LedBlink library makes it possible to change blink frequency of a given LED by calling the Blink function with a float parameter elsewhere in the code to visualise state changes in a program.
LedBlink.cpp
00001 #include "mbed.h" 00002 #include "LedBlink.h" 00003 Ticker LedTicker; 00004 volatile float led_timer = 0; 00005 bool ChangeStatusFlag = false; 00006 00007 LedBlink::LedBlink(PinName pin) :_pin(pin) { 00008 _pin = 1; 00009 } 00010 00011 void LedBlink::LedBlinkCallback(void) { 00012 if (led_timer == 0){ 00013 _pin = 1; 00014 } 00015 if (led_timer != 0){ 00016 _pin = !_pin; 00017 if (ChangeStatusFlag) { 00018 // re-task ticker flash rate 00019 LedTicker.detach(); 00020 LedTicker.attach(this, &LedBlink::LedBlinkCallback,led_timer); 00021 ChangeStatusFlag = false; 00022 } 00023 } 00024 } 00025 00026 void LedBlink::Blinker(float frequency){ 00027 led_timer = frequency; 00028 ChangeStatusFlag = true; 00029 LedBlinkCallback(); 00030 }
Generated on Mon Jul 18 2022 00:08:22 by
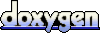