
Firmware enhancements for HSP_RPC_GUI 3.0.1
Fork of HSP_RPC_GUI by
S25FS512_RPC.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 00034 #include "S25FS512_RPC.h" 00035 #include "S25FS512.h" 00036 #include "StringInOut.h" 00037 #include "StringHelper.h" 00038 #include "Peripherals.h" 00039 #include "RpcServer.h" 00040 00041 int S25FS512_Reset(char argStrs[32][32], char replyStrs[32][32]) { 00042 uint32_t reply[1]; 00043 Peripherals::s25FS512()->reset(); 00044 reply[0] = 0x80; 00045 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00046 return 0; 00047 } 00048 00049 //****************************************************************************** 00050 int S25FS512_EnableHWReset(char argStrs[32][32], char replyStrs[32][32]) { 00051 uint32_t reply[1]; 00052 Peripherals::s25FS512()->enableHWReset(); 00053 reply[0] = 0x80; 00054 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00055 return 0; 00056 } 00057 00058 //****************************************************************************** 00059 int S25FS512_SpiWriteRead(char argStrs[32][32], char replyStrs[32][32]) { 00060 uint8_t args[16]; 00061 uint8_t reply[16]; 00062 uint8_t writeNumber; 00063 uint8_t readNumber; 00064 // get the number of bytes to write 00065 ProcessArgs(argStrs, args, 1); 00066 writeNumber = args[0]; 00067 ProcessArgs(argStrs, args, writeNumber + 2); 00068 readNumber = args[writeNumber + 1]; 00069 FormatReply(reply, readNumber, replyStrs); 00070 return 0; 00071 } 00072 00073 //****************************************************************************** 00074 int S25FS512_SpiWriteRead4Wire(char argStrs[32][32], char replyStrs[32][32]) { 00075 uint8_t args[16]; 00076 uint8_t reply[16]; 00077 uint8_t writeNumber; 00078 uint8_t readNumber; 00079 // get the number of bytes to write 00080 ProcessArgs(argStrs, args, 1); 00081 writeNumber = args[0]; 00082 ProcessArgs(argStrs, args, writeNumber + 2); 00083 readNumber = args[writeNumber + 1]; 00084 FormatReply(reply, readNumber, replyStrs); 00085 return 0; 00086 } 00087 00088 //****************************************************************************** 00089 int S25FS512_ReadPage(char argStrs[32][32], char replyStrs[32][32]) { 00090 uint32_t args[2]; 00091 uint32_t reply[1]; 00092 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00093 reply[0] = 0x80; 00094 FormatReply32(reply, sizeof(reply) / sizeof(uint32_t), replyStrs); 00095 return 0; 00096 } 00097 00098 //****************************************************************************** 00099 int S25FS512_ReadPagesBinary(char argStrs[32][32], char replyStrs[32][32]) { 00100 uint32_t args[2]; 00101 uint8_t pageData[258]; 00102 00103 uint32_t startPage; 00104 uint32_t endPage; 00105 uint32_t page; 00106 00107 // indicate that we are doing a flash binary transfer 00108 RPC_TransferingFlashPages(); 00109 00110 ProcessArgs32(argStrs, args, sizeof(args) / sizeof(uint32_t)); 00111 startPage = args[0]; 00112 endPage = args[1]; 00113 for (page = startPage; page <= endPage; page++) { 00114 Peripherals::s25FS512()->readPages_Helper(page, page, pageData, 0); 00115 //putBytes256Block(pageData, 1); 00116 pageData[sizeof(pageData) - 2] = 0x0D; 00117 pageData[sizeof(pageData) - 1] = 0x0A; 00118 putBytes(pageData, sizeof(pageData)); 00119 } 00120 return RPC_SKIP_CRLF; 00121 } 00122 00123 //****************************************************************************** 00124 int S25FS512_ReadId(char argStrs[32][32], char replyStrs[32][32]) { 00125 char str[32]; 00126 uint8_t data[128]; 00127 Peripherals::s25FS512()->readIdentification(data, sizeof(data)); 00128 Peripherals::s25FS512()->readIdentification(data, sizeof(data)); 00129 sprintf(str, "%02X%02X%02X%02X", data[0], data[1], data[2], data[3]); 00130 strcpy(replyStrs[0], str); 00131 return 0; 00132 }
Generated on Tue Jul 12 2022 22:52:24 by
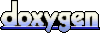