
Revisions to support JFET LoRa module
Dependencies: mbed LoRaWAN-lib SX1272Libjlc
Fork of LoRaWAN-demo-72_tjm by
utilities.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2013 Semtech 00008 00009 Description: Helper functions implementation 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #ifndef __UTILITIES_H__ 00016 #define __UTILITIES_H__ 00017 00018 /*! 00019 * \brief Returns the minimum value betwen a and b 00020 * 00021 * \param [IN] a 1st value 00022 * \param [IN] b 2nd value 00023 * \retval minValue Minimum value 00024 */ 00025 #define MIN( a, b ) ( ( ( a ) < ( b ) ) ? ( a ) : ( b ) ) 00026 00027 /*! 00028 * \brief Returns the maximum value betwen a and b 00029 * 00030 * \param [IN] a 1st value 00031 * \param [IN] b 2nd value 00032 * \retval maxValue Maximum value 00033 */ 00034 #define MAX( a, b ) ( ( ( a ) > ( b ) ) ? ( a ) : ( b ) ) 00035 00036 /*! 00037 * \brief Returns 2 raised to the power of n 00038 * 00039 * \param [IN] n power value 00040 * \retval result of raising 2 to the power n 00041 */ 00042 #define POW2( n ) ( 1 << n ) 00043 00044 /*! 00045 * \brief Initializes the pseudo ramdom generator initial value 00046 * 00047 * \param [IN] seed Pseudo ramdom generator initial value 00048 */ 00049 void srand1( uint32_t seed ); 00050 00051 /*! 00052 * \brief Computes a random number between min and max 00053 * 00054 * \param [IN] min range minimum value 00055 * \param [IN] max range maximum value 00056 * \retval random random value in range min..max 00057 */ 00058 int32_t randr( int32_t min, int32_t max ); 00059 00060 /*! 00061 * \brief Copies size elements of src array to dst array 00062 * 00063 * \remark STM32 Standard memcpy function only works on pointers that are aligned 00064 * 00065 * \param [OUT] dst Destination array 00066 * \param [IN] src Source array 00067 * \param [IN] size Number of bytes to be copied 00068 */ 00069 void memcpy1( uint8_t *dst, const uint8_t *src, uint16_t size ); 00070 00071 /*! 00072 * \brief Copies size elements of src array to dst array reversing the byte order 00073 * 00074 * \param [OUT] dst Destination array 00075 * \param [IN] src Source array 00076 * \param [IN] size Number of bytes to be copied 00077 */ 00078 void memcpyr( uint8_t *dst, const uint8_t *src, uint16_t size ); 00079 00080 /*! 00081 * \brief Set size elements of dst array with value 00082 * 00083 * \remark STM32 Standard memset function only works on pointers that are aligned 00084 * 00085 * \param [OUT] dst Destination array 00086 * \param [IN] value Default value 00087 * \param [IN] size Number of bytes to be copied 00088 */ 00089 void memset1( uint8_t *dst, uint8_t value, uint16_t size ); 00090 00091 /*! 00092 * \brief Converts a nibble to an hexadecimal character 00093 * 00094 * \param [IN] a Nibble to be converted 00095 * \retval hexChar Converted hexadecimal character 00096 */ 00097 int8_t Nibble2HexChar( uint8_t a ); 00098 00099 #endif // __UTILITIES_H__
Generated on Wed Jul 13 2022 08:37:14 by
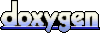