Dual DC Motor Drive using PWM for RenBuggy; there are two inputs to feed a hall sensor for distance / speed feedback.
DCMotorDrive.h
00001 /******************************************************************************* 00002 * RenBED DC Motor Drive for RenBuggy * 00003 * Copyright (c) 2014 Jon Fuge * 00004 * * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy * 00006 * of this software and associated documentation files (the "Software"), to deal* 00007 * in the Software without restriction, including without limitation the rights * 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell * 00009 * copies of the Software, and to permit persons to whom the Software is * 00010 * furnished to do so, subject to the following conditions: * 00011 * * 00012 * The above copyright notice and this permission notice shall be included in * 00013 * all copies or substantial portions of the Software. * 00014 * * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR * 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, * 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE * 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER * 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,* 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN * 00021 * THE SOFTWARE. * 00022 * * 00023 * DCMotorDrive.h * 00024 * * 00025 * V1.0 06/01/2014 First issue of code Jon Fuge * 00026 *******************************************************************************/ 00027 00028 #ifndef _DCMOTORDRIVE_H 00029 #define _DCMOTORDRIVE_H 00030 00031 #include "mbed.h" 00032 00033 #define PWM_PERIOD 20 00034 #define MOTOR_STALL_TIME 2 00035 00036 class DCMotorDrive 00037 { 00038 public: 00039 DCMotorDrive(PinName MotorOut, PinName BrakeOut, PinName SensorIn); 00040 void SetMotorPwm(int PwmValue); 00041 void SetMotorPwmAndRevolutions(int PwmValue, int MaxRevolutions); 00042 int GetAveragePulseTime(void); 00043 int GetLastPulseTime(void); 00044 int GetRevolutionsLeft(void); 00045 void ResetOdometer(void); 00046 int ReadOdometer(void); 00047 int ReadCaptureTime(void); 00048 00049 private: 00050 Ticker timeout; 00051 Timer PulseClock; 00052 volatile int ClockPointer; 00053 volatile int LastPulseTime; 00054 volatile int RotationCounter; 00055 volatile int RevolutionLimit; 00056 00057 PwmOut _MotorPin; 00058 DigitalOut _BrakePin; 00059 InterruptIn _SensorIn; 00060 00061 void Counter(void); 00062 void Stall(void); 00063 }; 00064 00065 #endif // _DCMOTORDRIVE_H
Generated on Sun Jul 24 2022 16:27:15 by
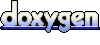