
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
aws_iot_mqtt_client_common_internal.h
Go to the documentation of this file.
00001 /* 00002 * Copyright 2015-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 // Based on Eclipse Paho. 00017 /******************************************************************************* 00018 * Copyright (c) 2014 IBM Corp. 00019 * 00020 * All rights reserved. This program and the accompanying materials 00021 * are made available under the terms of the Eclipse Public License v1.0 00022 * and Eclipse Distribution License v1.0 which accompany this distribution. 00023 * 00024 * The Eclipse Public License is available at 00025 * http://www.eclipse.org/legal/epl-v10.html 00026 * and the Eclipse Distribution License is available at 00027 * http://www.eclipse.org/org/documents/edl-v10.php. 00028 * 00029 * Contributors: 00030 * Ian Craggs - initial API and implementation and/or initial documentation 00031 * Xiang Rong - 442039 Add makefile to Embedded C client 00032 *******************************************************************************/ 00033 00034 /** 00035 * @file aws_iot_mqtt_client_common_internal.h 00036 * @brief Internal MQTT functions not exposed to application 00037 */ 00038 00039 #ifndef AWS_IOT_SDK_SRC_IOT_COMMON_INTERNAL_H 00040 #define AWS_IOT_SDK_SRC_IOT_COMMON_INTERNAL_H 00041 00042 #ifdef __cplusplus 00043 extern "C" { 00044 #endif 00045 00046 #include <stdint.h> 00047 #include <stddef.h> 00048 #include <string.h> 00049 00050 #include "aws_iot_log.h" 00051 #include "aws_iot_mqtt_client_interface.h" 00052 00053 /* Enum order should match the packet ids array defined in MQTTFormat.c */ 00054 typedef enum msgTypes { 00055 UNKNOWN = -1, 00056 CONNECT = 1, 00057 CONNACK = 2, 00058 PUBLISH = 3, 00059 PUBACK = 4, 00060 PUBREC = 5, 00061 PUBREL = 6, 00062 PUBCOMP = 7, 00063 SUBSCRIBE = 8, 00064 SUBACK = 9, 00065 UNSUBSCRIBE = 10, 00066 UNSUBACK = 11, 00067 PINGREQ = 12, 00068 PINGRESP = 13, 00069 DISCONNECT = 14 00070 } MessageTypes; 00071 00072 /** 00073 * Bitfields for the MQTT header byte. 00074 */ 00075 typedef union { 00076 unsigned char byte; /**< the whole byte */ 00077 #if defined(REVERSED) 00078 struct { 00079 unsigned int type : 4; /**< message type nibble */ 00080 unsigned int dup : 1; /**< DUP flag bit */ 00081 unsigned int qos : 2; /**< QoS value, 0, 1 or 2 */ 00082 unsigned int retain : 1; /**< retained flag bit */ 00083 } bits; 00084 #else 00085 struct { 00086 unsigned int retain : 1; /**< retained flag bit */ 00087 unsigned int qos : 2; /**< QoS value, 0, 1 or 2 */ 00088 unsigned int dup : 1; /**< DUP flag bit */ 00089 unsigned int type : 4; /**< message type nibble */ 00090 } bits; 00091 #endif 00092 } MQTTHeader; 00093 00094 IoT_Error_t aws_iot_mqtt_internal_init_header(MQTTHeader *pHeader, MessageTypes message_type, 00095 QoS qos, uint8_t dup, uint8_t retained); 00096 00097 IoT_Error_t aws_iot_mqtt_internal_serialize_ack(unsigned char *pTxBuf, size_t txBufLen, 00098 MessageTypes msgType, uint8_t dup, uint16_t packetId, 00099 uint32_t *pSerializedLen); 00100 IoT_Error_t aws_iot_mqtt_internal_deserialize_ack(unsigned char *, unsigned char *, 00101 uint16_t *, unsigned char *, size_t); 00102 00103 uint32_t aws_iot_mqtt_internal_get_final_packet_length_from_remaining_length(uint32_t rem_len); 00104 00105 size_t aws_iot_mqtt_internal_write_len_to_buffer(unsigned char *buf, uint32_t length); 00106 IoT_Error_t aws_iot_mqtt_internal_decode_remaining_length_from_buffer(unsigned char *buf, uint32_t *decodedLen, 00107 uint32_t *readBytesLen); 00108 00109 uint16_t aws_iot_mqtt_internal_read_uint16_t(unsigned char **pptr); 00110 void aws_iot_mqtt_internal_write_uint_16(unsigned char **pptr, uint16_t anInt); 00111 00112 unsigned char aws_iot_mqtt_internal_read_char(unsigned char **pptr); 00113 void aws_iot_mqtt_internal_write_char(unsigned char **pptr, unsigned char c); 00114 void aws_iot_mqtt_internal_write_utf8_string(unsigned char **pptr, const char *string, uint16_t stringLen); 00115 00116 IoT_Error_t aws_iot_mqtt_internal_send_packet(AWS_IoT_Client *pClient, size_t length, TimerAWS *pTimer); 00117 IoT_Error_t aws_iot_mqtt_internal_cycle_read(AWS_IoT_Client *pClient, TimerAWS *pTimer, uint8_t *pPacketType); 00118 IoT_Error_t aws_iot_mqtt_internal_wait_for_read(AWS_IoT_Client *pClient, uint8_t packetType, TimerAWS *pTimer); 00119 IoT_Error_t aws_iot_mqtt_internal_serialize_zero(unsigned char *pTxBuf, size_t txBufLen, 00120 MessageTypes packetType, size_t *pSerializedLength); 00121 IoT_Error_t aws_iot_mqtt_internal_deserialize_publish(uint8_t *dup, QoS *qos, 00122 uint8_t *retained, uint16_t *pPacketId, 00123 char **pTopicName, uint16_t *topicNameLen, 00124 unsigned char **payload, size_t *payloadLen, 00125 unsigned char *pRxBuf, size_t rxBufLen); 00126 00127 IoT_Error_t aws_iot_mqtt_set_client_state(AWS_IoT_Client *pClient, ClientState expectedCurrentState, 00128 ClientState newState); 00129 00130 #ifdef _ENABLE_THREAD_SUPPORT_ 00131 00132 IoT_Error_t aws_iot_mqtt_client_lock_mutex(AWS_IoT_Client *pClient, IoT_Mutex_t *pMutex); 00133 00134 IoT_Error_t aws_iot_mqtt_client_unlock_mutex(AWS_IoT_Client *pClient, IoT_Mutex_t *pMutex); 00135 00136 #endif 00137 00138 #ifdef __cplusplus 00139 } 00140 #endif 00141 00142 #endif /* AWS_IOT_SDK_SRC_IOT_COMMON_INTERNAL_H */
Generated on Tue Jul 12 2022 11:16:37 by
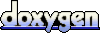