
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
aws_iot_json_utils.h
00001 /* 00002 * Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 /** 00017 * @file aws_json_utils.h 00018 * @brief Utilities for manipulating JSON 00019 * 00020 * json_utils provides JSON parsing utilities for use with the IoT SDK. 00021 * Underlying JSON parsing relies on the Jasmine JSON parser. 00022 * 00023 */ 00024 00025 #ifndef AWS_IOT_SDK_SRC_JSON_UTILS_H_ 00026 #define AWS_IOT_SDK_SRC_JSON_UTILS_H_ 00027 00028 #ifdef __cplusplus 00029 extern "C" { 00030 #endif 00031 00032 #include <stdbool.h> 00033 #include <stdint.h> 00034 00035 #include "aws_iot_error.h" 00036 #include "jsmn.h" 00037 00038 // utility functions 00039 /** 00040 * @brief JSON Equality Check 00041 * 00042 * Given a token pointing to a particular JSON node and an 00043 * input string, check to see if the key is equal to the string. 00044 * 00045 * @param json json string 00046 * @param tok json token - pointer to key to test for equality 00047 * @param s input string for key to test equality 00048 * 00049 * @return 0 if equal, 1 otherwise 00050 */ 00051 int8_t jsoneq(const char *json, jsmntok_t *tok, const char *s); 00052 00053 /** 00054 * @brief Parse a signed 32-bit integer value from a JSON node. 00055 * 00056 * Given a JSON node parse the integer value from the value. 00057 * 00058 * @param jsonString json string 00059 * @param tok json token - pointer to JSON node 00060 * @param i address of int32_t to be updated 00061 * 00062 * @return IOT_SUCCESS - success 00063 * @return JSON_PARSE_ERROR - error parsing value 00064 */ 00065 IoT_Error_t parseInteger32Value(int32_t *i, const char *jsonString, jsmntok_t *token); 00066 00067 /** 00068 * @brief Parse a signed 16-bit integer value from a JSON node. 00069 * 00070 * Given a JSON node parse the integer value from the value. 00071 * 00072 * @param jsonString json string 00073 * @param tok json token - pointer to JSON node 00074 * @param i address of int16_t to be updated 00075 * 00076 * @return IOT_SUCCESS - success 00077 * @return JSON_PARSE_ERROR - error parsing value 00078 */ 00079 IoT_Error_t parseInteger16Value(int16_t *i, const char *jsonString, jsmntok_t *token); 00080 00081 /** 00082 * @brief Parse a signed 8-bit integer value from a JSON node. 00083 * 00084 * Given a JSON node parse the integer value from the value. 00085 * 00086 * @param jsonString json string 00087 * @param tok json token - pointer to JSON node 00088 * @param i address of int8_t to be updated 00089 * 00090 * @return IOT_SUCCESS - success 00091 * @return JSON_PARSE_ERROR - error parsing value 00092 */ 00093 IoT_Error_t parseInteger8Value(int8_t *i, const char *jsonString, jsmntok_t *token); 00094 00095 /** 00096 * @brief Parse an unsigned 32-bit integer value from a JSON node. 00097 * 00098 * Given a JSON node parse the integer value from the value. 00099 * 00100 * @param jsonString json string 00101 * @param tok json token - pointer to JSON node 00102 * @param i address of uint32_t to be updated 00103 * 00104 * @return IOT_SUCCESS - success 00105 * @return JSON_PARSE_ERROR - error parsing value 00106 */ 00107 IoT_Error_t parseUnsignedInteger32Value(uint32_t *i, const char *jsonString, jsmntok_t *token); 00108 00109 /** 00110 * @brief Parse an unsigned 16-bit integer value from a JSON node. 00111 * 00112 * Given a JSON node parse the integer value from the value. 00113 * 00114 * @param jsonString json string 00115 * @param tok json token - pointer to JSON node 00116 * @param i address of uint16_t to be updated 00117 * 00118 * @return IOT_SUCCESS - success 00119 * @return JSON_PARSE_ERROR - error parsing value 00120 */ 00121 IoT_Error_t parseUnsignedInteger16Value(uint16_t *i, const char *jsonString, jsmntok_t *token); 00122 00123 /** 00124 * @brief Parse an unsigned 8-bit integer value from a JSON node. 00125 * 00126 * Given a JSON node parse the integer value from the value. 00127 * 00128 * @param jsonString json string 00129 * @param tok json token - pointer to JSON node 00130 * @param i address of uint8_t to be updated 00131 * 00132 * @return IOT_SUCCESS - success 00133 * @return JSON_PARSE_ERROR - error parsing value 00134 */ 00135 IoT_Error_t parseUnsignedInteger8Value(uint8_t *i, const char *jsonString, jsmntok_t *token); 00136 00137 /** 00138 * @brief Parse a float value from a JSON node. 00139 * 00140 * Given a JSON node parse the float value from the value. 00141 * 00142 * @param jsonString json string 00143 * @param tok json token - pointer to JSON node 00144 * @param f address of float to be updated 00145 * 00146 * @return IOT_SUCCESS - success 00147 * @return JSON_PARSE_ERROR - error parsing value 00148 */ 00149 IoT_Error_t parseFloatValue(float *f, const char *jsonString, jsmntok_t *token); 00150 00151 /** 00152 * @brief Parse a double value from a JSON node. 00153 * 00154 * Given a JSON node parse the double value from the value. 00155 * 00156 * @param jsonString json string 00157 * @param tok json token - pointer to JSON node 00158 * @param d address of double to be updated 00159 * 00160 * @return IOT_SUCCESS - success 00161 * @return JSON_PARSE_ERROR - error parsing value 00162 */ 00163 IoT_Error_t parseDoubleValue(double *d, const char *jsonString, jsmntok_t *token); 00164 00165 /** 00166 * @brief Parse a boolean value from a JSON node. 00167 * 00168 * Given a JSON node parse the boolean value from the value. 00169 * 00170 * @param jsonString json string 00171 * @param tok json token - pointer to JSON node 00172 * @param b address of boolean to be updated 00173 * 00174 * @return IOT_SUCCESS - success 00175 * @return JSON_PARSE_ERROR - error parsing value 00176 */ 00177 IoT_Error_t parseBooleanValue(bool *b, const char *jsonString, jsmntok_t *token); 00178 00179 /** 00180 * @brief Parse a string value from a JSON node. 00181 * 00182 * Given a JSON node parse the string value from the value. 00183 * 00184 * @param jsonString json string 00185 * @param tok json token - pointer to JSON node 00186 * @param s address of string to be updated 00187 * 00188 * @return IOT_SUCCESS - success 00189 * @return JSON_PARSE_ERROR - error parsing value 00190 */ 00191 IoT_Error_t parseStringValue(char *buf, const char *jsonString, jsmntok_t *token); 00192 00193 #ifdef __cplusplus 00194 } 00195 #endif 00196 00197 #endif /* AWS_IOT_SDK_SRC_JSON_UTILS_H_ */
Generated on Tue Jul 12 2022 11:16:36 by
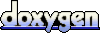