Using std::ostream with TextLCD
Dependencies: ACM1602NI TextLCD
Dependents: TextLCD_ostream_sample
TextLCD_ostream.h
00001 #ifndef TEXTLCD_OSTREAM_H 00002 #define TEXTLCD_OSTREAM_H 00003 00004 #include <ostream> 00005 #include <streambuf> 00006 00007 /** ostream wrapper for TextLCD. 00008 LCDCLASS(TextLCD) need to have putc(),locate(),cls() function. 00009 @code 00010 #include "mbed.h" 00011 #include "TextLCD.h" 00012 #include "TextLCD_ostream.h" 00013 00014 I2C i2c(p28,p27); // SDA, SCL 00015 TextLCD_I2C_N lcd(&i2c, ST7032_SA, TextLCD::LCD16x2, NC, TextLCD::ST7032_3V3); 00016 TextLCD_ostream<TextLCD_I2C_N> lcdstream(&lcd); 00017 00018 int main() { 00019 using namespace std; 00020 lcd.setContrast(32); 00021 lcdstream.cls() << "Hello world" << endl << "LCD ostream"; 00022 } 00023 @endcode 00024 */ 00025 template <class LCDCLASS> 00026 class TextLCD_ostream : public std::ostream { 00027 public: 00028 /// @param p pointer to object of LCD class 00029 TextLCD_ostream (LCDCLASS *p) 00030 : std::ostream(&lcd_buf),lcdp(p),lcd_buf(p) {} 00031 /// access LCD object 00032 LCDCLASS& lcd() {return *lcdp;} 00033 /// set cursor position. 00034 /// same as LCDCLASS::locate(), except return value type 00035 TextLCD_ostream& locate(int column, int row) { 00036 lcdp->locate(column,row); 00037 return *this; 00038 } 00039 /// clear screen 00040 /// same as LCDCLASS::cls(), except return value type 00041 TextLCD_ostream& cls(){ 00042 lcdp->cls(); 00043 return *this; 00044 } 00045 private: 00046 LCDCLASS* const lcdp; 00047 class TextLCD_streambuf : public std::streambuf { 00048 public: 00049 TextLCD_streambuf(LCDCLASS *p) : lcdp(p) {} 00050 virtual int_type overflow( int_type c = EOF ) { 00051 if( c != EOF ) lcdp->putc(c); 00052 return c; 00053 } 00054 00055 private: 00056 LCDCLASS *lcdp; 00057 } lcd_buf; 00058 }; 00059 00060 #ifndef NOTextLCD_h 00061 //for compatibility to previous version 00062 #include "TextLCD.h" 00063 // specialized version 00064 typedef TextLCD_ostream<TextLCD_Base> lcd_ostream; 00065 #endif 00066 00067 #endif
Generated on Fri Jul 15 2022 01:37:17 by
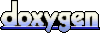