fork allowing use of the latest official mbed networking library
Fork of Agentbed by
Agentbed.h
00001 /* 00002 Agentbed library 00003 Modified for mbed, 2010 Suga. 00004 00005 00006 Agentuino.cpp - An Arduino library for a lightweight SNMP Agent. 00007 Copyright (C) 2010 Eric C. Gionet <lavco_eg@hotmail.com> 00008 All rights reserved. 00009 00010 This library is free software; you can redistribute it and/or 00011 modify it under the terms of the GNU Lesser General Public 00012 License as published by the Free Software Foundation; either 00013 version 2.1 of the License, or (at your option) any later version. 00014 00015 This library is distributed in the hope that it will be useful, 00016 but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU 00018 Lesser General Public License for more details. 00019 00020 You should have received a copy of the GNU Lesser General Public 00021 License along with this library; if not, write to the Free Software 00022 Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA 00023 */ 00024 00025 #ifndef Agentbed_h 00026 #define Agentbed_h 00027 00028 #define SNMP_DEFAULT_PORT 161 00029 #define SNMP_MIN_OID_LEN 2 00030 #define SNMP_MAX_OID_LEN 64 // 128 00031 #define SNMP_MAX_NAME_LEN 20 00032 #define SNMP_MAX_VALUE_LEN 64 // 128 ??? should limit this 00033 #define SNMP_MAX_PACKET_LEN SNMP_MAX_VALUE_LEN + SNMP_MAX_OID_LEN + 25 //??? 00034 #define SNMP_FREE(s) do { if (s) { free((void *)s); s=NULL; } } while(0) 00035 //Frees a pointer only if it is !NULL and sets its value to NULL. 00036 00037 #include "mbed.h" 00038 #include "UDPSocket.h" 00039 #include <stdlib.h> 00040 00041 extern "C" { 00042 // callback function 00043 typedef void (*onPduReceiveCallback)(void); 00044 } 00045 00046 //typedef long long int64_t; 00047 typedef unsigned long long uint64_t; 00048 //typedef long int32_t; 00049 //typedef unsigned long uint32_t; 00050 //typedef unsigned char uint8_t; 00051 //typedef short int16_t; 00052 //typedef unsigned short uint16_t; 00053 typedef unsigned char byte; 00054 00055 typedef union uint64_u { 00056 uint64_t uint64; 00057 byte data[8]; 00058 } uint64_u; 00059 00060 typedef union int32_u { 00061 int32_t int32; 00062 byte data[4]; 00063 } int32_u; 00064 00065 typedef union uint32_u{ 00066 uint32_t uint32; 00067 byte data[4]; 00068 } uint32_u; 00069 00070 typedef union int16_u { 00071 int16_t int16; 00072 byte data[2]; 00073 } int16_u; 00074 00075 //typedef union uint16_u { 00076 // uint16_t uint16; 00077 // byte data[2]; 00078 //}; 00079 00080 typedef union float_u{ 00081 float float_t; 00082 byte data[4]; 00083 } float_u; 00084 00085 enum ASN_BER_BASE_TYPES { 00086 // ASN/BER base types 00087 ASN_BER_BASE_UNIVERSAL = 0x0, 00088 ASN_BER_BASE_APPLICATION = 0x40, 00089 ASN_BER_BASE_CONTEXT = 0x80, 00090 ASN_BER_BASE_PUBLIC = 0xC0, 00091 ASN_BER_BASE_PRIMITIVE = 0x0, 00092 ASN_BER_BASE_CONSTRUCTOR = 0x20 00093 }; 00094 00095 enum SNMP_PDU_TYPES { 00096 // PDU choices 00097 SNMP_PDU_GET = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 0, 00098 SNMP_PDU_GET_NEXT = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 1, 00099 SNMP_PDU_RESPONSE = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 2, 00100 SNMP_PDU_SET = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 3, 00101 SNMP_PDU_TRAP = ASN_BER_BASE_CONTEXT | ASN_BER_BASE_CONSTRUCTOR | 4 00102 }; 00103 00104 enum SNMP_TRAP_TYPES { 00105 // Trap generic types: 00106 SNMP_TRAP_COLD_START = 0, 00107 SNMP_TRAP_WARM_START = 1, 00108 SNMP_TRAP_LINK_DOWN = 2, 00109 SNMP_TRAP_LINK_UP = 3, 00110 SNMP_TRAP_AUTHENTICATION_FAIL = 4, 00111 SNMP_TRAP_EGP_NEIGHBORLOSS = 5, 00112 SNMP_TRAP_ENTERPRISE_SPECIFIC = 6 00113 }; 00114 00115 enum SNMP_ERR_CODES { 00116 SNMP_ERR_NO_ERROR = 0, 00117 SNMP_ERR_TOO_BIG = 1, 00118 SNMP_ERR_NO_SUCH_NAME = 2, 00119 SNMP_ERR_BAD_VALUE = 3, 00120 SNMP_ERR_READ_ONLY = 4, 00121 SNMP_ERR_GEN_ERROR = 5, 00122 00123 SNMP_ERR_NO_ACCESS = 6, 00124 SNMP_ERR_WRONG_TYPE = 7, 00125 SNMP_ERR_WRONG_LENGTH = 8, 00126 SNMP_ERR_WRONG_ENCODING = 9, 00127 SNMP_ERR_WRONG_VALUE = 10, 00128 SNMP_ERR_NO_CREATION = 11, 00129 SNMP_ERR_INCONSISTANT_VALUE = 12, 00130 SNMP_ERR_RESOURCE_UNAVAILABLE = 13, 00131 SNMP_ERR_COMMIT_FAILED = 14, 00132 SNMP_ERR_UNDO_FAILED = 15, 00133 SNMP_ERR_AUTHORIZATION_ERROR = 16, 00134 SNMP_ERR_NOT_WRITABLE = 17, 00135 SNMP_ERR_INCONSISTEN_NAME = 18 00136 }; 00137 00138 enum SNMP_API_STAT_CODES { 00139 SNMP_API_STAT_SUCCESS = 0, 00140 SNMP_API_STAT_MALLOC_ERR = 1, 00141 SNMP_API_STAT_NAME_TOO_BIG = 2, 00142 SNMP_API_STAT_OID_TOO_BIG = 3, 00143 SNMP_API_STAT_VALUE_TOO_BIG = 4, 00144 SNMP_API_STAT_PACKET_INVALID = 5, 00145 SNMP_API_STAT_PACKET_TOO_BIG = 6 00146 }; 00147 00148 // 00149 // http://oreilly.com/catalog/esnmp/chapter/ch02.html Table 2-1: SMIv1 Datatypes 00150 00151 enum SNMP_SYNTAXES { 00152 // SNMP ObjectSyntax values 00153 SNMP_SYNTAX_SEQUENCE = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_CONSTRUCTOR | 0x10, 00154 // These values are used in the "syntax" member of VALUEs 00155 SNMP_SYNTAX_BOOL = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 1, 00156 SNMP_SYNTAX_INT = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 2, 00157 SNMP_SYNTAX_BITS = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 3, 00158 SNMP_SYNTAX_OCTETS = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 4, 00159 SNMP_SYNTAX_NULL = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 5, 00160 SNMP_SYNTAX_OID = ASN_BER_BASE_UNIVERSAL | ASN_BER_BASE_PRIMITIVE | 6, 00161 SNMP_SYNTAX_INT32 = SNMP_SYNTAX_INT, 00162 SNMP_SYNTAX_IP_ADDRESS = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 0, 00163 SNMP_SYNTAX_COUNTER = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 1, 00164 SNMP_SYNTAX_GAUGE = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 2, 00165 