example using DS3234 RTC connected to BBC micro:bit using SPI
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 #include "MicroBit.h" 00027 #include "SparkFunDS3234RTC.h" 00028 00029 MicroBit uBit; 00030 DS3234 rtcDS3234; 00031 00032 int mode = 0; 00033 bool displaying = false; 00034 bool interruptLoop = false; 00035 uint8_t sec, minute, hour, day, date, month, year; 00036 00037 void displayTime(){ 00038 rtcDS3234.update(); 00039 uBit.display.scroll(rtcDS3234.hour()); 00040 uBit.display.scroll(":"); 00041 uBit.display.scroll(rtcDS3234.minute()); 00042 uBit.display.scroll(":"); 00043 uBit.display.scroll(rtcDS3234.second()); 00044 } 00045 00046 void displayLoop(){ 00047 if (!displaying) { 00048 while (!interruptLoop) { 00049 displaying = true; 00050 displayTime(); 00051 uBit.sleep(60000); 00052 } 00053 interruptLoop = false; 00054 } 00055 } 00056 00057 void onButtonB(MicroBitEvent e) 00058 { 00059 switch(mode) { 00060 case 0: 00061 displayTime(); 00062 break; 00063 case 1: 00064 ++year; 00065 break; 00066 case 2: 00067 ++month; 00068 break; 00069 case 3: 00070 ++date; 00071 break; 00072 case 4: 00073 ++day; 00074 break; 00075 case 5: 00076 ++hour; 00077 break; 00078 case 6: 00079 ++minute; 00080 break; 00081 } 00082 } 00083 00084 void onButtonA(MicroBitEvent e) 00085 { 00086 switch(mode) { 00087 case 0: 00088 displayLoop(); 00089 break; 00090 case 1: 00091 --year; 00092 break; 00093 case 2: 00094 --month; 00095 break; 00096 case 3: 00097 --date; 00098 break; 00099 case 4: 00100 --day; 00101 break; 00102 case 5: 00103 --hour; 00104 break; 00105 case 6: 00106 --minute; 00107 break; 00108 } 00109 } 00110 00111 void onButtonAB(MicroBitEvent e) 00112 { 00113 switch(++mode) { 00114 case 1: 00115 rtcDS3234.update(); 00116 uBit.display.scroll("y"); 00117 year = rtcDS3234.year(); 00118 uBit.display.scroll(year); 00119 break; 00120 case 2: 00121 uBit.display.scroll("m"); 00122 month = rtcDS3234.month(); 00123 uBit.display.scroll(month); 00124 break; 00125 case 3: 00126 uBit.display.scroll("d"); 00127 date = rtcDS3234.date(); 00128 uBit.display.scroll(date); 00129 break; 00130 case 4: 00131 uBit.display.scroll("wd"); 00132 day = rtcDS3234.day(); 00133 uBit.display.scroll(day); 00134 break; 00135 case 5: 00136 uBit.display.scroll("h"); 00137 hour = rtcDS3234.hour(); 00138 uBit.display.scroll(hour); 00139 break; 00140 case 6: 00141 uBit.display.scroll("m"); 00142 minute = rtcDS3234.minute(); 00143 uBit.display.scroll(minute); 00144 break; 00145 default: 00146 mode = 0; 00147 sec = 0; 00148 rtcDS3234.setTime(sec, minute, hour, day, date, month, year); 00149 uBit.display.scroll(date); 00150 uBit.display.scroll("."); 00151 uBit.display.scroll(month); 00152 uBit.display.scroll("."); 00153 uBit.display.scroll(year); 00154 uBit.display.scroll(" "); 00155 displayTime(); 00156 } 00157 } 00158 00159 int main() 00160 { 00161 // Initialise the micro:bit runtime. 00162 uBit.init(); 00163 00164 // Insert your code here! 00165 uBit.display.scroll("init"); 00166 00167 rtcDS3234.begin(&uBit.io.P16, &uBit); 00168 uBit.messageBus.listen(MICROBIT_ID_BUTTON_A, MICROBIT_BUTTON_EVT_CLICK, onButtonA); 00169 uBit.messageBus.listen(MICROBIT_ID_BUTTON_B, MICROBIT_BUTTON_EVT_CLICK, onButtonB); 00170 uBit.messageBus.listen(MICROBIT_ID_BUTTON_AB, MICROBIT_BUTTON_EVT_CLICK, onButtonAB); 00171 00172 displayTime(); 00173 00174 release_fiber(); 00175 } 00176
Generated on Wed Jul 20 2022 07:11:22 by
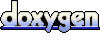