
opencv on mbed
Embed:
(wiki syntax)
Show/hide line numbers
eigen.hpp
00001 /*M/////////////////////////////////////////////////////////////////////////////////////// 00002 // 00003 // IMPORTANT: READ BEFORE DOWNLOADING, COPYING, INSTALLING OR USING. 00004 // 00005 // By downloading, copying, installing or using the software you agree to this license. 00006 // If you do not agree to this license, do not download, install, 00007 // copy or use the software. 00008 // 00009 // 00010 // License Agreement 00011 // For Open Source Computer Vision Library 00012 // 00013 // Copyright (C) 2000-2008, Intel Corporation, all rights reserved. 00014 // Copyright (C) 2009, Willow Garage Inc., all rights reserved. 00015 // Copyright (C) 2013, OpenCV Foundation, all rights reserved. 00016 // Third party copyrights are property of their respective owners. 00017 // 00018 // Redistribution and use in source and binary forms, with or without modification, 00019 // are permitted provided that the following conditions are met: 00020 // 00021 // * Redistribution's of source code must retain the above copyright notice, 00022 // this list of conditions and the following disclaimer. 00023 // 00024 // * Redistribution's in binary form must reproduce the above copyright notice, 00025 // this list of conditions and the following disclaimer in the documentation 00026 // and/or other materials provided with the distribution. 00027 // 00028 // * The name of the copyright holders may not be used to endorse or promote products 00029 // derived from this software without specific prior written permission. 00030 // 00031 // This software is provided by the copyright holders and contributors "as is" and 00032 // any express or implied warranties, including, but not limited to, the implied 00033 // warranties of merchantability and fitness for a particular purpose are disclaimed. 00034 // In no event shall the Intel Corporation or contributors be liable for any direct, 00035 // indirect, incidental, special, exemplary, or consequential damages 00036 // (including, but not limited to, procurement of substitute goods or services; 00037 // loss of use, data, or profits; or business interruption) however caused 00038 // and on any theory of liability, whether in contract, strict liability, 00039 // or tort (including negligence or otherwise) arising in any way out of 00040 // the use of this software, even if advised of the possibility of such damage. 00041 // 00042 //M*/ 00043 00044 00045 #ifndef __OPENCV_CORE_EIGEN_HPP__ 00046 #define __OPENCV_CORE_EIGEN_HPP__ 00047 00048 #include "opencv2/core.hpp" 00049 00050 #if defined _MSC_VER && _MSC_VER >= 1200 00051 #pragma warning( disable: 4714 ) //__forceinline is not inlined 00052 #pragma warning( disable: 4127 ) //conditional expression is constant 00053 #pragma warning( disable: 4244 ) //conversion from '__int64' to 'int', possible loss of data 00054 #endif 00055 00056 namespace cv 00057 { 00058 00059 //! @addtogroup core_eigen 00060 //! @{ 00061 00062 template<typename _Tp, int _rows, int _cols, int _options, int _maxRows, int _maxCols> static inline 00063 void eigen2cv( const Eigen::Matrix<_Tp, _rows, _cols, _options, _maxRows, _maxCols>& src, Mat& dst ) 00064 { 00065 if( !(src.Flags & Eigen::RowMajorBit) ) 00066 { 00067 Mat _src(src.cols(), src.rows(), DataType<_Tp>::type, 00068 (void*)src.data(), src.stride()*sizeof(_Tp)); 00069 transpose(_src, dst); 00070 } 00071 else 00072 { 00073 Mat _src(src.rows(), src.cols(), DataType<_Tp>::type, 00074 (void*)src.data(), src.stride()*sizeof(_Tp)); 00075 _src.copyTo(dst); 00076 } 00077 } 00078 00079 // Matx case 00080 template<typename _Tp, int _rows, int _cols, int _options, int _maxRows, int _maxCols> static inline 00081 void eigen2cv( const Eigen::Matrix<_Tp, _rows, _cols, _options, _maxRows, _maxCols>& src, 00082 Matx<_Tp, _rows, _cols>& dst ) 00083 { 00084 if( !(src.Flags & Eigen::RowMajorBit) ) 00085 { 00086 dst = Matx<_Tp, _cols, _rows>(static_cast<const _Tp*>(src.data())).t(); 00087 } 00088 else 00089 { 00090 dst = Matx<_Tp, _rows, _cols>(static_cast<const _Tp*>(src.data())); 00091 } 00092 } 00093 00094 template<typename _Tp, int _rows, int _cols, int _options, int _maxRows, int _maxCols> static inline 00095 void cv2eigen( const Mat& src, 00096 Eigen::Matrix<_Tp, _rows, _cols, _options, _maxRows, _maxCols>& dst ) 00097 { 00098 CV_DbgAssert(src.rows == _rows && src.cols == _cols); 00099 if( !(dst.Flags & Eigen::RowMajorBit) ) 00100 { 00101 const Mat _dst(src.cols, src.rows, DataType<_Tp>::type, 00102 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00103 if( src.type() == _dst.type() ) 00104 transpose(src, _dst); 00105 else if( src.cols == src.rows ) 00106 { 00107 src.convertTo(_dst, _dst.type()); 00108 transpose(_dst, _dst); 00109 } 00110 else 00111 Mat(src.t()).convertTo(_dst, _dst.type()); 00112 } 00113 else 00114 { 00115 const Mat _dst(src.rows, src.cols, DataType<_Tp>::type, 00116 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00117 src.convertTo(_dst, _dst.type()); 00118 } 00119 } 00120 00121 // Matx case 00122 template<typename _Tp, int _rows, int _cols, int _options, int _maxRows, int _maxCols> static inline 00123 void cv2eigen( const Matx<_Tp, _rows, _cols>& src, 00124 Eigen::Matrix<_Tp, _rows, _cols, _options, _maxRows, _maxCols>& dst ) 00125 { 00126 if( !