
This program makes the WAV file of the sinewave in a local file. The sampling frequency is 8KHz. In other words, it is the quality of the telephone. The quantization precision is 16 bits. You can change the frequency of the sinewave, the amplitude by changing a parameter in the top of the program. You can change the file name, too. I suggest that you make the plain name later. You can listen the wav file which You made in Windows Mediaplayer. See: http://blogs.yahoo.co.jp/jf1vrr_station/19862738.html (Japanese)
main.cpp
00001 /*This program makes the WAV file of the sinewave in a local file. 00002 The sampling frequency is 8KHz. In other words, it is the quality of the 00003 telephone. The quantization precision is 16 bits. You can change the 00004 frequency of the sinewave, the amplitude by changing a parameter in the 00005 top of the program. You can change the file name, too. I suggest that 00006 you make the plain name later. You can listen the wav file which You made 00007 in Windows Mediaplayer. 00008 */ 00009 00010 /*This program is made in reference to "Sound Programming in C Language" by Aoki Naofumi"*/ 00011 00012 #define FREQ 440 //Frequency (Hz) 00013 #define AMP 0.5 //Amplitude 0 - 1 00014 #define FILE_NAME "/local/440_05.wav" //Data file name max 8char.wav fffff_aa.wav 00015 00016 #include "mbed.h" 00017 #include "TextLCD.h" 00018 00019 TextLCD lcd(p24, p26, p27, p28, p29, p30); 00020 00021 LocalFileSystem local("local"); 00022 DigitalOut led_1(LED1); 00023 DigitalOut led_2(LED2); 00024 DigitalOut led_3(LED3); 00025 DigitalOut led_4(LED4); 00026 00027 int main(void) 00028 { 00029 typedef struct 00030 { 00031 int fs; 00032 int bits; 00033 int length; 00034 double *s; 00035 } MONO_PCM; 00036 00037 FILE *fp; 00038 MONO_PCM pcm; 00039 int i, j; 00040 double s; 00041 short data; 00042 double A, f0; 00043 00044 char riff_chunk_ID[4]; 00045 long riff_chunk_size; 00046 char riff_form_type[4]; 00047 char fmt_chunk_ID[4]; 00048 long fmt_chunk_size; 00049 short fmt_wave_format_type; 00050 short fmt_channel; 00051 long fmt_samples_per_sec; 00052 long fmt_bytes_per_sec; 00053 short fmt_block_size; 00054 short fmt_bits_per_sample; 00055 char data_chunk_ID[4]; 00056 long data_chunk_size; 00057 00058 lcd.cls(); 00059 lcd.locate(0,0); 00060 lcd.printf("Audio wave\n"); 00061 00062 int block_length = 1000; 00063 00064 pcm.fs = 8000; 00065 pcm.bits = 16; 00066 pcm.length = 40000; //about 5sec 00067 pcm.s = (double*) calloc(block_length, sizeof(double)); 00068 00069 A = AMP; 00070 f0 = FREQ; 00071 00072 if ( NULL == (fp = fopen(FILE_NAME, "wb" )) ) 00073 error( "" ); 00074 00075 led_1 = 1; 00076 riff_chunk_ID[0] = 'R'; 00077 riff_chunk_ID[1] = 'I'; 00078 riff_chunk_ID[2] = 'F'; 00079 riff_chunk_ID[3] = 'F'; 00080 riff_chunk_size = 36 + pcm.length * 2; 00081 riff_form_type[0] = 'W'; 00082 riff_form_type[1] = 'A'; 00083 riff_form_type[2] = 'V'; 00084 riff_form_type[3] = 'E'; 00085 00086 fmt_chunk_ID[0] = 'f'; 00087 fmt_chunk_ID[1] = 'm'; 00088 fmt_chunk_ID[2] = 't'; 00089 fmt_chunk_ID[3] = ' '; 00090 fmt_chunk_size = 16; 00091 fmt_wave_format_type = 1; 00092 fmt_channel = 1; 00093 fmt_samples_per_sec = pcm.fs; 00094 fmt_bytes_per_sec = pcm.fs * pcm.bits / 8; 00095 fmt_block_size = pcm.bits / 8; 00096 fmt_bits_per_sample = pcm.bits; 00097 00098 data_chunk_ID[0] = 'd'; 00099 data_chunk_ID[1] = 'a'; 00100 data_chunk_ID[2] = 't'; 00101 data_chunk_ID[3] = 'a'; 00102 data_chunk_size = pcm.length * 2; 00103 00104 fwrite(riff_chunk_ID, 1, 4, fp); 00105 fwrite(&riff_chunk_size, 4, 1, fp); 00106 fwrite(riff_form_type, 1, 4, fp); 00107 fwrite(fmt_chunk_ID, 1, 4, fp); 00108 fwrite(&fmt_chunk_size, 4, 1, fp); 00109 fwrite(&fmt_wave_format_type, 2, 1, fp); 00110 fwrite(&fmt_channel, 2, 1, fp); 00111 fwrite(&fmt_samples_per_sec, 4, 1, fp); 00112 fwrite(&fmt_bytes_per_sec, 4, 1, fp); 00113 fwrite(&fmt_block_size, 2, 1, fp); 00114 fwrite(&fmt_bits_per_sample, 2, 1, fp); 00115 fwrite(data_chunk_ID, 1, 4, fp); 00116 fwrite(&data_chunk_size, 4, 1, fp); 00117 wait(0.5); 00118 led_1 = 0; 00119 00120 led_2 = 1; 00121 for(i = 0; i < pcm.length/block_length; i++) 00122 { 00123 00124 for (j = 0; j < block_length; j++) 00125 { 00126 pcm.s[j] = A * sin(2.0 * 3.141592 * f0 * (i * block_length + j) / pcm.fs); 00127 s = (pcm.s[j] + 1.0) / 2.0 * 65536.0; 00128 00129 if (s > 65535.0) s = 65535.0; 00130 else if (s < 0.0) s = 0.0; 00131 00132 data = (short)(s + 0.5) - 32768; 00133 fwrite(&data, 2, 1, fp); 00134 } 00135 } 00136 led_2 = 0; 00137 fclose(fp); 00138 free(pcm.s); 00139 00140 lcd.locate(0,1); 00141 lcd.printf("Done!\n"); 00142 00143 return 0; 00144 }
Generated on Thu Jul 14 2022 21:04:23 by
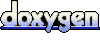