
This is the template with descriptions of the functionality and methods which needs to be implemented.
Embed:
(wiki syntax)
Show/hide line numbers
debounce_button.cpp
00001 #include "debounce_button.h" 00002 00003 /** 00004 Some tips and tricks: 00005 - To use the built-in LED: 00006 DigitalOut led1(LED1); 00007 ... 00008 led1 = 1; 00009 - To delay the call of a function: 00010 Timeout someTimeout; 00011 ... 00012 someTimeout.attach(callback(&someFunction), 0.5) with 0.5 as 500 milliseconds 00013 - The variables that are used in interrupt callbacks have to be volatile, 00014 because these variables can change at any time. Therefore, the compiler is not 00015 going to make optimisations. 00016 */ 00017 00018 /** 00019 TODO 00020 ---- 00021 This function: 00022 - stores the amount of clicks in a variable which is read by the main loop. 00023 - resets the click counter which is used inside this file. 00024 - lowers a flag which tells the main loop that the user stopped pressing the button 00025 such that it can proceed its program. 00026 - turns the built-in LED off. Therefore, the user gets informed that the program stopped counting the clicks. 00027 */ 00028 void button1_multiclick_reset_cb(void) { 00029 00030 } 00031 00032 /** 00033 TODO 00034 ---- 00035 This function enables the button again, such that unwanted clicks of the bouncing button get ignored. 00036 */ 00037 void button1_enabled_cb(void) 00038 { 00039 00040 } 00041 00042 /** 00043 TODO 00044 ---- 00045 This function: 00046 - turns the built-in LED on, so the user gets informed that the program has started with counting clicks 00047 - disables the button such that the debouncer is active 00048 - enables the button again after a certain amount of time 00049 (use interrupts with "button1_enabled_cb()" as callback. 00050 - counts the amount of clicks within a period of 1 second 00051 - informs the main loop that the button has been pressed 00052 - informs the main loop that the user is clicking the button. 00053 Therefore, this main loop cannot continue its procedure until the clicks within 1 second have been counted. 00054 */ 00055 void button1_onpressed_cb(void) 00056 { 00057 00058 }
Generated on Wed Aug 10 2022 19:47:01 by
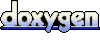