(dst.Flags & Eigen::RowMajorBit) ) 00127 { 00128 const Mat _dst(_cols, _rows, DataType<_Tp>::type, 00129 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00130 transpose(src, _dst); 00131 } 00132 else 00133 { 00134 const Mat _dst(_rows, _cols, DataType<_Tp>::type, 00135 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00136 Mat(src).copyTo(_dst); 00137 } 00138 } 00139 00140 template<typename _Tp> static inline 00141 void cv2eigen( const Mat& src, 00142 Eigen::Matrix<_Tp, Eigen::Dynamic, Eigen::Dynamic>& dst ) 00143 { 00144 dst.resize(src.rows, src.cols); 00145 if( !(dst.Flags & Eigen::RowMajorBit) ) 00146 { 00147 const Mat _dst(src.cols, src.rows, DataType<_Tp>::type, 00148 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00149 if( src.type() == _dst.type() ) 00150 transpose(src, _dst); 00151 else if( src.cols == src.rows ) 00152 { 00153 src.convertTo(_dst, _dst.type()); 00154 transpose(_dst, _dst); 00155 } 00156 else 00157 Mat(src.t()).convertTo(_dst, _dst.type()); 00158 } 00159 else 00160 { 00161 const Mat _dst(src.rows, src.cols, DataType<_Tp>::type, 00162 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00163 src.convertTo(_dst, _dst.type()); 00164 } 00165 } 00166 00167 // Matx case 00168 template<typename _Tp, int _rows, int _cols> static inline 00169 void cv2eigen( const Matx<_Tp, _rows, _cols>& src, 00170 Eigen::Matrix<_Tp, Eigen::Dynamic, Eigen::Dynamic>& dst ) 00171 { 00172 dst.resize(_rows, _cols); 00173 if( !(dst.Flags & Eigen::RowMajorBit) ) 00174 { 00175 const Mat _dst(_cols, _rows, DataType<_Tp>::type, 00176 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00177 transpose(src, _dst); 00178 } 00179 else 00180 { 00181 const Mat _dst(_rows, _cols, DataType<_Tp>::type, 00182 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00183 Mat(src).copyTo(_dst); 00184 } 00185 } 00186 00187 template<typename _Tp> static inline 00188 void cv2eigen( const Mat& src, 00189 Eigen::Matrix<_Tp, Eigen::Dynamic, 1>& dst ) 00190 { 00191 CV_Assert(src.cols == 1); 00192 dst.resize(src.rows); 00193 00194 if( !(dst.Flags & Eigen::RowMajorBit) ) 00195 { 00196 const Mat _dst(src.cols, src.rows, DataType<_Tp>::type, 00197 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00198 if( src.type() == _dst.type() ) 00199 transpose(src, _dst); 00200 else 00201 Mat(src.t()).convertTo(_dst, _dst.type()); 00202 } 00203 else 00204 { 00205 const Mat _dst(src.rows, src.cols, DataType<_Tp>::type, 00206 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00207 src.convertTo(_dst, _dst.type()); 00208 } 00209 } 00210 00211 // Matx case 00212 template<typename _Tp, int _rows> static inline 00213 void cv2eigen( const Matx<_Tp, _rows, 1>& src, 00214 Eigen::Matrix<_Tp, Eigen::Dynamic, 1>& dst ) 00215 { 00216 dst.resize(_rows); 00217 00218 if( !(dst.Flags & Eigen::RowMajorBit) ) 00219 { 00220 const Mat _dst(1, _rows, DataType<_Tp>::type, 00221 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00222 transpose(src, _dst); 00223 } 00224 else 00225 { 00226 const Mat _dst(_rows, 1, DataType<_Tp>::type, 00227 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00228 src.copyTo(_dst); 00229 } 00230 } 00231 00232 00233 template<typename _Tp> static inline 00234 void cv2eigen( const Mat& src, 00235 Eigen::Matrix<_Tp, 1, Eigen::Dynamic>& dst ) 00236 { 00237 CV_Assert(src.rows == 1); 00238 dst.resize(src.cols); 00239 if( !(dst.Flags & Eigen::RowMajorBit) ) 00240 { 00241 const Mat _dst(src.cols, src.rows, DataType<_Tp>::type, 00242 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00243 if( src.type() == _dst.type() ) 00244 transpose(src, _dst); 00245 else 00246 Mat(src.t()).convertTo(_dst, _dst.type()); 00247 } 00248 else 00249 { 00250 const Mat _dst(src.rows, src.cols, DataType<_Tp>::type, 00251 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00252 src.convertTo(_dst, _dst.type()); 00253 } 00254 } 00255 00256 //Matx 00257 template<typename _Tp, int _cols> static inline 00258 void cv2eigen( const Matx<_Tp, 1, _cols>& src, 00259 Eigen::Matrix<_Tp, 1, Eigen::Dynamic>& dst ) 00260 { 00261 dst.resize(_cols); 00262 if( !(dst.Flags & Eigen::RowMajorBit) ) 00263 { 00264 const Mat _dst(_cols, 1, DataType<_Tp>::type, 00265 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00266 transpose(src, _dst); 00267 } 00268 else 00269 { 00270 const Mat _dst(1, _cols, DataType<_Tp>::type, 00271 dst.data(), (size_t)(dst.stride()*sizeof(_Tp))); 00272 Mat(src).copyTo(_dst); 00273 } 00274 } 00275 00276 //! @} 00277 00278 } // cv 00279 00280 #endif 00281
Generated on Tue Jul 12 2022 16:42:38 by
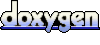