
this is fork and i will modify for STM32
Fork of AWS-test by
Embed:
(wiki syntax)
Show/hide line numbers
aws_iot_error.h
Go to the documentation of this file.
00001 /* 00002 * Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 /** 00017 * @file aws_iot_error.h 00018 * @brief Definition of error types for the SDK. 00019 */ 00020 00021 #ifndef AWS_IOT_SDK_SRC_IOT_ERROR_H_ 00022 #define AWS_IOT_SDK_SRC_IOT_ERROR_H_ 00023 00024 #ifdef __cplusplus 00025 extern "C" { 00026 #endif 00027 00028 /* Used to avoid warnings in case of unused parameters in function pointers */ 00029 #define IOT_UNUSED(x) (void)(x) 00030 00031 /*! \public 00032 * @brief IoT Error enum 00033 * 00034 * Enumeration of return values from the IoT_* functions within the SDK. 00035 * Values less than -1 are specific error codes 00036 * Value of -1 is a generic failure response 00037 * Value of 0 is a generic success response 00038 * Values greater than 0 are specific non-error return codes 00039 */ 00040 typedef enum { 00041 /** Returned when the Network physical layer is connected */ 00042 NETWORK_PHYSICAL_LAYER_CONNECTED = 6, 00043 /** Returned when the Network is manually disconnected */ 00044 NETWORK_MANUALLY_DISCONNECTED = 5, 00045 /** Returned when the Network is disconnected and the reconnect attempt is in progress */ 00046 NETWORK_ATTEMPTING_RECONNECT = 4, 00047 /** Return value of yield function to indicate auto-reconnect was successful */ 00048 NETWORK_RECONNECTED = 3, 00049 /** Returned when a read attempt is made on the TLS buffer and it is empty */ 00050 MQTT_NOTHING_TO_READ = 2, 00051 /** Returned when a connection request is successful and packet response is connection accepted */ 00052 MQTT_CONNACK_CONNECTION_ACCEPTED = 1, 00053 /** Success return value - no error occurred */ 00054 IOT_SUCCESS = 0, 00055 /** A generic error. Not enough information for a specific error code */ 00056 IOT_FAILURE = -1, 00057 /** A required parameter was passed as null */ 00058 NULL_VALUE_ERROR = -2, 00059 /** The TCP socket could not be established */ 00060 TCP_CONNECTION_ERROR = -3, 00061 /** The TLS handshake failed */ 00062 SSL_CONNECTION_ERROR = -4, 00063 /** Error associated with setting up the parameters of a Socket */ 00064 TCP_SETUP_ERROR = -5, 00065 /** A timeout occurred while waiting for the TLS handshake to complete. */ 00066 NETWORK_SSL_CONNECT_TIMEOUT_ERROR = -6, 00067 /** A Generic write error based on the platform used */ 00068 NETWORK_SSL_WRITE_ERROR = -7, 00069 /** SSL initialization error at the TLS layer */ 00070 NETWORK_SSL_INIT_ERROR = -8, 00071 /** An error occurred when loading the certificates. The certificates could not be located or are incorrectly formatted. */ 00072 NETWORK_SSL_CERT_ERROR = -9, 00073 /** SSL Write times out */ 00074 NETWORK_SSL_WRITE_TIMEOUT_ERROR = -10, 00075 /** SSL Read times out */ 00076 NETWORK_SSL_READ_TIMEOUT_ERROR = -11, 00077 /** A Generic error based on the platform used */ 00078 NETWORK_SSL_READ_ERROR = -12, 00079 /** Returned when the Network is disconnected and reconnect is either disabled or physical layer is disconnected */ 00080 NETWORK_DISCONNECTED_ERROR = -13, 00081 /** Returned when the Network is disconnected and the reconnect attempt has timed out */ 00082 NETWORK_RECONNECT_TIMED_OUT_ERROR = -14, 00083 /** Returned when the Network is already connected and a connection attempt is made */ 00084 NETWORK_ALREADY_CONNECTED_ERROR = -15, 00085 /** Network layer Error Codes */ 00086 /** Network layer Random number generator seeding failed */ 00087 NETWORK_MBEDTLS_ERR_CTR_DRBG_ENTROPY_SOURCE_FAILED = -16, 00088 /** A generic error code for Network layer errors */ 00089 NETWORK_SSL_UNKNOWN_ERROR = -17, 00090 /** Returned when the physical layer is disconnected */ 00091 NETWORK_PHYSICAL_LAYER_DISCONNECTED = -18, 00092 /** Returned when the root certificate is invalid */ 00093 NETWORK_X509_ROOT_CRT_PARSE_ERROR = -19, 00094 /** Returned when the device certificate is invalid */ 00095 NETWORK_X509_DEVICE_CRT_PARSE_ERROR = -20, 00096 /** Returned when the private key failed to parse */ 00097 NETWORK_PK_PRIVATE_KEY_PARSE_ERROR = -21, 00098 /** Returned when the network layer failed to open a socket */ 00099 NETWORK_ERR_NET_SOCKET_FAILED = -22, 00100 /** Returned when the server is unknown */ 00101 NETWORK_ERR_NET_UNKNOWN_HOST = -23, 00102 /** Returned when connect request failed */ 00103 NETWORK_ERR_NET_CONNECT_FAILED = -24, 00104 /** Returned when there is nothing to read in the TLS read buffer */ 00105 NETWORK_SSL_NOTHING_TO_READ = -25, 00106 /** A connection could not be established. */ 00107 MQTT_CONNECTION_ERROR = -26, 00108 /** A timeout occurred while waiting for the TLS handshake to complete */ 00109 MQTT_CONNECT_TIMEOUT_ERROR = -27, 00110 /** A timeout occurred while waiting for the TLS request complete */ 00111 MQTT_REQUEST_TIMEOUT_ERROR = -28, 00112 /** The current client state does not match the expected value */ 00113 MQTT_UNEXPECTED_CLIENT_STATE_ERROR = -29, 00114 /** The client state is not idle when request is being made */ 00115 MQTT_CLIENT_NOT_IDLE_ERROR = -30, 00116 /** The MQTT RX buffer received corrupt or unexpected message */ 00117 MQTT_RX_MESSAGE_PACKET_TYPE_INVALID_ERROR = -31, 00118 /** The MQTT RX buffer received a bigger message. The message will be dropped */ 00119 MQTT_RX_BUFFER_TOO_SHORT_ERROR = -32, 00120 /** The MQTT TX buffer is too short for the outgoing message. Request will fail */ 00121 MQTT_TX_BUFFER_TOO_SHORT_ERROR = -33, 00122 /** The client is subscribed to the maximum possible number of subscriptions */ 00123 MQTT_MAX_SUBSCRIPTIONS_REACHED_ERROR = -34, 00124 /** Failed to decode the remaining packet length on incoming packet */ 00125 MQTT_DECODE_REMAINING_LENGTH_ERROR = -35, 00126 /** Connect request failed with the server returning an unknown error */ 00127 MQTT_CONNACK_UNKNOWN_ERROR = -36, 00128 /** Connect request failed with the server returning an unacceptable protocol version error */ 00129 MQTT_CONNACK_UNACCEPTABLE_PROTOCOL_VERSION_ERROR = -37, 00130 /** Connect request failed with the server returning an identifier rejected error */ 00131 MQTT_CONNACK_IDENTIFIER_REJECTED_ERROR = -38, 00132 /** Connect request failed with the server returning an unavailable error */ 00133 MQTT_CONNACK_SERVER_UNAVAILABLE_ERROR = -39, 00134 /** Connect request failed with the server returning a bad userdata error */ 00135 MQTT_CONNACK_BAD_USERDATA_ERROR = -40, 00136 /** Connect request failed with the server failing to authenticate the request */ 00137 MQTT_CONNACK_NOT_AUTHORIZED_ERROR = -41, 00138 /** An error occurred while parsing the JSON string. Usually malformed JSON. */ 00139 JSON_PARSE_ERROR = -42, 00140 /** Shadow: The response Ack table is currently full waiting for previously published updates */ 00141 SHADOW_WAIT_FOR_PUBLISH = -43, 00142 /** Any time an snprintf writes more than size value, this error will be returned */ 00143 SHADOW_JSON_BUFFER_TRUNCATED = -44, 00144 /** Any time an snprintf encounters an encoding error or not enough space in the given buffer */ 00145 SHADOW_JSON_ERROR = -45, 00146 /** Mutex initialization failed */ 00147 MUTEX_INIT_ERROR = -46, 00148 /** Mutex lock request failed */ 00149 MUTEX_LOCK_ERROR = -47, 00150 /** Mutex unlock request failed */ 00151 MUTEX_UNLOCK_ERROR = -48, 00152 /** Mutex destroy failed */ 00153 MUTEX_DESTROY_ERROR = -49, 00154 } IoT_Error_t; 00155 00156 #ifdef __cplusplus 00157 } 00158 #endif 00159 00160 #endif /* AWS_IOT_SDK_SRC_IOT_ERROR_H_ */
Generated on Tue Jul 12 2022 11:16:36 by
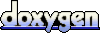