A simple WIP that logs data from a Grove sensor, and can send and receive information over USB and SMS.
Dependencies: DHT DS_1337 SDFileSystem USBDevice mbed
UsbComms.cpp
00001 #include "UsbComms.h" 00002 #include "mbed.h" 00003 00004 #include "USBDevice.h" 00005 #include "USBSerial.h" 00006 00007 #include "circbuff.h" 00008 00009 #define USB_CIRC_BUFF 256 00010 00011 extern DigitalOut myled1; // this led is used to notify state of USB comms 00012 00013 UsbComms::UsbComms(MyTimers *_timer) : AbstractHandler(_timer) 00014 { 00015 mode = usb_Start; 00016 00017 m_circBuff = new CircBuff(USB_CIRC_BUFF); 00018 00019 // Declare serial port for communication with PC over USB 00020 _serial = new USBSerial; 00021 } 00022 00023 UsbComms::~UsbComms() 00024 { 00025 delete _serial; 00026 delete m_circBuff; 00027 } 00028 00029 void UsbComms::run() 00030 { 00031 unsigned char temp; 00032 switch(mode) { 00033 case usb_Start: 00034 mode = usb_CheckInput; 00035 break; 00036 case usb_CheckInput: 00037 if (_serial->readable()) { 00038 _serial->getc(); 00039 // todo: do something with it! this is where config events are started 00040 // uncomment to print a message confirming input 00041 // _serial->writeBlock((unsigned char*)"getc\r\n", 6); 00042 //mode = usb_WaitForInput; 00043 mode = usb_CheckOutput; 00044 } else { 00045 mode = usb_CheckOutput; 00046 } 00047 break; 00048 case usb_CheckOutput: 00049 if (m_circBuff->dataAvailable() && _serial->writeable()) { 00050 // ensure only 64 bytes or less are written at a time 00051 unsigned char s[TX_USB_MSG_MAX]; 00052 int len = m_circBuff->read(s, TX_USB_MSG_MAX); 00053 _serial->writeBlock((unsigned char*)s, len); 00054 myled1 = 1; 00055 00056 } else { 00057 myled1 = 0; 00058 } 00059 mode = usb_CheckInput; 00060 break; 00061 } 00062 } 00063 00064 void UsbComms::setRequest(int request, void *data) 00065 { 00066 request_t req = (request_t )request; 00067 00068 switch (req) { 00069 case usbreq_PrintToTerminal: 00070 printToTerminal((char*)data); 00071 break; 00072 case usbreq_PrintToTerminalTimestamp: 00073 printToTerminalEx((char*)data); 00074 break; 00075 } 00076 } 00077 00078 void UsbComms::printToTerminal(char *s) 00079 { 00080 // simply add this string to the circular buffer 00081 m_circBuff->add((unsigned char*)s); 00082 } 00083 00084 // print the message, but prepend it with a standard timestamp 00085 // YYYYMMDD HHMMSS: 00086 // 01234567890123456 00087 void UsbComms::printToTerminalEx(char *s) 00088 { 00089 unsigned char tempBuff[TX_USB_BUFF_SIZE]; 00090 // this won't work! needs to be a circular buffer... 00091 uint16_t sSize = 0, i = 0, j = 0; 00092 00093 // get the length of the passed array (it should be null terminated, but include max buffer size for caution) 00094 for (sSize = 0; (sSize < TX_USB_BUFF_SIZE) && (s[sSize] != 0); sSize++); 00095 00096 00097 time_t _time = time(NULL); // get the seconds since dawn of time 00098 00099 // extract time_t to time info struct 00100 struct tm * timeinfo = localtime(&_time); 00101 00102 // print the formatted timestamp at the start of terminalBuffer 00103 sprintf((char*)&tempBuff[0], "%04d%02d%02d %02d%02d%02d:", (timeinfo->tm_year + 1900), 00104 (timeinfo->tm_mon + 1), 00105 timeinfo->tm_mday, 00106 timeinfo->tm_hour, 00107 timeinfo->tm_min, 00108 timeinfo->tm_sec); 00109 00110 00111 // copy the string into the remainder of the terminal buffer 00112 for (i = 16; i < (16 + sSize); i++) { 00113 tempBuff[i] = s[j++]; 00114 } 00115 00116 // add a carriage return and new line to the output buffer 00117 tempBuff[i++] = '\r'; 00118 tempBuff[i++] = '\n'; 00119 tempBuff[i++] = 0; 00120 00121 // add it to the circular buffer 00122 m_circBuff->add(tempBuff); 00123 } 00124 00125
Generated on Tue Jul 12 2022 23:07:44 by
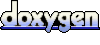