my version with changed conversion between duration units
Fork of BLE_API by
UUID.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef __UUID_H__ 00018 #define __UUID_H__ 00019 00020 #include <stdint.h> 00021 #include <string.h> 00022 00023 #include "blecommon.h" 00024 00025 class UUID { 00026 public: 00027 enum UUID_Type_t { 00028 UUID_TYPE_SHORT = 0, // Short BLE UUID 00029 UUID_TYPE_LONG = 1 // Full 128-bit UUID 00030 }; 00031 00032 typedef uint16_t ShortUUIDBytes_t; 00033 00034 static const unsigned LENGTH_OF_LONG_UUID = 16; 00035 typedef uint8_t LongUUIDBytes_t[LENGTH_OF_LONG_UUID]; 00036 00037 public: 00038 /** 00039 * Creates a new 128-bit UUID 00040 * 00041 * @note The UUID is a unique 128-bit (16 byte) ID used to identify 00042 * different service or characteristics on the BLE device. 00043 * 00044 * @param longUUID 00045 * The 128-bit (16-byte) UUID value, MSB first (big-endian). 00046 */ 00047 UUID(const LongUUIDBytes_t longUUID) : type(UUID_TYPE_LONG), baseUUID(), shortUUID(0) { 00048 setupLong(longUUID); 00049 } 00050 00051 /** 00052 * Creates a new 16-bit UUID 00053 * 00054 * @note The UUID is a unique 16-bit (2 byte) ID used to identify 00055 * different service or characteristics on the BLE device. 00056 * 00057 * For efficiency, and because 16 bytes would take a large chunk of the 00058 * 27-byte data payload length of the Link Layer, the BLE specification adds 00059 * two additional UUID formats: 16-bit and 32-bit UUIDs. These shortened 00060 * formats can be used only with UUIDs that are defined in the Bluetooth 00061 * specification (i.e., that are listed by the Bluetooth SIG as standard 00062 * Bluetooth UUIDs). 00063 * 00064 * To reconstruct the full 128-bit UUID from the shortened version, insert 00065 * the 16-bit short value (indicated by xxxx, including leading zeros) into 00066 * the Bluetooth Base UUID: 00067 * 00068 * 0000xxxx-0000-1000-8000-00805F9B34FB 00069 * 00070 * @note Shortening is not available for UUIDs that are not derived from the 00071 * Bluetooth Base UUID. Such non-standard UUIDs are commonly called 00072 * vendor-specific UUIDs. In these cases, you’ll need to use the full 00073 * 128-bit UUID value at all times. 00074 * 00075 * @note we don't yet support 32-bit shortened UUIDs. 00076 */ 00077 UUID(ShortUUIDBytes_t shortUUID) : type(UUID_TYPE_SHORT), baseUUID(), shortUUID(shortUUID) { 00078 /* empty */ 00079 } 00080 00081 UUID(const UUID &source) { 00082 type = source.type; 00083 shortUUID = source.shortUUID; 00084 memcpy(baseUUID, source.baseUUID, LENGTH_OF_LONG_UUID); 00085 } 00086 00087 UUID(void) : type(UUID_TYPE_SHORT), shortUUID(BLE_UUID_UNKNOWN) { 00088 /* empty */ 00089 } 00090 00091 /** 00092 * Fill in a 128-bit UUID; this is useful when UUID isn't known at the time of object construction. 00093 */ 00094 void setupLong(const LongUUIDBytes_t longUUID) { 00095 type = UUID_TYPE_LONG; 00096 memcpy(baseUUID, longUUID, LENGTH_OF_LONG_UUID); 00097 shortUUID = (uint16_t)((longUUID[2] << 8) | (longUUID[3])); 00098 } 00099 00100 public: 00101 UUID_Type_t shortOrLong(void) const {return type; } 00102 const uint8_t *getBaseUUID(void) const { 00103 if (type == UUID_TYPE_SHORT) { 00104 return (const uint8_t*)&shortUUID; 00105 } else { 00106 return baseUUID; 00107 } 00108 } 00109 00110 ShortUUIDBytes_t getShortUUID(void) const {return shortUUID;} 00111 uint8_t getLen(void) const { 00112 return ((type == UUID_TYPE_SHORT) ? sizeof(ShortUUIDBytes_t) : LENGTH_OF_LONG_UUID); 00113 } 00114 00115 bool operator== (const UUID &other) const { 00116 if ((this->type == UUID_TYPE_SHORT) && (other.type == UUID_TYPE_SHORT) && 00117 (this->shortUUID == other.shortUUID)) { 00118 return true; 00119 } 00120 00121 if ((this->type == UUID_TYPE_LONG) && (other.type == UUID_TYPE_LONG) && 00122 (memcmp(this->baseUUID, other.baseUUID, LENGTH_OF_LONG_UUID) == 0)) { 00123 return true; 00124 } 00125 00126 return false; 00127 } 00128 00129 bool operator!= (const UUID &other) const { 00130 return !(*this == other); 00131 } 00132 00133 private: 00134 UUID_Type_t type; // UUID_TYPE_SHORT or UUID_TYPE_LONG 00135 LongUUIDBytes_t baseUUID; /* the base of the long UUID (if 00136 * used). Note: bytes 12 and 13 (counting from LSB) 00137 * are zeroed out to allow comparison with other long 00138 * UUIDs which differ only in the 16-bit relative 00139 * part.*/ 00140 ShortUUIDBytes_t shortUUID; // 16 bit uuid (byte 2-3 using with base) 00141 }; 00142 00143 #endif // ifndef __UUID_H__
Generated on Wed Jul 13 2022 18:08:30 by
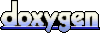