
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
aws_iot_shadow_actions.cpp
00001 /* 00002 * Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 /** 00017 * @file aws_iot_shadow_actions.c 00018 * @brief Shadow client Action API definitions 00019 */ 00020 00021 #ifdef __cplusplus 00022 extern "C" { 00023 #endif 00024 00025 #include "aws_iot_shadow_actions.h" 00026 00027 #include "aws_iot_log.h" 00028 #include "aws_iot_shadow_json.h" 00029 #include "aws_iot_shadow_records.h" 00030 #include "aws_iot_config.h" 00031 00032 IoT_Error_t aws_iot_shadow_internal_action(const char *pThingName, ShadowActions_t action, 00033 const char *pJsonDocumentToBeSent, fpActionCallback_t callback, 00034 void *pCallbackContext, uint32_t timeout_seconds, bool isSticky) { 00035 IoT_Error_t ret_val = IOT_SUCCESS; 00036 bool isClientTokenPresent = false; 00037 bool isAckWaitListFree = false; 00038 uint8_t indexAckWaitList; 00039 char extractedClientToken[MAX_SIZE_CLIENT_ID_WITH_SEQUENCE]; 00040 00041 FUNC_ENTRY; 00042 00043 if(NULL == pThingName || NULL == pJsonDocumentToBeSent) { 00044 FUNC_EXIT_RC(NULL_VALUE_ERROR); 00045 } 00046 00047 isClientTokenPresent = extractClientToken(pJsonDocumentToBeSent, extractedClientToken); 00048 00049 if(isClientTokenPresent && (NULL != callback)) { 00050 if(getNextFreeIndexOfAckWaitList(&indexAckWaitList)) { 00051 isAckWaitListFree = true; 00052 } 00053 00054 if(isAckWaitListFree) { 00055 if(!isSubscriptionPresent(pThingName, action)) { 00056 ret_val = subscribeToShadowActionAcks(pThingName, action, isSticky); 00057 } else { 00058 incrementSubscriptionCnt(pThingName, action, isSticky); 00059 } 00060 } 00061 else { 00062 ret_val = IOT_FAILURE; 00063 } 00064 } 00065 00066 if(IOT_SUCCESS == ret_val) { 00067 ret_val = publishToShadowAction(pThingName, action, pJsonDocumentToBeSent); 00068 } 00069 00070 if(isClientTokenPresent && (NULL != callback) && (IOT_SUCCESS == ret_val) && isAckWaitListFree) { 00071 addToAckWaitList(indexAckWaitList, pThingName, action, extractedClientToken, callback, pCallbackContext, 00072 timeout_seconds); 00073 } 00074 00075 FUNC_EXIT_RC(ret_val); 00076 } 00077 00078 #ifdef __cplusplus 00079 } 00080 #endif
Generated on Tue Jul 12 2022 11:16:37 by
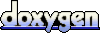