
sd
Dependencies: 4DGL-uLCD-SE PinDetect SDFileSystem mbed wave_player
Fork of WavePlayer_HelloWorld by
main.cpp
00001 //Jason Mar 00002 #include "mbed.h" 00003 #include "SDFileSystem.h" 00004 #include "wave_player.h" 00005 #include "uLCD_4DGL.h" 00006 #include "PinDetect.h" 00007 #include <string> 00008 #include <vector> 00009 00010 //initialize all pins 00011 SDFileSystem sd(p5, p6, p7, p8, p9, "sd"); //SD card 00012 uLCD_4DGL uLCD(p28, p27, p29); 00013 PinDetect pb1(p24); 00014 PinDetect pb2(p23); 00015 PinDetect pb3(p22); 00016 PinDetect pb4(p21); 00017 00018 AnalogOut DACout(p18); 00019 00020 wave_player waver(&DACout); 00021 //unused pins 00022 DigitalOut P10(p10); 00023 DigitalOut P11(p11); 00024 DigitalOut P12(p12); 00025 DigitalOut P13(p13); 00026 DigitalOut P14(p14); 00027 DigitalOut P15(p15); 00028 DigitalOut P16(p16); 00029 DigitalOut P17(p17); 00030 DigitalOut P19(p19); 00031 DigitalOut P20(p20); 00032 00033 vector<string> filenames; //filenames are stored in a vector string 00034 int volatile selection = 0; 00035 int volatile totalSongs = 0; 00036 bool play = false; 00037 bool *playptr = &play; 00038 00039 //implement pushbuttons 00040 void pb1_hit_callback (void) 00041 { 00042 play = !play; 00043 } 00044 void pb2_hit_callback (void) 00045 { 00046 selection++; 00047 if(selection >= totalSongs) 00048 selection = 0; 00049 } 00050 void pb3_hit_callback (void) 00051 { 00052 selection--; 00053 if(selection < 0) 00054 selection = totalSongs - 1; 00055 } 00056 void pb4_hit_callback (void) 00057 { 00058 int *vol = waver.getVolume(); 00059 *vol = *vol + 1; 00060 if (*vol >= 16){ 00061 *vol = 0; 00062 } 00063 } 00064 //reads the file names 00065 void read_file_names(char *dir) 00066 { 00067 DIR *dp; 00068 struct dirent *dirp; 00069 dp = opendir(dir); 00070 //read all directory and file names in current directory into filename vector 00071 while((dirp = readdir(dp)) != NULL) { 00072 filenames.push_back(string(dirp->d_name)); 00073 totalSongs++; 00074 } 00075 closedir(dp); 00076 } 00077 00078 00079 int main() 00080 { 00081 //initialize the buttons 00082 pb1.mode(PullUp); 00083 pb2.mode(PullUp); 00084 pb3.mode(PullUp); 00085 pb4.mode(PullUp); 00086 00087 // Delay for initial pullup to take effect 00088 wait(.01); 00089 00090 // Setup Interrupt callback functions for a pb hit 00091 pb1.attach_deasserted(&pb1_hit_callback); 00092 pb2.attach_deasserted(&pb2_hit_callback); 00093 pb3.attach_deasserted(&pb3_hit_callback); 00094 pb4.attach_deasserted(&pb4_hit_callback); 00095 00096 // Start sampling pb inputs using interrupts 00097 pb1.setSampleFrequency(); 00098 pb2.setSampleFrequency(); 00099 pb3.setSampleFrequency(); 00100 pb4.setSampleFrequency(); 00101 00102 //check for SD card insertion, if no sd card print Insert SD Card 00103 bool SDinsert = false; 00104 while(SDinsert == false){ 00105 SDinsert = sd.sd_inserted(); 00106 if(SDinsert == false){ 00107 uLCD.locate(0,1); 00108 uLCD.printf("Insert SD Card"); 00109 } 00110 else { 00111 sd.disk_initialize(); 00112 uLCD.cls(); 00113 } 00114 } 00115 FILE *file; 00116 read_file_names("/sd/myMusic"); 00117 00118 00119 string song; 00120 string dir; 00121 int c = 0; 00122 int prevfile = -1; 00123 while(1) { 00124 //prints the directory of songs and does not refresh until a pushbutton changes the selection 00125 if(c == 0 || selection != prevfile){ 00126 prevfile = selection; 00127 uLCD.cls(); 00128 uLCD.locate(0,0); 00129 //lists the directory songs in white and the selected song in red 00130 for(int i=0;i<totalSongs;i++){ 00131 if(i != selection){ 00132 int l=filenames[i].size(); 00133 uLCD.locate(0,i); 00134 uLCD.color(WHITE); 00135 //substrings the .wav out of the print 00136 uLCD.printf("%s",filenames[i].substr(0,l-4)); 00137 } 00138 else { 00139 int l=filenames[selection].size(); 00140 uLCD.locate(0,selection); 00141 uLCD.color(RED); 00142 uLCD.printf("%s",filenames[selection].substr(0,l-4)); 00143 } 00144 } 00145 } 00146 c++; 00147 00148 if(play==true) { 00149 //concatenates directory with songname 00150 dir="/sd/myMusic"; 00151 song = dir + "/" + filenames[selection]; 00152 //reads the wav file 00153 file = fopen(song.c_str(), "r"); 00154 //clears the screen to print song playiing 00155 uLCD.cls(); 00156 int l=filenames[selection].size(); 00157 uLCD.locate(0,0); 00158 uLCD.printf("%s",filenames[selection].substr(0,l-4)); 00159 uLCD.locate(0,1); 00160 uLCD.printf("%s","Now Playing"); 00161 waver.play(file,playptr); 00162 play=false; 00163 fclose(file); 00164 uLCD.cls(); 00165 //reprint the menu as it was before the song started 00166 for(int i=0;i<totalSongs;i++){ 00167 if(i != selection){ 00168 int l=filenames[i].size(); 00169 uLCD.locate(0,i); 00170 uLCD.color(WHITE); 00171 uLCD.printf("%s",filenames[i].substr(0,l-4)); 00172 } 00173 else { 00174 int l=filenames[selection].size(); 00175 uLCD.locate(0,selection); 00176 uLCD.color(RED); 00177 uLCD.printf("%s",filenames[selection].substr(0,l-4)); 00178 } 00179 } 00180 } 00181 else 00182 wait(0.3); 00183 } 00184 }
Generated on Tue Jul 12 2022 22:51:58 by
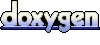