
change official mbed lib and buffer size
Dependencies: Servo WIZnetInterface mbed
Fork of My_Weatherforecast_WIZwiki-W7500 by
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetInterface.h" 00003 #include "Servo.h" 00004 00005 DigitalOut bled(D6); 00006 DigitalOut gled(D5); 00007 DigitalOut rled(D4); 00008 00009 Servo myservo(D15); 00010 00011 int main() { 00012 00013 int phy_link; 00014 printf("Wait a second...\r\n"); 00015 uint8_t mac_addr[6] = {0x00, 0x08, 0xDC, 0x00, 0x01, 0x02}; 00016 00017 EthernetInterface eth; 00018 eth.init(mac_addr); //Use DHCP 00019 00020 while(1){ 00021 00022 eth.connect(); 00023 00024 /* phy link */ 00025 do{ 00026 phy_link = eth.ethernet_link(); 00027 printf("..."); 00028 wait(2); 00029 }while(!phy_link); 00030 printf("\r\n"); 00031 00032 printf("IP Address is %s\r\n", eth.getIPAddress()); 00033 00034 /* TCP socket connect */ 00035 TCPSocketConnection sock; 00036 sock.connect("api.openweathermap.org", 80); 00037 00038 /* weather */ 00039 00040 //char http_cmd[] = "GET /data/2.5/weather?id=2172797 HTTP/1.0\n\n"; 00041 //char http_cmd[] = "GET /data/2.5/weather?q=Seoul,kr HTTP/1.0\n\n"; 00042 char http_cmd[] = "GET /data/2.5/weather?q=London,uk,&appid=2de143494c0b295cca9337e1e96b00e0 HTTP/1.0\r\n\r\n"; 00043 //char http_cmd[] = "GET /data/2.5/weather?q=Berlin,de HTTP/1.0\n\n"; 00044 sock.send_all(http_cmd, sizeof(http_cmd)-1); 00045 00046 /* get info */ 00047 char buffer[1024]; 00048 int ret; 00049 while (true) { 00050 ret = sock.receive(buffer, sizeof(buffer)-1); 00051 if (ret <= 0) 00052 break; 00053 buffer[ret] = '\0'; 00054 printf("Received %d chars from server: %s\n", ret, buffer); 00055 } 00056 00057 /* get weather, city, tempurature */ 00058 char *weather; 00059 char *city; 00060 char *tempure; 00061 00062 char weather_stu[3]; 00063 char city_name[6]; 00064 char tempure_data[6]; 00065 00066 weather = strstr(buffer, "main"); 00067 printf("%.4s\n", weather + 7); 00068 for(int i=0; i<3;i++){ 00069 weather_stu[i] = weather[i+7]; 00070 printf("%c",weather_stu[i]); 00071 } 00072 00073 city = strstr(buffer, "name"); 00074 printf("%.6s\n", city + 7); 00075 for(int k=0; k<6;k++){ 00076 city_name[k] = city[k+7]; 00077 printf("%c",city_name[k]); 00078 } 00079 00080 tempure = strstr(buffer, "temp"); 00081 printf("%.3s\n", tempure + 6); 00082 for(int j=0; j<6;j++){ 00083 tempure_data[j] = tempure[j+6]; 00084 printf("%c",tempure_data[j]); 00085 } 00086 00087 /*tempurature display */ 00088 float data[2]={0}; 00089 data[0] = tempure_data[1]-'7'; 00090 data[1] = tempure_data[2]-'3'; 00091 00092 myservo = (data[0]*10+data[1])/40; 00093 00094 printf("%f",(data[0]*10+data[1])/40); 00095 00096 /* weather display */ 00097 if(strcmp(weather_stu,"Clo")==0) { 00098 rled = 1; 00099 gled = 1; 00100 bled = 1; 00101 }else if(strcmp(weather_stu,"Rai")==0) { 00102 rled = 0; 00103 gled = 0; 00104 bled = 1; 00105 }else if(strcmp(weather_stu,"Cle")==0){ 00106 rled = 1; 00107 gled = 1; 00108 bled = 0; 00109 }else if(strcmp(weather_stu,"Mis")==0){ 00110 rled = 1; 00111 gled = 0; 00112 bled = 1; 00113 }else if(strcmp(weather_stu,"Haz")==0) { 00114 rled = 1; 00115 gled = 1; 00116 bled = 1; 00117 }else if(strcmp(weather_stu,"Fog")==0){ 00118 rled = 1; 00119 gled = 0; 00120 bled = 1; 00121 }else { 00122 rled = 0; 00123 gled = 0; 00124 bled = 0; 00125 } 00126 00127 sock.close(); 00128 00129 eth.disconnect(); 00130 00131 wait(60.0); 00132 }; 00133 00134 }
Generated on Wed Jul 13 2022 11:53:59 by
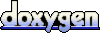