A simple WIP that logs data from a Grove sensor, and can send and receive information over USB and SMS.
Dependencies: DHT DS_1337 SDFileSystem USBDevice mbed
SdHandler.h
00001 #ifndef __SD_HANDLER_H__ 00002 #define __SD_HANDLER_H__ 00003 00004 #include "SDFileSystem.h" 00005 #include "AbstractHandler.h" 00006 00007 class CircBuff; 00008 00009 /*! 00010 * \brief The SdHandler class writes messages to file and handles SD card status 00011 * 00012 * A data CSV file is written with timestamps and the result from the GroveDht22. 00013 * A system log, not yet fully implemented, tracks system events for debugging purposes. 00014 */ 00015 class SdHandler : public AbstractHandler 00016 { 00017 public: 00018 SdHandler(MyTimers * _timer); 00019 ~SdHandler(); 00020 00021 void run(); 00022 00023 void setRequest(int request, void *data = 0); 00024 00025 bool sdOk(); 00026 00027 enum request_t { 00028 sdreq_SdNone, ///< to init 00029 sdreq_LogData, ///< Send struct containing a result and timestamp. This turns it into a line in a csv file 00030 sdreq_LogSystem ///< write raw string to system logging file (errors, events, etc) 00031 }; 00032 00033 private: 00034 SDFileSystem * m_sdfs; 00035 FILE * m_data; 00036 FILE * m_syslog; 00037 00038 enum mode_t{ 00039 sd_Start, ///< Set up the state machine 00040 sd_CheckSysLogBuffer, ///< See if any data should be written to the log file 00041 sd_CheckDataLogBuffer, ///< See if any data should be written to the data file 00042 00043 sd_WaitError ///< Error. wait for a while 00044 }; 00045 mode_t mode; 00046 00047 request_t m_lastRequest; 00048 00049 // helpers 00050 void csvStart(time_t time); 00051 void csvData(const char * s, int len); 00052 void csvEnd(); 00053 void logEvent(const char * s); 00054 00055 CircBuff *m_dataLogBuff; ///< Data waiting to be written to the data CSV file 00056 CircBuff *m_sysLogBuff; ///< Data waiting to be written to the system log file (not yet implemented) 00057 }; 00058 00059 #endif // __SD_HANDLER_H__
Generated on Tue Jul 12 2022 23:07:44 by
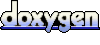