
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
traits.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Copyright (C) 2013, OpenCV Foundation, all rights reserved. 00016 // Third party copyrights are property of their respective owners. 00017 // 00018 // Redistribution and use in source and binary forms, with or without modification, 00019 // are permitted provided that the following conditions are met: 00020 // 00021 // * Redistribution's of source code must retain the above copyright notice, 00022 // this list of conditions and the following disclaimer. 00023 // 00024 // * Redistribution's in binary form must reproduce the above copyright notice, 00025 // this list of conditions and the following disclaimer in the documentation 00026 // and/or other materials provided with the distribution. 00027 // 00028 // * The name of the copyright holders may not be used to endorse or promote products 00029 // derived from this software without specific prior written permission. 00030 // 00031 // This software is provided by the copyright holders and contributors "as is" and 00032 // any express or implied warranties, including, but not limited to, the implied 00033 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00034 // In no event shall the Intel Corporation or contributors be liable for any direct, 00035 // indirect, incidental, special, exemplary, or consequential damages 00036 // (including, but not limited to, procurement of substitute goods or services; 00037 // loss of use, data, or profits; or business interruption) however caused 00038 // and on any theory of liability, whether in contract, strict liability, 00039 // or tort (including negligence or otherwise) arising in any way out of 00040 // the use of this software, even if advised of the possibility of such damage. 00041 // 00042 //M*/ 00043 00044 #ifndef __OPENCV_CORE_TRAITS_HPP__ 00045 #define __OPENCV_CORE_TRAITS_HPP__ 00046 00047 #include "opencv2/core/cvdef.h" 00048 00049 namespace cv 00050 { 00051 00052 //! @addtogroup core_basic 00053 //! @{ 00054 00055 /** @brief Template "trait" class for OpenCV primitive data types. 00056 00057 A primitive OpenCV data type is one of unsigned char, bool, signed char, unsigned short, signed 00058 short, int, float, double, or a tuple of values of one of these types, where all the values in the 00059 tuple have the same type. Any primitive type from the list can be defined by an identifier in the 00060 form CV_<bit-depth>{U|S|F}C(<number_of_channels>), for example: uchar \~ CV_8UC1, 3-element 00061 floating-point tuple \~ CV_32FC3, and so on. A universal OpenCV structure that is able to store a 00062 single instance of such a primitive data type is Vec. Multiple instances of such a type can be 00063 stored in a std::vector, Mat, Mat_, SparseMat, SparseMat_, or any other container that is able to 00064 store Vec instances. 00065 00066 The DataType class is basically used to provide a description of such primitive data types without 00067 adding any fields or methods to the corresponding classes (and it is actually impossible to add 00068 anything to primitive C/C++ data types). This technique is known in C++ as class traits. It is not 00069 DataType itself that is used but its specialized versions, such as: 00070 @code 00071 template<> class DataType<uchar> 00072 { 00073 typedef uchar value_type; 00074 typedef int work_type; 00075 typedef uchar channel_type; 00076 enum { channel_type = CV_8U, channels = 1, fmt='u', type = CV_8U }; 00077 }; 00078 ... 00079 template<typename _Tp> DataType<std::complex<_Tp> > 00080 { 00081 typedef std::complex<_Tp> value_type; 00082 typedef std::complex<_Tp> work_type; 00083 typedef _Tp channel_type; 00084 // DataDepth is another helper trait class 00085 enum { depth = DataDepth<_Tp>::value, channels=2, 00086 fmt=(channels-1)*256+DataDepth<_Tp>::fmt, 00087 type=CV_MAKETYPE(depth, channels) }; 00088 }; 00089 ... 00090 @endcode 00091 The main purpose of this class is to convert compilation-time type information to an 00092 OpenCV-compatible data type identifier, for example: 00093 @code 00094 // allocates a 30x40 floating-point matrix 00095 Mat A(30, 40, DataType<float>::type); 00096 00097 Mat B = Mat_<std::complex<double> >(3, 3); 00098 // the statement below will print 6, 2 , that is depth == CV_64F, channels == 2 00099 cout << B.depth() << ", " << B.channels() << endl; 00100 @endcode 00101 So, such traits are used to tell OpenCV which data type you are working with, even if such a type is 00102 not native to OpenCV. For example, the matrix B initialization above is compiled because OpenCV 00103 defines the proper specialized template class DataType<complex<_Tp> > . This mechanism is also 00104 useful (and used in OpenCV this way) for generic algorithms implementations. 