Simple IoT Board用のライブラリです。 ESP8266ライブラリの軽量化 送信のみのソフトシリアルライブラリを含んでいます。
Dependents: SITB_HttpGetSample SITB_IFTTTSample SITB_INA226PRC AmbientExampleSITB ... more
ESP8266.h
00001 /* Copyright (C) 2012 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00005 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00006 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00007 * furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 * 00018 * @section DESCRIPTION 00019 * 00020 * ESP8266 serial wifi module 00021 * 00022 * Datasheet: 00023 * 00024 * http://www.electrodragon.com/w/Wi07c 00025 */ 00026 00027 #ifndef ESP8266_H 00028 #define ESP8266_H 00029 00030 #include "mbed.h" 00031 #include "CBuffer.h" 00032 00033 #define DEFAULT_WAIT_RESP_TIMEOUT 500 00034 #define ESP_TCP_TYPE 1 00035 #define ESP_UDP_TYPE 0 00036 #define ESP_MBUFFE_MAX 256 00037 00038 /** 00039 * The ESP8266 class 00040 */ 00041 class ESP8266 00042 { 00043 00044 public: 00045 /** 00046 * Constructor 00047 * 00048 * @param tx mbed pin to use for tx line of Serial interface 00049 * @param rx mbed pin to use for rx line of Serial interface 00050 * @param reset reset pin of the wifi module () 00051 * @param ssid ssid of the network 00052 * @param phrase WEP, WPA or WPA2 key 00053 * @param baud the baudrate of the serial connection 00054 */ 00055 ESP8266( PinName tx, PinName rx, PinName reset, const char * ssid, const char * phrase, uint32_t baud ); 00056 00057 /** 00058 * Connect the wifi module to the ssid contained in the constructor. 00059 * 00060 * @return true if connected, false otherwise 00061 */ 00062 bool join(); 00063 00064 /** 00065 * Same as Join: connect to the ssid and get DHCP settings 00066 * @return true if successful 00067 */ 00068 bool connect(); 00069 00070 /** 00071 * Check connection to the access point 00072 * @return true if successful 00073 */ 00074 bool is_connected(); 00075 00076 /** 00077 * Disconnect the ESP8266 module from the access point 00078 * 00079 * @return true if successful 00080 */ 00081 bool disconnect(); 00082 00083 /* 00084 * Start up a UDP or TCP Connection 00085 * @param type 0 for UDP, 1 for TCP 00086 * @param ip A string that contains the IP, no quotes 00087 * @param port Numerical port number to connect to 00088 * @param id number between 0-4, if defined it denotes ID to use in multimode (Default to Single connection mode with -1) 00089 * @return true if sucessful, 0 if fail 00090 */ 00091 bool start(bool type, char* ip, int port, int id = -1); 00092 00093 /* 00094 * Legacy Start for UDP only connection in transparent mode 00095 * @param ip A string that contains the IP, no quotes 00096 * @param id number between 0-4 00097 * @param port Numerical port number to connect to 00098 * @param length number of characters in the message being sent 00099 */ 00100 bool startUDP(char* ip, int port, int id, int length); 00101 00102 /* 00103 * Legacy Start for UDP only connection in transparent mode 00104 * @param ip A string that contains the IP, no quotes 00105 * @param id number between 0-4 00106 * @param port Numerical port number to connect to 00107 * @param length number of characters in the message being sent 00108 */ 00109 //bool startUDP(char* ip, int port, int id, int length); 00110 00111 /* 00112 *Starts the ESP chip as a TCP Server 00113 *@param port Numerical port of the server, default is 333 00114 */ 00115 bool startTCPServer(int port = 333); 00116 00117 /** 00118 * Close a connection 00119 * 00120 * @return true if successful 00121 */ 00122 bool close(); 00123 00124 /** 00125 * Return the IP address 00126 * @return IP address as a string 00127 */ 00128 char* getIPAddress(); 00129 00130 /** 00131 * Return the IP address from host name 00132 * @return true on success, false on failure 00133 */ 00134 bool gethostbyname(const char * host, char * ip); 00135 00136 /** 00137 * Reset the wifi module 00138 */ 00139 void reset(); 00140 00141 /** 00142 * Reboot the wifi module 00143 */ 00144 bool reboot(); 00145 00146 /** 00147 * Check if characters are available 00148 * 00149 * @return number of available characters 00150 */ 00151 int readable(); 00152 00153 /** 00154 * Check if characters are available 00155 * 00156 * @return number of available characters 00157 */ 00158 int writeable(); 00159 00160 /** 00161 * Read a character 00162 * 00163 * @return the character read 00164 */ 00165 char getc(); 00166 00167 /** 00168 * Write a character 00169 * 00170 * @param the character which will be written 00171 */ 00172 int putc(char c); 00173 00174 /** 00175 * Flush the buffer 00176 */ 00177 void flush(); 00178 00179 /** 00180 * Send a command to the wifi module. Check if the module is in command mode. If not enter in command mode 00181 * 00182 * @param str string to be sent 00183 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknowledged. (default: "NO") 00184 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00185 * 00186 * @return true if successful 00187 */ 00188 bool sendCommand(const char * cmd, const char * ack = NULL, char * res = NULL, int timeout = DEFAULT_WAIT_RESP_TIMEOUT); 00189 00190 /** 00191 * Send a string to the wifi module by serial port. This function desactivates the user interrupt handler when a character is received to analyze the response from the wifi module. 00192 * Useful to send a command to the module and wait a response. 00193 * 00194 * 00195 * @param str string to be sent 00196 * @param len string length 00197 * @param ACK string which must be acknowledge by the wifi module. If ACK == NULL, no string has to be acknoledged. (default: "NO") 00198 * @param res this field will contain the response from the wifi module, result of a command sent. This field is available only if ACK = "NO" AND res != NULL (default: NULL) 00199 * 00200 * @return true if ACK has been found in the response from the wifi module. False otherwise or if there is no response in 5s. 00201 */ 00202 int send(const char * buf, int len); 00203 00204 static ESP8266 * getInstance() { 00205 return inst; 00206 }; 00207 00208 protected: 00209 int strfind(const char *str,const char *chkstr,int pos=0); 00210 char* substr(const char *str , char *outstr , int pos1 , int pos2 ); 00211 int strcount(const char *str , char countstr ); 00212 00213 00214 RawSerial wifi; 00215 DigitalOut reset_pin; 00216 char phrase[30]; 00217 char ssid[30]; 00218 char ipString[20]; 00219 CircBuffer<char> buf_ESP8266; 00220 00221 static ESP8266 * inst; 00222 00223 void attach_rx(bool null); 00224 void handler_rx(void); 00225 00226 00227 typedef struct STATE { 00228 bool associated; 00229 bool cmdMode; 00230 } State; 00231 00232 State state; 00233 }; 00234 00235 #endif
Generated on Tue Jul 12 2022 18:13:35 by
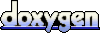