Simple IoT Board用のライブラリです。 ESP8266ライブラリの軽量化 送信のみのソフトシリアルライブラリを含んでいます。
Dependents: SITB_HttpGetSample SITB_IFTTTSample SITB_INA226PRC AmbientExampleSITB ... more
TCPSocketConnection.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 #include "TCPSocketConnection.h" 00019 #include <cstring> 00020 #include <algorithm> 00021 00022 using std::memset; 00023 using std::memcpy; 00024 00025 //Debug is disabled by default 00026 #if 1 00027 #define DBG(x, ...) printf("[TCPConnection : DBG]"x" \t[%s,%d]\r\n", ##__VA_ARGS__,__FILE__,__LINE__); 00028 #define WARN(x, ...) printf("[TCPConnection: WARN]"x" \t[%s,%d]\r\n", ##__VA_ARGS__,__FILE__,__LINE__); 00029 #define ERR(x, ...) printf("[TCPConnection : ERR]"x" \t[%s,%d]\r\n", ##__VA_ARGS__,__FILE__,__LINE__); 00030 #else 00031 #define DBG(x, ...) 00032 #define WARN(x, ...) 00033 #define ERR(x, ...) 00034 #endif 00035 00036 TCPSocketConnection::TCPSocketConnection() : 00037 _is_connected(false) 00038 { 00039 } 00040 00041 int TCPSocketConnection::connect(const char* host, const int port) 00042 { 00043 // if (init_socket(SOCK_STREAM) < 0) 00044 // return -1; 00045 // 00046 if (set_address(host, port) != 0) 00047 return -1; 00048 // 00049 // if (lwip_connect(_sock_fd, (const struct sockaddr *) &_remoteHost, sizeof(_remoteHost)) < 0) { 00050 // close(); 00051 // return -1; 00052 // } 00053 // _is_connected = true; 00054 00055 _is_connected = ESP8266->start(ESP_TCP_TYPE,_ipAddress,_port); 00056 if(_is_connected) { //success 00057 return 0; 00058 } else { // fail 00059 return -1; 00060 } 00061 } 00062 00063 bool TCPSocketConnection::is_connected(void) 00064 { 00065 return _is_connected; 00066 } 00067 00068 int TCPSocketConnection::send(char* data, int length) 00069 { 00070 if (!_is_connected) { 00071 ERR("TCPSocketConnection::receive() - _is_connected is false : you cant receive data until you connect to a socket!"); 00072 return -1; 00073 } 00074 Timer tmr; 00075 int idx = 0; 00076 tmr.start(); 00077 while ((tmr.read_ms() < _timeout) || _blocking) { 00078 00079 idx += wifi->send(data, length); 00080 00081 if (idx == length) 00082 return idx; 00083 } 00084 return (idx == 0) ? -1 : idx; 00085 00086 //return wifi->send(data,length); 00087 // 00088 // if (!_blocking) { 00089 // TimeInterval timeout(_timeout); 00090 // if (wait_writable(timeout) != 0) 00091 // return -1; 00092 // } 00093 // 00094 // int n = lwip_send(_sock_fd, data, length, 0); 00095 // _is_connected = (n != 0); 00096 // 00097 // return n; 00098 00099 } 00100 00101 // -1 if unsuccessful, else number of bytes written 00102 int TCPSocketConnection::send_all(char* data, int length) 00103 { 00104 // if ((_sock_fd < 0) || !_is_connected) 00105 // return -1; 00106 // 00107 // int writtenLen = 0; 00108 // TimeInterval timeout(_timeout); 00109 // while (writtenLen < length) { 00110 // if (!_blocking) { 00111 // // Wait for socket to be writeable 00112 // if (wait_writable(timeout) != 0) 00113 // return writtenLen; 00114 // } 00115 // 00116 // int ret = lwip_send(_sock_fd, data + writtenLen, length - writtenLen, 0); 00117 // if (ret > 0) { 00118 // writtenLen += ret; 00119 // continue; 00120 // } else if (ret == 0) { 00121 // _is_connected = false; 00122 // return writtenLen; 00123 // } else { 00124 // return -1; //Connnection error 00125 // } 00126 // } 00127 // return writtenLen; 00128 return send(data,length); // just remap to send 00129 } 00130 00131 int TCPSocketConnection::receive(char* buffer, int length) 00132 { 00133 if (!