00105 */ 00106 template<typename _Tp> class DataType 00107 { 00108 public: 00109 typedef _Tp value_type; 00110 typedef value_type work_type; 00111 typedef value_type channel_type; 00112 typedef value_type vec_type; 00113 enum { generic_type = 1, 00114 depth = -1, 00115 channels = 1, 00116 fmt = 0, 00117 type = CV_MAKETYPE(depth, channels) 00118 }; 00119 }; 00120 00121 template<> class DataType<bool> 00122 { 00123 public: 00124 typedef bool value_type; 00125 typedef int work_type; 00126 typedef value_type channel_type; 00127 typedef value_type vec_type; 00128 enum { generic_type = 0, 00129 depth = CV_8U, 00130 channels = 1, 00131 fmt = (int)'u', 00132 type = CV_MAKETYPE(depth, channels) 00133 }; 00134 }; 00135 00136 template<> class DataType<uchar> 00137 { 00138 public: 00139 typedef uchar value_type; 00140 typedef int work_type; 00141 typedef value_type channel_type; 00142 typedef value_type vec_type; 00143 enum { generic_type = 0, 00144 depth = CV_8U, 00145 channels = 1, 00146 fmt = (int)'u', 00147 type = CV_MAKETYPE(depth, channels) 00148 }; 00149 }; 00150 00151 template<> class DataType<schar> 00152 { 00153 public: 00154 typedef schar value_type; 00155 typedef int work_type; 00156 typedef value_type channel_type; 00157 typedef value_type vec_type; 00158 enum { generic_type = 0, 00159 depth = CV_8S, 00160 channels = 1, 00161 fmt = (int)'c', 00162 type = CV_MAKETYPE(depth, channels) 00163 }; 00164 }; 00165 00166 template<> class DataType<char> 00167 { 00168 public: 00169 typedef schar value_type; 00170 typedef int work_type; 00171 typedef value_type channel_type; 00172 typedef value_type vec_type; 00173 enum { generic_type = 0, 00174 depth = CV_8S, 00175 channels = 1, 00176 fmt = (int)'c', 00177 type = CV_MAKETYPE(depth, channels) 00178 }; 00179 }; 00180 00181 template<> class DataType<ushort> 00182 { 00183 public: 00184 typedef ushort value_type; 00185 typedef int work_type; 00186 typedef value_type channel_type; 00187 typedef value_type vec_type; 00188 enum { generic_type = 0, 00189 depth = CV_16U, 00190 channels = 1, 00191 fmt = (int)'w', 00192 type = CV_MAKETYPE(depth, channels) 00193 }; 00194 }; 00195 00196 template<> class DataType<short> 00197 { 00198 public: 00199 typedef short value_type; 00200 typedef int work_type; 00201 typedef value_type channel_type; 00202 typedef value_type vec_type; 00203 enum { generic_type = 0, 00204 depth = CV_16S, 00205 channels = 1, 00206 fmt = (int)'s', 00207 type = CV_MAKETYPE(depth, channels) 00208 }; 00209 }; 00210 00211 template<> class DataType<int> 00212 { 00213 public: 00214 typedef int value_type; 00215 typedef value_type work_type; 00216 typedef value_type channel_type; 00217 typedef value_type vec_type; 00218 enum { generic_type = 0, 00219 depth = CV_32S, 00220 channels = 1, 00221 fmt = (int)'i', 00222 type = CV_MAKETYPE(depth, channels) 00223 }; 00224 }; 00225 00226 template<> class DataType<float> 00227 { 00228 public: 00229 typedef float value_type; 00230 typedef value_type work_type; 00231 typedef value_type channel_type; 00232 typedef value_type vec_type; 00233 enum { generic_type = 0, 00234 depth = CV_32F, 00235 channels = 1, 00236 fmt = (int)'f', 00237 type = CV_MAKETYPE(depth, channels) 00238 }; 00239 }; 00240 00241 template<> class DataType<double> 00242 { 00243 public: 00244 typedef double value_type; 00245 typedef value_type work_type; 00246 typedef value_type channel_type; 00247 typedef value_type vec_type; 00248 enum { generic_type = 0, 00249 depth = CV_64F, 00250 channels = 1, 00251 fmt = (int)'d', 00252 type = CV_MAKETYPE(depth, channels) 00253 }; 00254 }; 00255 00256 00257 /** @brief A helper class for cv::DataType 00258 00259 The class is specialized for each fundamental numerical data type supported by OpenCV. It provides 00260 DataDepth<T>::value constant. 00261 */ 00262 template<typename _Tp> class DataDepth 00263 { 00264 public: 00265 enum 00266 { 00267 value = DataType<_Tp>::depth, 00268 fmt = DataType<_Tp>::fmt 00269 }; 00270 }; 00271 00272 00273 00274 template<int _depth> class TypeDepth 00275 { 00276 enum { depth = CV_USRTYPE1 }; 00277 typedef void value_type; 00278 }; 00279 00280 template<> class TypeDepth<CV_8U> 00281 { 00282 enum { depth = CV_8U }; 00283 typedef uchar value_type; 00284 }; 00285 00286 template<> class TypeDepth<CV_8S> 00287 { 00288 enum { depth = CV_8S }; 00289 typedef schar value_type; 00290 }; 00291 00292 template<> class TypeDepth<CV_16U> 00293 { 00294 enum { depth = CV_16U }; 00295 typedef ushort value_type; 00296 }; 00297 00298 template<> class TypeDepth<CV_16S> 00299 { 00300 enum { depth = CV_16S }; 00301 typedef short value_type; 00302 }; 00303 00304 template<> class TypeDepth<CV_32S> 00305 { 00306 enum { depth = CV_32S }; 00307 typedef int value_type; 00308 }; 00309 00310 template<> class TypeDepth<CV_32F> 00311 { 00312 enum { depth = CV_32F }; 00313 typedef float value_type; 00314 }; 00315 00316 template<> class TypeDepth<CV_64F> 00317 { 00318 enum { depth = CV_64F }; 00319 typedef double value_type; 00320 }; 00321 00322 //! @} 00323 00324 } // cv 00325 00326 #endif // __OPENCV_CORE_TRAITS_HPP__ 00327
Generated on Tue Jul 12 2022 16:42:40 by
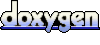