SNMP_SYNTAX_TIME_TICKS = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 3, 00166 SNMP_SYNTAX_OPAQUE = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 4, 00167 SNMP_SYNTAX_NSAPADDR = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 5, 00168 SNMP_SYNTAX_COUNTER64 = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 6, 00169 SNMP_SYNTAX_UINT32 = ASN_BER_BASE_APPLICATION | ASN_BER_BASE_PRIMITIVE | 7, 00170 SNMP_SYNTAX_OPAQUE_FLOAT = 0x78, 00171 }; 00172 00173 typedef struct SNMP_OID { 00174 byte data[SNMP_MAX_OID_LEN]; // ushort array insted?? 00175 size_t size; 00176 // 00177 void fromString(const char *buffer) { 00178 } 00179 // 00180 void toString(char *buffer) { 00181 buffer[0] = '1'; 00182 buffer[1] = '.'; 00183 buffer[2] = '3'; 00184 buffer[3] = '\0'; 00185 // 00186 // tmp buffer - short (Int16) 00187 //char *buff = (char *)malloc(sizeof(char)*16); // I ya ya keeps hanging the MCU 00188 char buff[16]; 00189 byte hsize = size - 1; 00190 byte hpos = 1; 00191 uint16_t subid; 00192 // 00193 while ( hsize > 0 ) { 00194 subid = 0; 00195 uint16_t b = 0; 00196 do { 00197 uint16_t next = data[hpos++]; 00198 b = next & 0xFF; 00199 subid = (subid << 7) + (b & ~0x80); 00200 hsize--; 00201 } while ( (hsize > 0) && ((b & 0x80) != 0) ); 00202 //utoa(subid, buff, 10); 00203 sprintf(buff, "%d", subid); 00204 strcat(buffer, "."); 00205 strcat(buffer, buff); 00206 } 00207 // 00208 // free buff 00209 //SNMP_FREE(buff); 00210 }; 00211 } SNMP_OID; 00212 00213 // union for values? 00214 // 00215 typedef struct SNMP_VALUE { 00216 byte data[SNMP_MAX_VALUE_LEN]; 00217 size_t size; 00218 SNMP_SYNTAXES syntax; 00219 // 00220 byte i; // for encoding/decoding functions 00221 // 00222 // clear's buffer and sets size to 0 00223 void clear(void) { 00224 memset(data, 0, SNMP_MAX_VALUE_LEN); 00225 size = 0; 00226 } 00227 // 00228 // 00229 // ASN.1 decoding functions 00230 // 00231 // decode's an octet string, object-identifier, opaque syntax to string 00232 SNMP_ERR_CODES decode(char *value, size_t max_size) { 00233 if ( syntax == SNMP_SYNTAX_OCTETS || syntax == SNMP_SYNTAX_OID 00234 || syntax == SNMP_SYNTAX_OPAQUE ) { 00235 if ( strlen(value) - 1 < max_size ) { 00236 if ( syntax == SNMP_SYNTAX_OID ) { 00237 value[0] = '1'; 00238 value[1] = '.'; 00239 value[2] = '3'; 00240 value[3] = '\0'; 00241 // 00242 // tmp buffer - ushort (UInt16) 00243 //char *buff = (char *)malloc(sizeof(char)*16); // I ya ya..keep hanging the MCU 00244 char buff[16]; 00245 byte hsize = size - 1; 00246 byte hpos = 1; 00247 uint16_t subid; 00248 // 00249 while ( hsize > 0 ) { 00250 subid = 0; 00251 uint16_t b = 0; 00252 do { 00253 uint16_t next = data[hpos++]; 00254 b = next & 0xFF; 00255 subid = (subid << 7) + (b & ~0x80); 00256 hsize--; 00257 } while ( (hsize > 0) && ((b & 0x80) != 0) ); 00258 //utoa(subid, buff, 10); 00259 sprintf(buff, "%d", subid); 00260 strcat(value, "."); 00261 