
WIZnet Remote Boat V1.0
Dependencies: Servo WizFi250Interface mbed
main.cpp
00001 #include <stdio.h> 00002 #include "mbed.h" 00003 #include "WizFi250Interface.h" 00004 #include "Servo.h" 00005 00006 00007 00008 Serial pc(USBTX, USBRX); 00009 00010 /* network setup */ 00011 WizFi250Interface wizfi250(P13,P14,P11,P12,P15,NC,115200); 00012 TCPSocketServer server; 00013 TCPSocketConnection client; 00014 00015 #define SECURE WizFi250::SEC_WPA2_MIXED 00016 #define SSID "WIZnet_Boat" 00017 #define PASS "wiznetboat" 00018 #define PORT 5000 00019 00020 00021 /* motor setup */ 00022 Servo servo(P23); 00023 PwmOut motor(P21); 00024 00025 00026 00027 /* direction define */ 00028 #define forward "WIZnet_boat_direction:forward" 00029 #define back "WIZnet_boat_direction:back" 00030 #define left "WIZnet_boat_direction:left" 00031 #define right "WIZnet_boat_direction:right" 00032 #define stop "WIZnet_boat_direction:stop" 00033 00034 00035 00036 /* Connection Steps */ 00037 typedef enum ConnectionStep { DISASSOCIATED = 1, ASSOCIATED, LISTENED, CONNECTED, DISCONNECTED } conStep; 00038 conStep ConnectionStep = DISASSOCIATED; 00039 00040 /* functions */ 00041 int TryApUp(); 00042 int TryServerUpAndWaitClient(); 00043 00044 00045 int main() 00046 { 00047 pc.baud(115200); 00048 motor.period_us(1000000/490); 00049 float speed = 0.0; 00050 00051 while(1) 00052 { 00053 char rcvBuf[1024] = {0,}; 00054 int len = 0; 00055 00056 switch(ConnectionStep) 00057 { 00058 case DISASSOCIATED: 00059 TryApUp(); 00060 break; 00061 00062 case ASSOCIATED: 00063 case LISTENED: 00064 TryServerUpAndWaitClient(); 00065 break; 00066 00067 case CONNECTED: 00068 00069 if( client.is_connected() == 0 ) ConnectionStep = DISCONNECTED; 00070 00071 if( wizfi250.readable(0) > 0 ) 00072 { 00073 len = client.receive(rcvBuf, 1023); 00074 if( len < 0 ) ConnectionStep = DISCONNECTED; 00075 else if( len > 0) 00076 { 00077 if( strcmp(rcvBuf,forward) == 0 ) 00078 { 00079 speed = speed + 0.01; 00080 printf("forward\r\n"); 00081 } 00082 else if( strcmp(rcvBuf,back) == 0 ) 00083 { 00084 speed = speed - 0.01; 00085 printf("back\r\n"); 00086 } 00087 else if( strcmp(rcvBuf,left) == 0 ) 00088 { 00089 servo = servo - 0.1; 00090 printf("left\r\n"); 00091 } 00092 else if( strcmp(rcvBuf,right) == 0 ) 00093 { 00094 servo = servo + 0.1; 00095 printf("right\r\n"); 00096 } 00097 else if( strcmp(rcvBuf,stop) == 0 ) 00098 { 00099 servo = 0.5; // center 00100 speed = 0; 00101 printf("stop\r\n"); 00102 } 00103 00104 if( speed < 0.55 ) speed = 0.55; 00105 else if ( speed > 1.0 ) speed = 1.0; 00106 printf("speed %.3f\r\n", speed); 00107 motor.write(speed); 00108 } 00109 } 00110 break; 00111 case DISCONNECTED: 00112 servo = 0.5; 00113 motor.write(0); 00114 ConnectionStep = LISTENED; 00115 } 00116 } 00117 } 00118 00119 int TryApUp() 00120 { 00121 00122 if( wizfi250.isAssociated() == 1 ) return -1; 00123 00124 printf(" AP UP\r\n"); 00125 printf("SSID: %s\r\nPASS: %s", SSID, PASS); 00126 00127 for(int i= 0; i<5; i++) 00128 { 00129 wizfi250.init("192.168.0.2","255.255.255.0","192.168.0.2"); 00130 if ( wizfi250.connect(SECURE, SSID, PASS, WizFi250::WM_AP) ) 00131 continue; 00132 else { 00133 printf("IP Address is %s\r\n", wizfi250.getIPAddress()); 00134 if( wizfi250.isAssociated() == 1 ) 00135 { 00136 ConnectionStep = ASSOCIATED; 00137 return 0; 00138 } else { 00139 continue; 00140 } 00141 } 00142 } 00143 00144 printf("Fail to make AP\r\n"); 00145 ConnectionStep = DISASSOCIATED; 00146 return -1; 00147 } 00148 00149 00150 int TryServerUpAndWaitClient() 00151 { 00152 if( wizfi250.isAssociated() != 1 ) return -1; 00153 00154 if( ConnectionStep == ASSOCIATED ) 00155 { 00156 if( server.bind(PORT) < 0 ) 00157 { 00158 printf("Bind fail..\r\n"); 00159 return -1; 00160 } 00161 00162 if( server.listen(1) < 0 ) 00163 { 00164 printf("Listen fail..\r\n"); 00165 return -1; 00166 } else { 00167 printf("Listen PORT: %d\r\n",PORT); 00168 ConnectionStep = LISTENED; 00169 } 00170 } 00171 00172 while(ConnectionStep == LISTENED) 00173 { 00174 if( server.accept(client) < 0 ) 00175 { 00176 printf("accept fail..\r\n"); 00177 return -1; 00178 }else{ 00179 printf("Connection Success!!\r\nIP: %s\r\n", client.get_address()); 00180 ConnectionStep = CONNECTED; 00181 return 0; 00182 } 00183 } 00184 00185 return -1; 00186 }
Generated on Fri Jul 29 2022 20:37:19 by
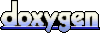