Simple IoT Board用のライブラリです。 ESP8266ライブラリの軽量化 送信のみのソフトシリアルライブラリを含んでいます。
Dependents: SITB_HttpGetSample SITB_IFTTTSample SITB_INA226PRC AmbientExampleSITB ... more
Socket.cpp
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "Socket.h" 00020 #include <cstring> 00021 00022 //Debug is disabled by default 00023 #if 0 00024 //Enable debug 00025 #include <cstdio> 00026 #define DBG(x, ...) std::printf("[Socket : DBG]"x" \t[%s,%d]\r\n", ##__VA_ARGS__,__FILE__,__LINE__); 00027 #define WARN(x, ...) std::printf("[Socket : WARN]"x" \t[%s,%d]\r\n", ##__VA_ARGS__,__FILE__,__LINE__); 00028 #define ERR(x, ...) std::printf("[Socket : ERR]"x" \t[%s,%d]\r\n", ##__VA_ARGS__,__FILE__,__LINE__); 00029 00030 #else 00031 //Disable debug 00032 #define DBG(x, ...) 00033 #define WARN(x, ...) 00034 #define ERR(x, ...) 00035 00036 #endif 00037 00038 Socket::Socket() : _blocking(true), _timeout(1500) { 00039 wifi = ESP8266::getInstance(); 00040 if (wifi == NULL) 00041 ERR("Socket constructor error: no ESP8266 instance available!"); 00042 } 00043 00044 void Socket::set_blocking(bool blocking, unsigned int timeout) { 00045 DBG("set blocking: %d %d", blocking, timeout); 00046 _blocking = blocking; 00047 _timeout = timeout; 00048 } 00049 00050 int Socket::close() { 00051 00052 return (wifi->close()) ? 0 : -1; 00053 } 00054 00055 Socket::~Socket() { 00056 close(); //Don't want to leak 00057 }
Generated on Tue Jul 12 2022 18:13:35 by
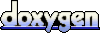