strcat(value, buff); 00262 } 00263 // 00264 // free buff 00265 //SNMP_FREE(buff); 00266 } else { 00267 for ( i = 0; i < size; i++ ) { 00268 value[i] = (char)data[i]; 00269 } 00270 value[size] = '\0'; 00271 } 00272 return SNMP_ERR_NO_ERROR; 00273 } else { 00274 clear(); 00275 return SNMP_ERR_TOO_BIG; 00276 } 00277 } else { 00278 clear(); 00279 return SNMP_ERR_WRONG_TYPE; 00280 } 00281 } 00282 // 00283 // decode's an int syntax to int16 ?? is this applicable 00284 SNMP_ERR_CODES decode(int16_t *value) { 00285 if ( syntax == SNMP_SYNTAX_INT ) { 00286 int16_u tmp; 00287 tmp.data[1] = data[0]; 00288 tmp.data[0] = data[1]; 00289 *value = tmp.int16; 00290 return SNMP_ERR_NO_ERROR; 00291 } else { 00292 clear(); 00293 return SNMP_ERR_WRONG_TYPE; 00294 } 00295 } 00296 // 00297 // decode's an int32 syntax to int32 00298 SNMP_ERR_CODES decode(int32_t *value) { 00299 if ( syntax == SNMP_SYNTAX_INT32 ) { 00300 int32_u tmp; 00301 tmp.data[3] = data[0]; 00302 tmp.data[2] = data[1]; 00303 tmp.data[1] = data[2]; 00304 tmp.data[0] = data[3]; 00305 *value = tmp.int32; 00306 return SNMP_ERR_NO_ERROR; 00307 } else { 00308 clear(); 00309 return SNMP_ERR_WRONG_TYPE; 00310 } 00311 } 00312 // 00313 // decode's an uint32, counter, time-ticks, gauge syntax to uint32 00314 SNMP_ERR_CODES decode(uint32_t *value) { 00315 if ( syntax == SNMP_SYNTAX_COUNTER || syntax == SNMP_SYNTAX_TIME_TICKS 00316 || syntax == SNMP_SYNTAX_GAUGE || syntax == SNMP_SYNTAX_UINT32 ) { 00317 uint32_u tmp; 00318 tmp.data[3] = data[0]; 00319 tmp.data[2] = data[1]; 00320 tmp.data[1] = data[2]; 00321 tmp.data[0] = data[3]; 00322 *value = tmp.uint32; 00323 return SNMP_ERR_NO_ERROR; 00324 } else { 00325 clear(); 00326 return SNMP_ERR_WRONG_TYPE; 00327 } 00328 } 00329 // 00330 // decode's an ip-address, NSAP-address syntax to an ip-address byte array 00331 SNMP_ERR_CODES decode(byte *value) { 00332 memset(data, 0, SNMP_MAX_VALUE_LEN); 00333 if ( syntax == SNMP_SYNTAX_IP_ADDRESS || syntax == SNMP_SYNTAX_NSAPADDR ) { 00334 if ( sizeof(value) > 4 ) { 00335 clear(); 00336 return SNMP_ERR_TOO_BIG; 00337 } else { 00338 size = 4; 00339 data[0] = value[3]; 00340 data[1] = value[2]; 00341 data[2] = value[1]; 00342 data[3] = value[0]; 00343 return SNMP_ERR_NO_ERROR; 00344 } 00345 } else { 00346 clear(); 00347 return SNMP_ERR_WRONG_TYPE; 00348 } 00349 } 00350 // 00351 // decode's a boolean syntax to boolean 00352 SNMP_ERR_CODES decode(bool *value) { 00353 if ( syntax == SNMP_SYNTAX_BOOL ) { 00354 *value = (data[0] != 0); 00355 return SNMP_ERR_NO_ERROR; 00356 } else { 00357 clear(); 00358 return SNMP_ERR_WRONG_TYPE; 00359 } 00360 } 00361 // 00362 // 00363 // ASN.1 encoding functions 00364 // 00365 // encode's a octet string to a string, opaque syntax 00366 // encode object-identifier here?? 00367 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const char *value) { 00368 memset(data, 0, SNMP_MAX_VALUE_LEN); 00369 if ( syn == SNMP_SYNTAX_OCTETS || syn == SNMP_SYNTAX_OPAQUE ) { 00370 if ( strlen(value) - 1 < SNMP_MAX_VALUE_LEN ) { 00371 syntax = syn; 00372 size = strlen(value); 00373 for ( i = 0; i < size; i++ ) { 00374 data[i] = (byte)value[i]; 00375 } 00376 return SNMP_ERR_NO_ERROR; 00377 } else { 00378 clear(); 00379 return SNMP_ERR_TOO_BIG; 00380 } 00381 } else { 00382 clear(); 00383 return SNMP_ERR_WRONG_TYPE; 00384 } 00385 } 00386 // 00387 // encode's an int16 to int, opaque syntax 00388 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const int16_t value) { 00389 memset(data, 0, SNMP_MAX_VALUE_LEN); 00390 if ( syn == SNMP_SYNTAX_INT || syn == SNMP_SYNTAX_OPAQUE ) { 00391 int16_u tmp; 00392 size = 2; 00393 syntax = syn; 00394 tmp.int16 = value; 00395 data[0] = tmp.data[1]; 00396 data[1] = tmp.data[0]; 00397 return SNMP_ERR_NO_ERROR; 00398 } else { 00399 clear(); 00400 return SNMP_ERR_WRONG_TYPE; 00401 } 00402 } 00403 // 00404 // encode's an int32 to int32, opaque syntax 00405 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const int32_t value) { 00406 memset(data, 0, SNMP_MAX_VALUE_LEN); 00407 if ( syn == SNMP_SYNTAX_INT32 || syn == SNMP_SYNTAX_OPAQUE ) { 00408 int32_u tmp; 00409 size = 4; 00410 syntax = syn; 00411 tmp.int32 = value; 00412 data[0] = tmp.data[3]; 00413 data[1] = tmp.data[2]; 00414 data[2] = tmp.data[1]; 00415 data[3] = tmp.data[0]; 00416 return SNMP_ERR_NO_ERROR; 00417 } else { 00418 clear(); 00419 return SNMP_ERR_WRONG_TYPE; 00420 } 00421 } 00422 // 00423 // encode's an uint32 to uint32, counter, time-ticks, gauge, opaque syntax 00424 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const uint32_t value) { 00425 memset(data, 0, SNMP_MAX_VALUE_LEN); 00426 if ( syn == SNMP_SYNTAX_COUNTER || syn == SNMP_SYNTAX_TIME_TICKS 00427 || syn == SNMP_SYNTAX_GAUGE || syn == SNMP_SYNTAX_UINT32 00428 || syn == SNMP_SYNTAX_OPAQUE ) { 00429 uint32_u tmp; 00430 size = 4; 00431 syntax = syn; 00432 tmp.uint32 = value; 00433 data[0] = tmp.data[3]; 00434 data[1] = tmp.data[2]; 00435 data[2] = tmp.data[1]; 00436 data[3] = tmp.data[0]; 00437 return SNMP_ERR_NO_ERROR; 00438 } else { 00439 clear(); 00440 return SNMP_ERR_WRONG_TYPE; 00441 } 00442 } 00443 // 00444 // encode's an ip-address byte array to ip-address, NSAP-address, opaque syntax 00445 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const byte *value) { 00446 memset(data, 0, SNMP_MAX_VALUE_LEN); 00447 if ( syn == SNMP_SYNTAX_IP_ADDRESS || syn == SNMP_SYNTAX_NSAPADDR 00448 || syn == SNMP_SYNTAX_OPAQUE ) { 00449 if ( sizeof(value) > 4 ) { 00450 clear(); 00451 return SNMP_ERR_TOO_BIG; 00452 } else { 00453 size = 4; 00454 syntax = syn; 00455 data[0] = value[3]; 00456 data[1] = value[2]; 00457 data[2] = value[1]; 00458 data[3] = value[0]; 00459 return SNMP_ERR_NO_ERROR; 00460 } 00461 } else { 00462 clear(); 00463 return SNMP_ERR_WRONG_TYPE; 00464 } 00465 } 00466 // 00467 // encode's a boolean to boolean, opaque syntax 00468 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const bool value) { 00469 memset(data, 0, SNMP_MAX_VALUE_LEN); 00470 if ( syn == SNMP_SYNTAX_BOOL || syn == SNMP_SYNTAX_OPAQUE ) { 00471 size = 1; 00472 syntax = syn; 00473 data[0] = value ? 