
Hello World example program for the OV528 Camera Module
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "CameraOV528.h" 00003 #include "FATFileSystem.h" 00004 #include "SDBlockDevice.h" 00005 #include <stdio.h> 00006 00007 CameraOV528 camera(D1, D0); // DX, RX 00008 SDBlockDevice bd(PTE3, PTE1, PTE2, PTE4); // MOSI, MISO, SCLK, CS 00009 FATFileSystem fs("fs"); 00010 EventQueue queue(32 * EVENTS_EVENT_SIZE); 00011 Thread t; 00012 DigitalOut green_led(LED_GREEN); 00013 DigitalOut red_led(LED_RED); 00014 InterruptIn btn(SW2); 00015 int picture_count; 00016 00017 int format_fs(){ 00018 int error = 0; 00019 printf("Welcome to the filesystem example.\r\n" 00020 "Formatting a FAT, RAM-backed filesystem. "); 00021 error = FATFileSystem::format(&bd); 00022 if (error) 00023 printf("Failure. %d\r\n", error); 00024 else 00025 printf("done.\r\n"); 00026 return error; 00027 } 00028 00029 int mount_fs() { 00030 printf("Mounting the filesystem on \"/fs\". "); 00031 int error = fs.mount(&bd); 00032 if (error) 00033 printf("Failure. %d\r\n", error); 00034 else 00035 printf("done.\r\n"); 00036 return error; 00037 } 00038 00039 void init_fs() { 00040 if (mount_fs()) { 00041 format_fs(); 00042 mount_fs(); 00043 } 00044 } 00045 00046 void swap_led() { 00047 green_led = !green_led; 00048 red_led = !red_led; 00049 } 00050 00051 void take_and_store_photo() { 00052 // Change LED to Red (for take_and_store_photo duration) 00053 swap_led(); 00054 00055 // Take and store the picture 00056 if(camera.take_picture() == 0) { 00057 printf("Picture taken.\r\n"); 00058 00059 uint32_t size = camera.get_picture_size(); 00060 uint8_t* data_buff = (uint8_t*)malloc(size); 00061 uint32_t bytes_read = camera.read_picture_data(data_buff, size); 00062 char filename[30]; 00063 sprintf(filename, "/fs/img%d.jpg", picture_count++); 00064 printf("Opening a new file, %s.\r\n", filename); 00065 00066 FILE* fd = fopen(filename, "wb"); 00067 fwrite(data_buff, sizeof(uint8_t), bytes_read, fd); 00068 fclose(fd); 00069 00070 printf("Picture saved.\r\n\n"); 00071 00072 free(data_buff); 00073 } 00074 else { 00075 printf("Failed to take photo.\r\n\n"); 00076 } 00077 00078 // Change LED to Green 00079 swap_led(); 00080 } 00081 00082 void rise_handler(void) { 00083 // Add the take_and_store_photo event to the queue 00084 queue.call(take_and_store_photo); 00085 } 00086 00087 int main() { 00088 // Initialize LED to Green 00089 red_led = !red_led; 00090 00091 // Initialize picture count to zero 00092 picture_count = 0; 00093 00094 // Initialize FATFileSystem and Camera 00095 init_fs(); 00096 camera.powerup(); 00097 00098 // Start the event queue's dispatch thread 00099 t.start(callback(&queue, &EventQueue::dispatch_forever)); 00100 00101 // On button press (rise), execute rise_handler in the context of thread 't' 00102 btn.rise(queue.event(rise_handler)); 00103 }
Generated on Sat Jul 16 2022 15:00:59 by
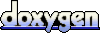