_is_connected) { 00134 ERR("TCPSocketConnection::receive() - _is_connected is false : you cant receive data until you connect to a socket!"); 00135 return -1; 00136 } 00137 Timer tmr; 00138 int idx = 0; 00139 int nb_available = 0; 00140 int time = -1; 00141 00142 //make this the non-blocking case and return if <= 0 00143 // remember to change the config to blocking 00144 // if ( ! _blocking) { 00145 // if ( wifi.readable <= 0 ) { 00146 // return (wifi.readable); 00147 // } 00148 // } 00149 //--- 00150 tmr.start(); 00151 if (_blocking) { 00152 while (1) { 00153 nb_available = wifi->readable(); 00154 if (nb_available != 0) { 00155 break; 00156 } 00157 } 00158 } 00159 //--- 00160 // blocking case 00161 else { 00162 tmr.reset(); 00163 00164 while (time < _timeout) { 00165 nb_available = wifi->readable(); 00166 if (nb_available < 0) return nb_available; 00167 if (nb_available > 0) break ; 00168 time = tmr.read_ms(); 00169 } 00170 00171 if (nb_available == 0) return nb_available; 00172 } 00173 00174 // change this to < 20 mS timeout per byte to detect end of packet gap 00175 // this may not work due to buffering at the UART interface 00176 tmr.reset(); 00177 // while ( tmr.read_ms() < 20 ) { 00178 // if ( wifi.readable() && (idx < length) ) { 00179 // buffer[idx++] = wifi->getc(); 00180 // tmr.reset(); 00181 // } 00182 // if ( idx == length ) { 00183 // break; 00184 // } 00185 // } 00186 //--- 00187 while (time < _timeout) { 00188 00189 nb_available = wifi->readable(); 00190 //for (int i = 0; i < min(nb_available, length); i++) { 00191 for (int i = 0; i < min(nb_available, (length-idx)); i++) { 00192 buffer[idx] = wifi->getc(); 00193 idx++; 00194 } 00195 if (idx == length) { 00196 break; 00197 } 00198 time = tmr.read_ms(); 00199 } 00200 //--- 00201 return (idx == 0) ? -1 : idx; 00202 00203 //************************ original code below 00204 // 00205 // if (!_blocking) { 00206 // TimeInterval timeout(_timeout); 00207 // if (wait_readable(timeout) != 0) 00208 // return -1; 00209 // } 00210 // 00211 // int n = lwip_recv(_sock_fd, data, length, 0); 00212 // _is_connected = (n != 0); 00213 // 00214 // return n; 00215 00216 } 00217 00218 // -1 if unsuccessful, else number of bytes received 00219 int TCPSocketConnection::receive_all(char* data, int length) 00220 { 00221 //ERR("receive_all() not yet implimented"); 00222 // if ((_sock_fd < 0) || !_is_connected) 00223 // return -1; 00224 // 00225 // int readLen = 0; 00226 // TimeInterval timeout(_timeout); 00227 // while (readLen < length) { 00228 // if (!_blocking) { 00229 // //Wait for socket to be readable 00230 // if (wait_readable(timeout) != 0) 00231 // return readLen; 00232 // } 00233 // 00234 // int ret = lwip_recv(_sock_fd, data + readLen, length - readLen, 0); 00235 // if (ret > 0) { 00236 // readLen += ret; 00237 // } else if (ret == 0) { 00238 // _is_connected = false; 00239 // return readLen; 00240 // } else { 00241 // return -1; //Connnection error 00242 // } 00243 // } 00244 // return readLen; 00245 receive(data,length); 00246 }
Generated on Tue Jul 12 2022 18:13:35 by
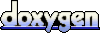