
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "TextLCD.h" 00003 #include "Counter.h" 00004 #include "LIS302.h" 00005 #include "Servo.h" 00006 #include "MSCFileSystem.h" 00007 00008 Servo myservo(p21); 00009 LIS302 acc (p5,p6,p7,p8); 00010 DigitalIn on(p20); 00011 DigitalOut LIS302(LIS302); 00012 DigitalOut led1(LED1); 00013 DigitalOut led2(LED2); 00014 DigitalOut led3(LED3); 00015 DigitalOut led4(LED4); 00016 #define TICK_PERIOD 0.5 00017 MSCFileSystem fs("fs"); 00018 Counter counter(p18); 00019 00020 00021 Ticker tick; 00022 00023 Timer timer; 00024 volatile int tick_active = 0; 00025 00026 void dotick (void) { 00027 tick_active = 1; 00028 00029 } 00030 float samples [2] = {0}; 00031 int index2 = 0; 00032 int start = 1; 00033 00034 char FileName[20]; // this is where we store the file name 00035 00036 int rpm_counter=0; 00037 int main() { 00038 00039 float samples [1] = {0}; 00040 int index = 0; 00041 int flipped_right = 1; 00042 int flipped_left = 1; 00043 00044 00045 // read the current time, and con convert it to a DD/MM/YYYY HH:MM:SS structure 00046 time_t seconds = time(NULL); 00047 struct tm *t = localtime(&seconds); 00048 00049 // use the time to make a new file name 00050 // file name is /fs/ddmmHHMM.csv 00051 // Note we have to add one to the month as it is represented as 0-11 rather than 1-12 :-( 00052 sprintf(FileName,"/fs/%02d%02d%02d%02d.csv",t->tm_mday,1+(t->tm_mon),t->tm_hour,t->tm_min); 00053 FILE *fp = fopen(FileName,"w"); 00054 00055 00056 00057 fprintf(fp, "RPM,X,Y,Z\n");//this part prints the columns headers 00058 00059 fclose(fp); 00060 00061 tick.attach(dotick,0.5); 00062 00063 while (1) { 00064 while (tick_active == 0) {} 00065 rpm_counter = counter.read(); 00066 counter.reset(); 00067 00068 tick_active = 0; 00069 samples[index2] = 120*rpm_counter; 00070 index2++; 00071 if (index2 >= 2) { 00072 index2 = 0; 00073 } 00074 int i; 00075 start = 1; 00076 for (i=0; i<2; i++) { 00077 if (samples[i] <60) { 00078 start = 0; 00079 } 00080 } 00081 if (on) { 00082 led2 = 1; 00083 } 00084 if (!on) { 00085 led2 = 0; 00086 } 00087 if (start) { 00088 led3 = 1; 00089 } 00090 if (!start) { 00091 led3 = 0; // the leds are only to check the program is doing what i want it to! 00092 } 00093 00094 if (start&&on) 00095 FILE *fp = fopen(FileName,"a"); 00096 timer.start (); 00097 while (start&&on) { //if the light gate reads over 60RPM and the swich is on then it will enter this loop 00098 00099 while (tick_active == 0) {} 00100 00101 rpm_counter = counter.read(); 00102 counter.reset(); 00103 00104 00105 fprintf(fp,"%d,%.2f,%.2f,%.2f\n",60*rpm_counter,acc.x(),acc.y(),acc.z()); //the data colected is saved in the file that was opend 00106 led1 = !led1; 00107 tick_active = 0; 00108 00109 00110 samples[index] = acc.y(); 00111 index++; 00112 if (index >= 1) { 00113 index = 0; 00114 } 00115 int i; 00116 00117 flipped_right = 1; 00118 flipped_left =1; 00119 00120 for (i=0; i<1; i++) { 00121 if (samples[i] >= -0.8) { 00122 flipped_left = 0; 00123 } 00124 00125 if (samples[i] <= 0.8) { 00126 flipped_right = 0; //the bit abouve is an array that is looking to see if the accelerometer is reading between 0.8 and -0.8 00127 00128 00129 00130 00131 } 00132 00133 if (flipped_left||flipped_right||!on) { 00134 // if the accelerometer is not reading between 0.8 and -0.8 on the y.acc or the swich is off the following will happen 00135 00136 00137 myservo = 1; // 1. the servo will turn to turn off the engine 00138 00139 timer.stop(); // 2. the timer will stop 00140 00141 fprintf(fp,"this run lasted %f seconds \n", timer.read()); // 3. the timer time will be printed to the file 00142 fprintf(fp,"off,off,off,off"); 00143 fclose(fp); // and last the file will be closed 00144 led1 = 0; 00145 led4 = 1; 00146 } 00147 } 00148 } 00149 } 00150 }
Generated on Wed Jul 13 2022 03:39:42 by
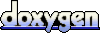