A simple WIP that logs data from a Grove sensor, and can send and receive information over USB and SMS.
Dependencies: DHT DS_1337 SDFileSystem USBDevice mbed
circbuff.cpp
00001 #include "circbuff.h" 00002 00003 CircBuff::CircBuff(uint16_t buffSize) 00004 { 00005 // set up the buffer with parsed size 00006 m_buffSize = buffSize; 00007 m_buf = new unsigned char [m_buffSize]; 00008 for (int i = 0; i < m_buffSize; i++) { 00009 m_buf[i] = 0; 00010 } 00011 00012 // init indexes 00013 m_start = 0; 00014 m_end = 0; 00015 } 00016 00017 CircBuff::~CircBuff() 00018 { 00019 delete m_buf; 00020 } 00021 00022 void CircBuff::putc(unsigned char c) 00023 { 00024 // get the remaining space left in the buffer by checking end and start idx 00025 uint16_t remSize = remainingSize(); 00026 00027 // check we have enough room for the new array passed in 00028 if (remSize == 0) { 00029 return; 00030 } 00031 00032 // else copy the byte in 00033 m_buf[m_end++] = c; 00034 if (m_end == m_buffSize) { 00035 m_end = 0; // wrap around 00036 } 00037 00038 } 00039 00040 void CircBuff::add(unsigned char *s) 00041 { 00042 // check if can write? How to check if we have connected. 00043 uint16_t sSize = 0, i = 0, j = 0; 00044 00045 // get the length of the passed array (it should be null terminated, but include max buffer size for caution) 00046 for (sSize = 0; (sSize < m_buffSize) && (s[sSize] != 0); sSize++); 00047 00048 // get the remaining space left in the buffer by checking end and start idx 00049 uint16_t remSize = remainingSize(); 00050 00051 // check we have enough room for the new array passed in 00052 if (sSize > remSize) { 00053 return; 00054 } 00055 00056 // copy the array in 00057 for (i = 0; i < sSize; i++) { 00058 m_buf[m_end++] = s[i]; 00059 if (m_end == m_buffSize) { 00060 m_end = 0; // wrap around 00061 } 00062 } 00063 } 00064 00065 uint16_t CircBuff::remainingSize() 00066 { 00067 // get the remaining space left in the buffer by checking end and start idx 00068 uint16_t retval = 0; 00069 if (m_start == m_end) { 00070 retval = m_buffSize; 00071 } 00072 else if (m_start < m_end) { 00073 // the distance between the start and end point 00074 // subtract that from the total length of the buffer 00075 retval = m_buffSize - (m_end - m_start); 00076 } 00077 else { 00078 // the amount of space left is whatever is between the end point and the start point 00079 retval = m_start - m_end; 00080 } 00081 return retval; 00082 } 00083 00084 uint16_t CircBuff::read(unsigned char *s, uint16_t len) 00085 { 00086 if (m_start == m_end) { 00087 return 0; // there is nothing stored in the circular buffer 00088 } 00089 00090 // start copying the desired amount over 00091 for (int i = 0; i < len; i++) { 00092 s[i] = m_buf[m_start++]; 00093 00094 if (m_start == m_buffSize) { 00095 m_start = 0; // wrap start pointer 00096 } 00097 00098 if (m_start == m_end) { 00099 s[++i] = 0; 00100 return (i); // we have reached the end of the buffer 00101 } 00102 } 00103 return len; 00104 } 00105 00106
Generated on Tue Jul 12 2022 23:07:44 by
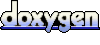