
vvv
Dependencies: C027_Support C027_SupportTest SDFileSystem mbed
Fork of C027_SupportTest by
main.cpp
00001 #include "mbed.h" 00002 00003 //------------------------------------------------------------------------------------ 00004 /* This example was tested on C027-U20 and C027-G35 with the on board modem. 00005 00006 Additionally it was tested with a shield where the SARA-G350/U260/U270 RX/TX/PWRON 00007 is connected to D0/D1/D4 and the GPS SCL/SDA is connected D15/D15. In this 00008 configuration the following platforms were tested (it is likely that others 00009 will work as well) 00010 - U-BLOX: C027-G35, C027-U20, C027-C20 (for shield set define C027_FORCE_SHIELD) 00011 - NXP: LPC1549v2, LPC4088qsb 00012 - Freescale: FRDM-KL05Z, FRDM-KL25Z, FRDM-KL46Z, FRDM-K64F 00013 - STM: NUCLEO-F401RE, NUCLEO-F030R8 00014 mount resistors SB13/14 1k, SB62/63 0R 00015 */ 00016 #include "GPS.h" 00017 #include "MDM.h" 00018 //------------------------------------------------------------------------------------ 00019 // You need to configure these cellular modem / SIM parameters. 00020 // These parameters are ignored for LISA-C200 variants and can be left NULL. 00021 //------------------------------------------------------------------------------------ 00022 //! Set your secret SIM pin here (e.g. "1234"). Check your SIM manual. 00023 #define SIMPIN "08965247" 00024 /*! The APN of your network operator SIM, sometimes it is "internet" check your 00025 contract with the network operator. You can also try to look-up your settings in 00026 google: https://www.google.de/search?q=APN+list */ 00027 #define APN "orange.fr" 00028 //! Set the user name for your APN, or NULL if not needed 00029 #define USERNAME "orange" 00030 //! Set the password for your APN, or NULL if not needed 00031 #define PASSWORD "orange" 00032 //------------------------------------------------------------------------------------ 00033 00034 #define CELLOCATE 00035 00036 int main(void) 00037 { 00038 int ret; 00039 #ifdef LARGE_DATA 00040 char buf[2048] = ""; 00041 #else 00042 char buf[512] = ""; 00043 #endif 00044 00045 // Create the GPS object 00046 #if 1 // use GPSI2C class 00047 GPSI2C gps; 00048 #else // or GPSSerial class 00049 GPSSerial gps; 00050 #endif 00051 // Create the modem object 00052 MDMSerial mdm; 00053 //mdm.setDebug(4); // enable this for debugging issues 00054 // initialize the modem 00055 MDMParser::DevStatus devStatus = {}; 00056 MDMParser::NetStatus netStatus = {}; 00057 bool mdmOk = mdm.init(SIMPIN, &devStatus); 00058 mdm.dumpDevStatus(&devStatus); 00059 if (mdmOk) { 00060 #if 0 00061 // file system API 00062 const char* filename = "File"; 00063 char buf[] = "Hello World"; 00064 printf("writeFile \"%s\"\r\n", buf); 00065 if (mdm.writeFile(filename, buf, sizeof(buf))) 00066 { 00067 memset(buf, 0, sizeof(buf)); 00068 int len = mdm.readFile(filename, buf, sizeof(buf)); 00069 if (len >= 0) 00070 printf("readFile %d \"%.*s\"\r\n", len, len, buf); 00071 mdm.delFile(filename); 00072 } 00073 #endif 00074 00075 // wait until we are connected 00076 mdmOk = mdm.registerNet(&netStatus); 00077 mdm.dumpNetStatus(&netStatus); 00078 } 00079 if (mdmOk) 00080 { 00081 // http://www.geckobeach.com/cellular/secrets/gsmcodes.php 00082 // http://de.wikipedia.org/wiki/USSD-Codes 00083 const char* ussd = "*130#"; // You may get answer "UNKNOWN APPLICATION" 00084 printf("Ussd Send Command %s\r\n", ussd); 00085 ret = mdm.ussdCommand(ussd, buf); 00086 if (ret > 0) 00087 printf("Ussd Got Answer: \"%*s\"\r\n", ret, buf); 00088 00089 // join the internet connection 00090 MDMParser::IP ip = mdm.join(APN,USERNAME,PASSWORD); 00091 if (ip != NOIP) 00092 { 00093 mdm.dumpIp(ip); 00094 printf("Make a Http Post Request\r\n"); 00095 int socket = mdm.socketSocket(MDMParser::IPPROTO_TCP); 00096 if (socket >= 0) 00097 { 00098 mdm.socketSetBlocking(socket, 10000); 00099 if (mdm.socketConnect(socket, "mbed.org", 80)) 00100 { 00101 const char http[] = "GET /media/uploads/mbed_official/hello.txt HTTP/1.0\r\n\r\n"; 00102 mdm.socketSend(socket, http, sizeof(http)-1); 00103 00104 ret = mdm.socketRecv(socket, buf, sizeof(buf)-1); 00105 if (ret > 0) 00106 printf("Socket Recv \"%*s\"\r\n", ret, buf); 00107 mdm.socketClose(socket); 00108 } 00109 mdm.socketFree(socket); 00110 } 00111 00112 int port = 7; 00113 const char* host = "echo.u-blox.com"; 00114 MDMParser::IP ip = mdm.gethostbyname(host); 00115 char data[] = "\r\nxxx Socket Hello World\r\n" 00116 #ifdef LARGE_DATA 00117 "00 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00118 "01 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00119 "02 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00120 "03 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00121 "04 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00122 00123 "05 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00124 "06 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00125 "07 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00126 "08 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00127 "09 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00128 00129 "10 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00130 "11 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00131 "12 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00132 "13 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00133 "14 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00134 00135 "15 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00136 "16 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00137 "17 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00138 "18 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00139 "19 0123456789 0123456789 0123456789 0123456789 0123456789 \r\n" 00140 #endif 00141 "End\r\n"; 00142 00143 printf("Testing TCP sockets with ECHO server\r\n"); 00144 socket = mdm.socketSocket(MDMParser::IPPROTO_TCP); 00145 if (socket >= 0) 00146 { 00147 mdm.socketSetBlocking(socket, 10000); 00148 if (mdm.socketConnect(socket, host, port)) { 00149 memcpy(data, "\r\nTCP", 5); 00150 ret = mdm.socketSend(socket, data, sizeof(data)-1); 00151 if (ret == sizeof(data)-1) { 00152 printf("Socket Send %d \"%s\"\r\n", ret, data); 00153 } 00154 ret = mdm.socketRecv(socket, buf, sizeof(buf)-1); 00155 if (ret >= 0) { 00156 printf("Socket Recv %d \"%.*s\"\r\n", ret, ret, buf); 00157 } 00158 mdm.socketClose(socket); 00159 } 00160 mdm.socketFree(socket); 00161 } 00162 00163 printf("Testing UDP sockets with ECHO server\r\n"); 00164 socket = mdm.socketSocket(MDMParser::IPPROTO_UDP, port); 00165 if (socket >= 0) 00166 { 00167 mdm.socketSetBlocking(socket, 10000); 00168 memcpy(data, "\r\nUDP", 5); 00169 ret = mdm.socketSendTo(socket, ip, port, data, sizeof(data)-1); 00170 if (ret == sizeof(data)-1) { 00171 printf("Socket SendTo %s:%d " IPSTR " %d \"%s\"\r\n", host, port, IPNUM(ip), ret, data); 00172 } 00173 ret = mdm.socketRecvFrom(socket, &ip, &port, buf, sizeof(buf)-1); 00174 if (ret >= 0) { 00175 printf("Socket RecvFrom " IPSTR ":%d %d \"%.*s\" \r\n", IPNUM(ip),port, ret, ret,buf); 00176 } 00177 mdm.socketFree(socket); 00178 } 00179 00180 // disconnect 00181 mdm.disconnect(); 00182 } 00183 } 00184 printf("SMS and GPS Loop\r\n"); 00185 char link[128] = ""; 00186 unsigned int i = 0xFFFFFFFF; 00187 const int wait = 100; 00188 bool abort = false; 00189 #ifdef CELLOCATE 00190 const int sensorMask = 3; // Hybrid: GNSS + CellLocate 00191 const int timeoutMargin = 5; // seconds 00192 const int submitPeriod = 60; // 1 minutes in seconds 00193 const int targetAccuracy = 1; // meters 00194 unsigned int j = submitPeriod * 1000/wait; 00195 bool cellLocWait = false; 00196 MDMParser::CellLocData loc; 00197 00198 //Token can be released from u-blox site, when you got one replace "TOKEN" below 00199 if (!mdm.cellLocSrvHttp("TOKEN")) 00200 mdm.cellLocSrvUdp(); 00201 mdm.cellLocConfigSensor(1); // Deep scan mode 00202 //mdm.cellUnsolIndication(1); 00203 #endif 00204 //DigitalOut led(LED1); 00205 while (!abort) { 00206 // led = !led; 00207 #ifndef CELLOCATE 00208 while ((ret = gps.getMessage(buf, sizeof(buf))) > 0) 00209 { 00210 int len = LENGTH(ret); 00211 //printf("NMEA: %.