PushDuration maps n callback functions to the duration of a button press. E.g. foo() is called when a button is released after 1 second where bar() is called after 3 seconds.
PushDuration.h
00001 /** 00002 * PushDuration Library by Jens Strümper. For questions or suggestions on how to improve the 00003 * library please contact me via email: jens.struemper@gmail.com 00004 **/ 00005 #ifndef __PUSHDURATION_H_INCLUDED__ 00006 #define __PUSHDURATION_H_INCLUDED__ 00007 00008 namespace js{ 00009 00010 /** 00011 * PushDuration calls a function based on the duration of a button push 00012 * 00013 * The following code demonstrates the usage of the PushDuration library. 00014 * The library is meant for cases where different callbank functions are triggered by 00015 * different button push durations. E.g function "state1" is called when the button is 00016 * released after 1 second, "state4" is called when the button is released after 4 seconds 00017 * and so on. Please note that the implementation is far from being perfect. E.g. it 00018 * will not work if you instantiate the class in ram. 00019 00020 * Paramters are:- 00021 * <ul> 00022 * <li> Key value table for function <-> duration assignment. </li> 00023 * <li> PinName used for Button </li> 00024 * <li> Interval - typically "1" for full seconds </li> 00025 * </ul> 00026 * 00027 * 00028 * Example: 00029 * @code 00030 00031 00032 * #include "mbed.h" 00033 * #include "PushDuration.h" 00034 00035 * void state1() { 00036 * //function called after 1 seconds 00037 * printf("state1\n"); 00038 * } 00039 * void state4() { 00040 * //function called after 4 seconds 00041 * printf("state4\n"); 00042 * } 00043 * void state10() { 00044 * //function called after 4 seconds 00045 * printf("state8\n"); 00046 * } 00047 00048 * PinName pin(p17); 00049 00050 * //Action Table specifiying push duration and callback function 00051 * const action action_table[] = 00052 * { 00053 * { 1, state1 }, 00054 * { 4, state4 }, 00055 * { 10, state10 }, 00056 * }; 00057 00058 * int main() 00059 * //creating the ButtonHandler Object by specifiying arraysize, action table, pin and interval in seconds 00060 * { 00061 * ButtonHandler green( 00062 * sizeof(action_table)/sizeof(action_table[0]), 00063 * action_table, 00064 * pin, 00065 * 2 00066 * ); 00067 00068 * //enabling Button Handler 00069 * green.enable(); 00070 * while(1){ 00071 * printf("alive\n"); 00072 * wait(5); 00073 * } 00074 * } 00075 **/ 00076 00077 /** 00078 * struct to define duration and 00079 * callback function 00080 **/ 00081 00082 struct action 00083 { 00084 int counter_limit; 00085 void (*transition)(void); 00086 }; 00087 00088 /** 00089 * struct to hold table size and p 00090 * pointer to the first element of the table 00091 **/ 00092 00093 struct action_list 00094 { 00095 size_t size; 00096 const action *table; 00097 }; 00098 00099 00100 class ButtonHandler { 00101 public: 00102 /** 00103 * ButtonHandler Constructor: 00104 * 00105 * @param table_size sitze_t 00106 * @param begin const action* 00107 * @param pin PinName 00108 * @param seconds float 00109 **/ 00110 ButtonHandler(std::size_t table_size, const action* begin, PinName pin, float seconds ): buttonPin(pin){ 00111 mTable.size = table_size; 00112 mTable.table = begin; 00113 intervalInSeconds = seconds; 00114 printf("initialized\n"); 00115 } 00116 00117 /** 00118 * Enables Button Handler 00119 **/ 00120 void enable(); 00121 00122 /** 00123 * Disables Button Handler 00124 **/ 00125 00126 void disable(); 00127 00128 /** 00129 * ButtonHandler Destructor 00130 **/ 00131 virtual ~ButtonHandler() { 00132 disable(); 00133 } 00134 protected: 00135 void press(); 00136 void secondsCount(); 00137 void release(); 00138 void react(int counter) const; 00139 00140 private: 00141 volatile unsigned counter; 00142 InterruptIn buttonPin; 00143 float intervalInSeconds; 00144 action_list mTable; 00145 Ticker ticker; 00146 00147 }; 00148 }; //name space ends 00149 00150 using namespace js; 00151 00152 #endif //__PUSHDURATION_H_INCLUDED__
Generated on Fri Jul 29 2022 07:49:11 by
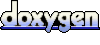