Simple IoT Board用のライブラリです。 ESP8266ライブラリの軽量化 送信のみのソフトシリアルライブラリを含んでいます。
Dependents: SITB_HttpGetSample SITB_IFTTTSample SITB_INA226PRC AmbientExampleSITB ... more
SoftSerialSendOnry.h
00001 #ifndef SOFTSERIAL_SEND_ONRY_H 00002 #define SOFTSERIAL_SEND_ONRY_H 00003 00004 #include "mbed.h" 00005 #include "SoftSerial_Ticker.h" 00006 /** A software serial implementation 00007 * 00008 */ 00009 class SoftSerialSendOnry: public Stream { 00010 00011 public: 00012 /** 00013 * Constructor 00014 * 00015 * @param TX Name of the TX pin, NC for not connected 00016 * @param name Name of the connection 00017 */ 00018 SoftSerialSendOnry(PinName TX, const char* name = NULL); 00019 virtual ~SoftSerialSendOnry(); 00020 00021 /** Set the baud rate of the serial port 00022 * 00023 * @param baudrate The baudrate of the serial port (default = 9600). 00024 */ 00025 void baud(int baudrate); 00026 00027 enum Parity { 00028 None = 0, 00029 Odd, 00030 Even, 00031 Forced1, 00032 Forced0 00033 }; 00034 00035 enum IrqType { 00036 RxIrq = 0, 00037 TxIrq 00038 }; 00039 00040 /** Set the transmission format used by the serial port 00041 * 00042 * @param bits The number of bits in a word (default = 8) 00043 * @param parity The parity used (SerialBase::None, SerialBase::Odd, SerialBase::Even, SerialBase::Forced1, SerialBase::Forced0; default = SerialBase::None) 00044 * @param stop The number of stop bits (default = 1) 00045 */ 00046 void format(int bits=8, Parity parity=SoftSerialSendOnry::None, int stop_bits=1); 00047 00048 /** Determine if there is space available to write a character 00049 * 00050 * @returns 00051 * 1 if there is space to write a character, 00052 * 0 otherwise 00053 */ 00054 int writeable(); 00055 00056 /** Attach a function to call whenever a serial interrupt is generated 00057 * 00058 * @param fptr A pointer to a void function, or 0 to set as none 00059 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00060 */ 00061 void attach(void (*fptr)(void), IrqType type=RxIrq) { 00062 fpointer[type].attach(fptr); 00063 } 00064 00065 /** Attach a member function to call whenever a serial interrupt is generated 00066 * 00067 * @param tptr pointer to the object to call the member function on 00068 * @param mptr pointer to the member function to be called 00069 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00070 */ 00071 template<typename T> 00072 void attach(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00073 fpointer[type].attach(tptr, mptr); 00074 } 00075 00076 /** Generate a break condition on the serial line 00077 */ 00078 void send_break(); 00079 00080 protected: 00081 DigitalOut *tx; 00082 00083 bool tx_en; 00084 int bit_period; 00085 int _bits, _stop_bits, _total_bits; 00086 Parity _parity; 00087 00088 FunctionPointer fpointer[2]; 00089 00090 //tx 00091 void tx_handler(void); 00092 void prepare_tx(int c); 00093 FlexTicker txticker; 00094 int _char; 00095 volatile int tx_bit; 00096 00097 00098 00099 virtual int _getc(); 00100 virtual int _putc(int c); 00101 }; 00102 00103 00104 #endif 00105
Generated on Tue Jul 12 2022 18:13:35 by
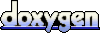