
うおーるぼっと用プログラム Wiiリモコンからのダイレクト操作モードのみ BlueUSBをベースに使用しています。
Dependencies: BD6211F mbed SimpleFilter
AutoEvents.cpp
00001 00002 /* 00003 Copyright (c) 2010 Peter Barrett 00004 00005 Permission is hereby granted, free of charge, to any person obtaining a copy 00006 of this software and associated documentation files (the "Software"), to deal 00007 in the Software without restriction, including without limitation the rights 00008 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 copies of the Software, and to permit persons to whom the Software is 00010 furnished to do so, subject to the following conditions: 00011 00012 The above copyright notice and this permission notice shall be included in 00013 all copies or substantial portions of the Software. 00014 00015 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 THE SOFTWARE. 00022 */ 00023 00024 #include "mbed.h" 00025 #include "USBHost.h" 00026 #include "Utils.h" 00027 00028 #define AUTOEVT(_class,_subclass,_protocol) (((_class) << 16) | ((_subclass) << 8) | _protocol) 00029 #define AUTO_KEYBOARD AUTOEVT(CLASS_HID,1,1) 00030 #define AUTO_MOUSE AUTOEVT(CLASS_HID,1,2) 00031 00032 u8 auto_mouse[4]; // buttons,dx,dy,scroll 00033 u8 auto_keyboard[8]; // modifiers,reserved,keycode1..keycode6 00034 u8 auto_joystick[4]; // x,y,buttons,throttle 00035 00036 void AutoEventCallback(int device, int endpoint, int status, u8* data, int len, void* userData) 00037 { 00038 int evt = (int)userData; 00039 switch (evt) 00040 { 00041 case AUTO_KEYBOARD: 00042 printf("AUTO_KEYBOARD "); 00043 break; 00044 case AUTO_MOUSE: 00045 printf("AUTO_MOUSE "); 00046 break; 00047 default: 00048 printf("HUH "); 00049 } 00050 printfBytes("data",data,len); 00051 USBInterruptTransfer(device,endpoint,data,len,AutoEventCallback,userData); 00052 } 00053 00054 // Establish transfers for interrupt events 00055 void AddAutoEvent(int device, InterfaceDescriptor* id, EndpointDescriptor* ed) 00056 { 00057 if ((ed->bmAttributes & 3) != ENDPOINT_INTERRUPT || !(ed->bEndpointAddress & 0x80)) 00058 return; 00059 00060 // Make automatic interrupt enpoints for known devices 00061 u32 evt = AUTOEVT(id->bInterfaceClass,id->bInterfaceSubClass,id->bInterfaceProtocol); 00062 u8* dst = 0; 00063 int len; 00064 switch (evt) 00065 { 00066 case AUTO_MOUSE: 00067 dst = auto_mouse; 00068 len = sizeof(auto_mouse); 00069 break; 00070 case AUTO_KEYBOARD: 00071 dst = auto_keyboard; 00072 len = sizeof(auto_keyboard); 00073 break; 00074 default: 00075 printf("Interrupt endpoint %02X %08X\n",ed->bEndpointAddress,evt); 00076 break; 00077 } 00078 if (dst) 00079 { 00080 printf("Auto Event for %02X %08X\n",ed->bEndpointAddress,evt); 00081 USBInterruptTransfer(device,ed->bEndpointAddress,dst,len,AutoEventCallback,(void*)evt); 00082 } 00083 } 00084 00085 void PrintString(int device, int i) 00086 { 00087 u8 buffer[256]; 00088 int le = GetDescriptor(device,DESCRIPTOR_TYPE_STRING,i,buffer,255); 00089 if (le < 0) 00090 return; 00091 char* dst = (char*)buffer; 00092 for (int j = 2; j < le; j += 2) 00093 *dst++ = buffer[j]; 00094 *dst = 0; 00095 printf("%d:%s\n",i,(const char*)buffer); 00096 } 00097 00098 // Walk descriptors and create endpoints for a given device 00099 int StartAutoEvent(int device, int configuration, int interfaceNumber) 00100 { 00101 u8 buffer[255]; 00102 int err = GetDescriptor(device,DESCRIPTOR_TYPE_CONFIGURATION,0,buffer,255); 00103 if (err < 0) 00104 return err; 00105 00106 int len = buffer[2] | (buffer[3] << 8); 00107 u8* d = buffer; 00108 u8* end = d + len; 00109 while (d < end) 00110 { 00111 if (d[1] == DESCRIPTOR_TYPE_INTERFACE) 00112 { 00113 InterfaceDescriptor* id = (InterfaceDescriptor*)d; 00114 if (id->bInterfaceNumber == interfaceNumber) 00115 { 00116 d += d[0]; 00117 while (d < end && d[1] != DESCRIPTOR_TYPE_INTERFACE) 00118 { 00119 if (d[1] == DESCRIPTOR_TYPE_ENDPOINT) 00120 AddAutoEvent(device,id,(EndpointDescriptor*)d); 00121 d += d[0]; 00122 } 00123 } 00124 } 00125 d += d[0]; 00126 } 00127 return 0; 00128 } 00129 00130 // Implemented in main.cpp 00131 int OnDiskInsert(int device); 00132 00133 // Implemented in TestShell.cpp 00134 int OnBluetoothInsert(int device); 00135 00136 void OnLoadDevice(int device, DeviceDescriptor* deviceDesc, InterfaceDescriptor* interfaceDesc) 00137 { 00138 printf("LoadDevice %d %02X:%02X:%02X\n",device,interfaceDesc->bInterfaceClass,interfaceDesc->bInterfaceSubClass,interfaceDesc->bInterfaceProtocol); 00139 char s[128]; 00140 for (int i = 1; i < 3; i++) 00141 { 00142 if (GetString(device,i,s,sizeof(s)) < 0) 00143 break; 00144 printf("%d: %s\n",i,s); 00145 } 00146 00147 switch (interfaceDesc->bInterfaceClass) 00148 { 00149 case CLASS_MASS_STORAGE: 00150 if (interfaceDesc->bInterfaceSubClass == 0x06 && interfaceDesc->bInterfaceProtocol == 0x50) 00151 OnDiskInsert(device); // it's SCSI! 00152 break; 00153 case CLASS_WIRELESS_CONTROLLER: 00154 if (interfaceDesc->bInterfaceSubClass == 0x01 && interfaceDesc->bInterfaceProtocol == 0x01) 00155 OnBluetoothInsert(device); // it's bluetooth! 00156 break; 00157 default: 00158 StartAutoEvent(device,1,0); 00159 break; 00160 } 00161 }
Generated on Thu Jul 14 2022 13:01:38 by
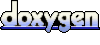