
うおーるぼっと用プログラム Wiiリモコンからのダイレクト操作モードのみ BlueUSBをベースに使用しています。
Dependencies: BD6211F mbed SimpleFilter
USBHost.h
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #ifndef USBHOST_H 00025 #define USBHOST_H 00026 00027 #ifndef u8 00028 typedef unsigned char u8; 00029 typedef unsigned short u16; 00030 typedef unsigned long u32; 00031 00032 typedef char s8; 00033 typedef short s16; 00034 typedef char s32; 00035 #endif 00036 00037 #define ENDPOINT_CONTROL 0 00038 #define ENDPOINT_ISOCRONOUS 1 00039 #define ENDPOINT_BULK 2 00040 #define ENDPOINT_INTERRUPT 3 00041 00042 #define DESCRIPTOR_TYPE_DEVICE 1 00043 #define DESCRIPTOR_TYPE_CONFIGURATION 2 00044 #define DESCRIPTOR_TYPE_STRING 3 00045 #define DESCRIPTOR_TYPE_INTERFACE 4 00046 #define DESCRIPTOR_TYPE_ENDPOINT 5 00047 00048 #define DESCRIPTOR_TYPE_HID 0x21 00049 #define DESCRIPTOR_TYPE_REPORT 0x22 00050 #define DESCRIPTOR_TYPE_PHYSICAL 0x23 00051 #define DESCRIPTOR_TYPE_HUB 0x29 00052 00053 enum USB_CLASS_CODE 00054 { 00055 CLASS_DEVICE, 00056 CLASS_AUDIO, 00057 CLASS_COMM_AND_CDC_CONTROL, 00058 CLASS_HID, 00059 CLASS_PHYSICAL = 0x05, 00060 CLASS_STILL_IMAGING, 00061 CLASS_PRINTER, 00062 CLASS_MASS_STORAGE, 00063 CLASS_HUB, 00064 CLASS_CDC_DATA, 00065 CLASS_SMART_CARD, 00066 CLASS_CONTENT_SECURITY = 0x0D, 00067 CLASS_VIDEO = 0x0E, 00068 CLASS_DIAGNOSTIC_DEVICE = 0xDC, 00069 CLASS_WIRELESS_CONTROLLER = 0xE0, 00070 CLASS_MISCELLANEOUS = 0xEF, 00071 CLASS_APP_SPECIFIC = 0xFE, 00072 CLASS_VENDOR_SPECIFIC = 0xFF 00073 }; 00074 00075 #define DEVICE_TO_HOST 0x80 00076 #define HOST_TO_DEVICE 0x00 00077 #define REQUEST_TYPE_CLASS 0x20 00078 #define RECIPIENT_DEVICE 0x00 00079 #define RECIPIENT_INTERFACE 0x01 00080 #define RECIPIENT_ENDPOINT 0x02 00081 #define RECIPIENT_OTHER 0x03 00082 00083 #define GET_STATUS 0 00084 #define CLEAR_FEATURE 1 00085 #define SET_FEATURE 3 00086 #define SET_ADDRESS 5 00087 #define GET_DESCRIPTOR 6 00088 #define SET_DESCRIPTOR 7 00089 #define GET_CONFIGURATION 8 00090 #define SET_CONFIGURATION 9 00091 #define GET_INTERFACE 10 00092 #define SET_INTERFACE 11 00093 #define SYNCH_FRAME 11 00094 00095 // -5 is nak 00096 /* 00097 0010 ACK Handshake 00098 1010 NAK Handshake 00099 1110 STALL Handshake 00100 0110 NYET (No Response Yet) 00101 */ 00102 00103 #define IO_PENDING -100 00104 #define ERR_ENDPOINT_NONE_LEFT -101 00105 #define ERR_ENDPOINT_NOT_FOUND -102 00106 #define ERR_DEVICE_NOT_FOUND -103 00107 #define ERR_DEVICE_NONE_LEFT -104 00108 #define ERR_HUB_INIT_FAILED -105 00109 #define ERR_INTERFACE_NOT_FOUND -106 00110 00111 typedef struct 00112 { 00113 u8 bLength; 00114 u8 bDescriptorType; 00115 u16 bcdUSB; 00116 u8 bDeviceClass; 00117 u8 bDeviceSubClass; 00118 u8 bDeviceProtocol; 00119 u8 bMaxPacketSize; 00120 u16 idVendor; 00121 u16 idProduct; 00122 u16 bcdDevice; // version 00123 u8 iManufacturer; 00124 u8 iProduct; 00125 u8 iSerialNumber; 00126 u8 bNumConfigurations; 00127 } DeviceDescriptor; // 16 bytes 00128 00129 typedef struct 00130 { 00131 u8 bLength; 00132 u8 bDescriptorType; 00133 u16 wTotalLength; 00134 u8 bNumInterfaces; 00135 u8 bConfigurationValue; // Value to use as an argument to select this configuration 00136 u8 iConfiguration; // Index of String Descriptor describing this configuration 00137 u8 bmAttributes; // Bitmap D7 Reserved, set to 1. (USB 1.0 Bus Powered),D6 Self Powered,D5 Remote Wakeup,D4..0 = 0 00138 u8 bMaxPower; // Maximum Power Consumption in 2mA units 00139 } ConfigurationDescriptor; 00140 00141 typedef struct 00142 { 00143 u8 bLength; 00144 u8 bDescriptorType; 00145 u8 bInterfaceNumber; 00146 u8 bAlternateSetting; 00147 u8 bNumEndpoints; 00148 u8 bInterfaceClass; 00149 u8 bInterfaceSubClass; 00150 u8 bInterfaceProtocol; 00151 u8 iInterface; // Index of String Descriptor Describing this interface 00152 } InterfaceDescriptor; 00153 00154 typedef struct 00155 { 00156 u8 bLength; 00157 u8 bDescriptorType; 00158 u8 bEndpointAddress; // Bits 0:3 endpoint, Bits 7 Direction 0 = Out, 1 = In (Ignored for Control Endpoints) 00159 u8 bmAttributes; // Bits 0:1 00 = Control, 01 = Isochronous, 10 = Bulk, 11 = Interrupt 00160 u16 wMaxPacketSize; 00161 u8 bInterval; // Interval for polling endpoint data transfers. 00162 } EndpointDescriptor; 00163 00164 typedef struct { 00165 u8 bLength; 00166 u8 bDescriptorType; 00167 u16 bcdHID; 00168 u8 bCountryCode; 00169 u8 bNumDescriptors; 00170 u8 bDescriptorType2; 00171 u16 wDescriptorLength; 00172 } HIDDescriptor; 00173 00174 //============================================================================ 00175 //============================================================================ 00176 00177 00178 void USBInit(); 00179 void USBLoop(); 00180 u8* USBGetBuffer(u32* len); 00181 00182 // Optional callback for transfers, called at interrupt time 00183 typedef void (*USBCallback)(int device, int endpoint, int status, u8* data, int len, void* userData); 00184 00185 // Transfers 00186 int USBControlTransfer(int device, int request_type, int request, int value, int index, u8* data, int length, USBCallback callback = 0, void* userData = 0); 00187 int USBInterruptTransfer(int device, int ep, u8* data, int length, USBCallback callback = 0, void* userData = 0); 00188 int USBBulkTransfer(int device, int ep, u8* data, int length, USBCallback callback = 0, void* userData = 0); 00189 00190 // Standard Device methods 00191 int GetDescriptor(int device, int descType, int descIndex, u8* data, int length); 00192 int GetString(int device, int index, char* dst, int length); 00193 int SetAddress(int device, int new_addr); 00194 int SetConfiguration(int device, int configNum); 00195 int SetInterface(int device, int ifNum, int altNum); 00196 00197 // Implemented to notify app of the arrival of a device 00198 void OnLoadDevice(int device, DeviceDescriptor* deviceDesc, InterfaceDescriptor* interfaceDesc); 00199 00200 #endif
Generated on Thu Jul 14 2022 13:01:38 by
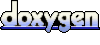