfo.tm_hour = 0; timeinfo.tm_min = 0; timeinfo.tm_sec = 0; 00137 timeinfo.tm_year = (2001 - 1900); timeinfo.tm_mon = 0; timeinfo.tm_mday = 1; 00138 set_time(mktime(&timeinfo)); 00139 00140 // declare usbcomms 00141 usbcomms = new UsbComms(mytimer); 00142 00143 // declare sd handler 00144 sdhandler = new SdHandler(mytimer); 00145 00146 #ifdef ENABLE_GPRS_TESTING 00147 gprs = new GprsHandler(mytimer, usbcomms); 00148 measure = new MeasurementHandler(sdhandler, usbcomms, gprs, mytimer); 00149 #else 00150 measure = new MeasurementHandler(sdhandler, usbcomms, mytimer); 00151 #endif 00152 00153 // declare grove 00154 grove = new GroveDht22(measure, mytimer); 00155 00156 // put the handlers in an array for easy reference 00157 #ifdef ENABLE_GPRS_TESTING 00158 AbstractHandler* handlers[] = {grove, usbcomms, sdhandler, measure, gprs}; 00159 #else 00160 AbstractHandler* handlers[] = {grove, usbcomms, measure, sdhandler}; 00161 #endif 00162 00163 // send startup message to terminal 00164 usbcomms->setRequest(UsbComms::usbreq_PrintToTerminalTimestamp, (char*)PROGRAM_TITLE); 00165 usbcomms->setRequest(UsbComms::usbreq_PrintToTerminalTimestamp, (char*)PROGRAM_INFO); 00166 usbcomms->setRequest(UsbComms::usbreq_PrintToTerminalTimestamp, (char*)"\r\n******************\r\n"); 00167 00168 // flush output 00169 fflush(stdout); 00170 00171 00172 /* Pulse LED1 again to signify start up was successful */ 00173 myled1 = 1; 00174 wait(0.2); 00175 myled1 = 0; 00176 wait(0.2); 00177 myled1 = 1; 00178 wait(0.2); 00179 myled1 = 0; 00180 wait(1); 00181 myled1 = 1; 00182 wait(0.2); 00183 myled1 = 0; 00184 wait(0.2); 00185 myled1 = 1; 00186 wait(0.2); 00187 myled1 = 0; 00188 wait(1); 00189 00190 00191 while(1) 00192 { 00193 // perform run functions for all handlers, one after the other 00194 for (int i = 0; i < NUM_HANDLERS; i++) { 00195 handlers[i]->run(); 00196 } 00197 } // while 00198 00199 for (int i = 0; i < NUM_HANDLERS; i++) { 00200 delete handlers[i]; 00201 } 00202 } // main 00203 00204 00205
Generated on Tue Jul 12 2022 23:07:44 by
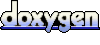