
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
net_socket.cpp
00001 #include "net_sockets.h" 00002 #include "network_interface.h" 00003 //#include "EthernetInterface.h" 00004 #include "TCPSocket.h" 00005 #include "ESP8266Interface.h" 00006 00007 TCPSocket * tcp_socket; 00008 00009 void mbedtls_net_init(mbedtls_net_context *ctx) { 00010 //EthernetInterface eth_iface; 00011 ESP8266Interface eth_iface(D1, D0); 00012 //eth_iface.connect(); 00013 eth_iface.connect(MBED_CONF_APP_WIFI_SSID, MBED_CONF_APP_WIFI_PASSWORD, NSAPI_SECURITY_WPA_WPA2); 00014 tcp_socket = new TCPSocket(ð_iface); 00015 return; 00016 } 00017 00018 int mbedtls_net_connect(mbedtls_net_context *ctx, const char *host, 00019 const char *port, int proto) { 00020 return tcp_socket->connect(host, atoi(port)); 00021 } 00022 00023 int mbedtls_net_set_block(mbedtls_net_context *ctx) { 00024 tcp_socket->set_blocking(false); 00025 return 0; 00026 } 00027 00028 int mbedtls_net_recv(void *ctx, unsigned char *buf, size_t len) { 00029 return tcp_socket->recv(buf, len); 00030 } 00031 00032 int mbedtls_net_send(void *ctx, const unsigned char *buf, size_t len) { 00033 int size = -1; 00034 00035 size = tcp_socket->send(buf, len); 00036 00037 if(NSAPI_ERROR_WOULD_BLOCK == size){ 00038 return len; 00039 }else if(size < 0){ 00040 return -1; 00041 }else{ 00042 return size; 00043 } 00044 } 00045 00046 int mbedtls_net_recv_timeout(void *ctx, unsigned char *buf, size_t len, 00047 uint32_t timeout) { 00048 00049 int recv = -1; 00050 tcp_socket->set_timeout(timeout); 00051 recv = tcp_socket->recv(buf, len); 00052 00053 if(NSAPI_ERROR_WOULD_BLOCK == recv || 00054 recv == 0){ 00055 return 0; 00056 }else if(recv < 0){ 00057 return -1; 00058 }else{ 00059 return recv; 00060 } 00061 } 00062 00063 void mbedtls_net_free(mbedtls_net_context *ctx) { 00064 return; 00065 }
Generated on Tue Jul 12 2022 11:16:38 by
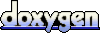