*s\r\n", len-2, msg); 00212 if ((PROTOCOL(ret) == GPSParser::NMEA) && (len > 6)) 00213 { 00214 // talker is $GA=Galileo $GB=Beidou $GL=Glonass $GN=Combined $GP=GPS 00215 if ((buf[0] == '$') || buf[1] == 'G') { 00216 #define _CHECK_TALKER(s) ((buf[3] == s[0]) && (buf[4] == s[1]) && (buf[5] == s[2])) 00217 if (_CHECK_TALKER("GLL")) { 00218 double la = 0, lo = 0; 00219 char ch; 00220 if (gps.getNmeaAngle(1,buf,len,la) && 00221 gps.getNmeaAngle(3,buf,len,lo) && 00222 gps.getNmeaItem(6,buf,len,ch) && ch == 'A') 00223 { 00224 printf("GPS Location: %.5f %.5f\r\n", la, lo); 00225 sprintf(link, "I am here!\n" 00226 "https://maps.google.com/?q=%.5f,%.5f", la, lo); 00227 } 00228 } else if (_CHECK_TALKER("GGA") || _CHECK_TALKER("GNS") ) { 00229 double a = 0; 00230 if (gps.getNmeaItem(9,buf,len,a)) // altitude msl [m] 00231 printf("GPS Altitude: %.1f\r\n", a); 00232 } else if (_CHECK_TALKER("VTG")) { 00233 double s = 0; 00234 if (gps.getNmeaItem(7,buf,len,s)) // speed [km/h] 00235 printf("GPS Speed: %.1f\r\n", s); 00236 } 00237 } 00238 } 00239 } 00240 #endif 00241 #ifdef CELLOCATE 00242 if (mdmOk && (j++ == submitPeriod * 1000/wait)) { 00243 j=0; 00244 printf("CellLocate Request\r\n"); 00245 mdm.cellLocRequest(sensorMask, submitPeriod-timeoutMargin, targetAccuracy); 00246 cellLocWait = true; 00247 } 00248 if (cellLocWait && mdm.cellLocGet(&loc)){ 00249 cellLocWait = false; 00250 printf("CellLocate position received, sensor_used: %d, \r\n", loc.sensorUsed ); 00251 printf(" latitude: %0.5f, longitude: %0.5f, altitute: %d\r\n", loc.latitue, loc.longitude, loc.altitutude); 00252 if (loc.sensorUsed == 1) 00253 printf(" uncertainty: %d, speed: %d, direction: %d, vertical_acc: %d, satellite used: %d \r\n", loc.uncertainty,loc.speed,loc.direction,loc.verticalAcc,loc.svUsed); 00254 if (loc.sensorUsed == 1 || loc.sensorUsed == 2) 00255 sprintf(link, "I am here!\n" 00256 "https://maps.google.com/?q=%.5f,%.5f", loc.latitue, loc.longitude); 00257 } 00258 if (cellLocWait && (j%100 == 0 )) 00259 printf("Waiting for CellLocate...\r\n"); 00260 #endif 00261 if (mdmOk && (i++ == 5000/wait)) { 00262 i = 0; 00263 // check the network status 00264 if (mdm.checkNetStatus(&netStatus)) { 00265 mdm.dumpNetStatus(&netStatus, fprintf, stdout); 00266 } 00267 00268 // checking unread sms 00269 int ix[8]; 00270 int n = mdm.smsList("REC UNREAD", ix, 8); 00271 if (8 < n) n = 8; 00272 while (0 < n--) 00273 { 00274 char num[32]; 00275 printf("Unread SMS at index %d\r\n", ix[n]); 00276 if (mdm.smsRead(ix[n], num, buf, sizeof(buf))) { 00277 printf("Got SMS from \"%s\" with text \"%s\"\r\n", num, buf); 00278 printf("Delete SMS at index %d\r\n", ix[n]); 00279 mdm.smsDelete(ix[n]); 00280 // provide a reply 00281 const char* reply = "Hello my friend"; 00282 if (strstr(buf, /*w*/"here are you")) 00283 reply = *link ? link : "I don't know"; // reply wil location link 00284 else if (strstr(buf, /*s*/"hutdown")) 00285 abort = true, reply = "bye bye"; 00286 printf("Send SMS reply \"%s\" to \"%s\"\r\n", reply, num); 00287 mdm.smsSend(num, reply); 00288 } 00289 } 00290 } 00291 wait_ms(wait); 00292 } 00293 gps.powerOff(); 00294 mdm.powerOff(); 00295 return 0; 00296 }
Generated on Tue Jul 12 2022 20:39:12 by
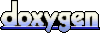