
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
timer.cpp
00001 /** 00002 * @file timer.c 00003 * @brief mbed-os implementation of the timer interface needed for AWS. 00004 */ 00005 #ifdef __cplusplus 00006 extern "C" { 00007 #endif 00008 00009 #include <stddef.h> 00010 #include "timer_interface.h" 00011 00012 TimerExt::TimerExt() : mbed::Timer(){ 00013 00014 } 00015 00016 bool has_timer_expired(TimerAWS* timer) { 00017 return (timer->read_ms() > timer->timeout_ms); 00018 } 00019 00020 void countdown_ms(TimerAWS *timer, uint32_t timeout) { 00021 timer->timeout_ms = timeout; 00022 timer->reset(); 00023 timer->start(); 00024 } 00025 00026 void countdown_sec(TimerAWS *timer, uint32_t timeout) { 00027 timer->timeout_ms = (timeout * 1000); 00028 timer->reset(); 00029 timer->start(); 00030 } 00031 00032 uint32_t left_ms(TimerAWS* timer) { 00033 if (timer->read_ms() < timer->timeout_ms) 00034 return (timer->timeout_ms - timer->read_ms()); 00035 return 0; 00036 } 00037 00038 void init_timer(TimerAWS * timer){ 00039 timer->stop(); 00040 timer->reset(); 00041 timer->timeout_ms = 0; 00042 } 00043 #ifdef __cplusplus 00044 } 00045 #endif
Generated on Tue Jul 12 2022 11:16:38 by
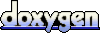