0xff : 0; 00474 return SNMP_ERR_NO_ERROR; 00475 } else { 00476 clear(); 00477 return SNMP_ERR_WRONG_TYPE; 00478 } 00479 } 00480 // 00481 // encode's an uint64 to counter64, opaque syntax 00482 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const uint64_t value) { 00483 memset(data, 0, SNMP_MAX_VALUE_LEN); 00484 if ( syn == SNMP_SYNTAX_COUNTER64 || syn == SNMP_SYNTAX_OPAQUE ) { 00485 uint64_u tmp; 00486 size = 8; 00487 syntax = syn; 00488 tmp.uint64 = value; 00489 data[0] = tmp.data[7]; 00490 data[1] = tmp.data[6]; 00491 data[2] = tmp.data[5]; 00492 data[3] = tmp.data[4]; 00493 data[4] = tmp.data[3]; 00494 data[5] = tmp.data[2]; 00495 data[6] = tmp.data[1]; 00496 data[7] = tmp.data[0]; 00497 return SNMP_ERR_NO_ERROR; 00498 } else { 00499 clear(); 00500 return SNMP_ERR_WRONG_TYPE; 00501 } 00502 } 00503 // 00504 // encode's an int32 to int32, opaque syntax 00505 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn, const float value) { 00506 memset(data, 0, SNMP_MAX_VALUE_LEN); 00507 if ( syn == SNMP_SYNTAX_OPAQUE_FLOAT ) { 00508 float_u tmp; 00509 size = 4; 00510 syntax = syn; 00511 tmp.float_t = value; 00512 data[0] = tmp.data[3]; 00513 data[1] = tmp.data[2]; 00514 data[2] = tmp.data[1]; 00515 data[3] = tmp.data[0]; 00516 return SNMP_ERR_NO_ERROR; 00517 } else { 00518 clear(); 00519 return SNMP_ERR_WRONG_TYPE; 00520 } 00521 } 00522 // 00523 // encode's a null to null, opaque syntax 00524 SNMP_ERR_CODES encode(SNMP_SYNTAXES syn) { 00525 clear(); 00526 if ( syn == SNMP_SYNTAX_NULL || syn == SNMP_SYNTAX_OPAQUE ) { 00527 size = 0; 00528 syntax = syn; 00529 return SNMP_ERR_NO_ERROR; 00530 } else { 00531 return SNMP_ERR_WRONG_TYPE; 00532 } 00533 } 00534 } SNMP_VALUE; 00535 00536 typedef struct SNMP_PDU { 00537 SNMP_PDU_TYPES type; 00538 int32_t version; 00539 int32_t requestId; 00540 SNMP_ERR_CODES error; 00541 int32_t errorIndex; 00542 SNMP_OID OID; 00543 SNMP_VALUE VALUE; 00544 } SNMP_PDU; 00545 00546 class SNMP { 00547 public: 00548 // Agent functions 00549 SNMP_API_STAT_CODES init(); 00550 SNMP_API_STAT_CODES init(char *getCommName, char *setCommName); 00551 SNMP_API_STAT_CODES toPdu( SNMP_PDU *pdu,char *_packet,uint16_t _packetSize); 00552 uint16_t fromPdu(SNMP_PDU *pdu,char *_packet); 00553 void freePdu(SNMP_PDU *pdu); 00554 00555 // Helper functions 00556 00557 private: 00558 uint16_t _packetPos; 00559 SNMP_PDU_TYPES _dstType; 00560 char *_getCommName; 00561 size_t _getSize; 00562 char *_setCommName; 00563 size_t _setSize; 00564 }; 00565 00566 //extern SNMP Agentbed; 00567 00568 #endif
Generated on Wed Jul 13 2022 06:43:37 by
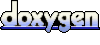