Class header for the AT42QT1010 device.
Dependents: AT42QT1010_Hello_world
AT42QT1010.h
00001 #ifndef AT42QT1010_H 00002 #define AT42QT1010_H 00003 00004 #include "mbed.h" 00005 00006 ///Class for the AT42QT1010 Capactitive Touch Sensor 00007 class AT42QT1010 { 00008 00009 public: 00010 /**Define the device without use of the on-board LED*/ 00011 explicit AT42QT1010(PinName); 00012 /**Define the device using both breakout I/O pins. Requires manual modification to the breakout before use.*/ 00013 explicit AT42QT1010(PinName, PinName); 00014 ~AT42QT1010(); 00015 /**Writes to the LED, if defined. 00016 @param state 0 or 1 to power the LED 00017 */ 00018 void write(int state); 00019 /**Take in the current value being output by the device.*/ 00020 int read(); 00021 /**Shorthand for read().*/ 00022 operator int(){return _data;} 00023 /**Initiate interrupts for the rising edge of data. 00024 @param fpr Function pointer to interrupt routine. 00025 */ 00026 void attach_rise(void (*fpr)(void)); 00027 /**Initiate interrupts for the falling edge of data. 00028 @param fpr Function pointer to interrupt routine. 00029 */ 00030 void attach_fall(void (*fpr)(void)); 00031 private: 00032 InterruptIn _data; 00033 DigitalOut *_led_state; 00034 }; 00035 00036 AT42QT1010::AT42QT1010(PinName data) : _data(data) { 00037 _led_state=NULL; 00038 } 00039 00040 AT42QT1010::AT42QT1010(PinName data, PinName led_pin) : _data(data){ 00041 _led_state = (DigitalOut *) new DigitalOut(led_pin); 00042 } 00043 00044 AT42QT1010::~AT42QT1010(){ 00045 if(_led_state!=NULL){ 00046 delete _led_state; 00047 } 00048 } 00049 00050 void AT42QT1010::write(int state){ 00051 if(_led_state!=NULL){ 00052 if(state!=0) (*_led_state)=1; 00053 else (*_led_state)=0; 00054 } 00055 } 00056 00057 int AT42QT1010::read(){ 00058 return _data; 00059 } 00060 00061 void AT42QT1010::attach_rise(void (*fpr)(void)){ 00062 _data.rise(fpr); 00063 } 00064 void AT42QT1010::attach_fall(void (*fpr)(void)){ 00065 _data.fall(fpr); 00066 } 00067 00068 #endif
Generated on Thu Jul 14 2022 13:49:56 by
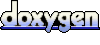