
Firmware enhancements for HSP_RPC_GUI 3.0.1
Fork of HSP_RPC_GUI by
HspBLE.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2016 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "HspBLE.h" 00034 #include "Peripherals.h" 00035 #include "MAX30001_helper.h" 00036 00037 #define LOW_BYTE(x) ((uint8_t)((x)&0xFF)) 00038 #define HIGH_BYTE(x) ((uint8_t)(((x) >> 8) & 0xFF)) 00039 00040 /// define all of the characteristic UUIDs 00041 uint8_t HspBLE::temperatureTopCharUUID[] = {0x35,0x44,0x53,0x1b,0x00,0xc3,0x43,0x42,0x97,0x55,0xb5,0x6a,0xbe,0x8e,0x6c,0x67}; 00042 uint8_t HspBLE::temperatureBottomCharUUID[] = {0x35,0x44,0x53,0x1b,0x00,0xc3,0x43,0x42,0x97,0x55,0xb5,0x6a,0xbe,0x8e,0x6a,0x66}; 00043 uint8_t HspBLE::accelerometerCharUUID[] = {0xe6,0xc9,0xda,0x1a,0x80,0x96,0x48,0xbc,0x83,0xa4,0x3f,0xca,0x38,0x37,0x05,0xaf}; 00044 uint8_t HspBLE::heartrateCharUUID[] = {0x62,0x1a,0x00,0xe3,0xb0,0x93,0x46,0xbf,0xaa,0xdc,0xab,0xe4,0xc6,0x48,0xc5,0x69}; 00045 uint8_t HspBLE::pressureCharUUID[] = {0x1d,0x8a,0x19,0x32,0xda,0x49,0x49,0xad,0x91,0xd8,0x80,0x08,0x32,0xe7,0xe9,0x40}; 00046 uint8_t HspBLE::dataCharUUID[] = {0xaa,0x8a,0x19,0x32,0xda,0x49,0x49,0xad,0x91,0xd8,0x80,0x08,0x32,0xe7,0xe9,0x40}; 00047 uint8_t HspBLE::commandCharUUID[] = {0x36,0xe5,0x5e,0x37,0x6b,0x5b,0x42,0x0b,0x91,0x07,0x0d,0x34,0xa0,0xe8,0x67,0x5a}; 00048 00049 00050 /// define the BLE device name 00051 uint8_t HspBLE::deviceName[] = "MAXREFDES100"; 00052 /// define the BLE serial number 00053 uint8_t HspBLE::serialNumber[] = {0x77, 0x22, 0x33, 0x45, 0x67, 0x89}; 00054 /// define the BLE service UUID 00055 uint8_t HspBLE::envServiceUUID[] = {0x5c,0x6e,0x40,0xe8,0x3b,0x7f,0x42,0x86,0xa5,0x2f,0xda,0xec,0x46,0xab,0xe8,0x51}; 00056 00057 HspBLE *HspBLE::instance = NULL; 00058 00059 /** 00060 * @brief Constructor that inits the BLE helper object 00061 * @param ble Pointer to the mbed BLE object 00062 */ 00063 HspBLE::HspBLE(BLE *ble) { 00064 bluetoothLE = new BluetoothLE(ble, NUMBER_OF_CHARACTERISTICS); 00065 instance = this; 00066 notificationUpdateRoundRobin = 0; 00067 } 00068 00069 /** 00070 * @brief Constructor that deletes the bluetoothLE object 00071 */ 00072 HspBLE::~HspBLE(void) { delete bluetoothLE; } 00073 00074 /** 00075 * @brief Initialize all of the HSP characteristics, initialize the ble service 00076 * and attach callbacks 00077 */ 00078 void HspBLE::init(void) { 00079 uint8_t *serialNumberPtr; 00080 // uint8_t serialNumberBuffer[6]; 00081 serialNumberPtr = MAX30001_Helper_getVersion(); 00082 printf("MAX30001 Version = %02X:%02X:%02X:%02X:%02X:%02X...\n", 00083 serialNumberPtr[0], serialNumberPtr[1], serialNumberPtr[2], 00084 serialNumberPtr[3], serialNumberPtr[4], serialNumberPtr[5]); 00085 serialNumberPtr[3] = 0x00; 00086 serialNumberPtr[4] = 0x00; 00087 serialNumberPtr[5] = 0x03; 00088 printf("BLE DeviceID = %02X:%02X:%02X:%02X:%02X:%02X...\n", 00089 serialNumberPtr[0], serialNumberPtr[1], serialNumberPtr[2], 00090 serialNumberPtr[3], serialNumberPtr[4], serialNumberPtr[5]); 00091 00092 bluetoothLE->addCharacteristic(new Characteristic(2 /* number of bytes */,temperatureTopCharUUID,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY)); 00093 bluetoothLE->addCharacteristic(new Characteristic(2 /* number of bytes */,temperatureBottomCharUUID,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY)); 00094 bluetoothLE->addCharacteristic(new Characteristic(6 /* number of bytes */,accelerometerCharUUID,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY)); 00095 bluetoothLE->addCharacteristic(new Characteristic(4 /* number of bytes */,heartrateCharUUID,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY)); 00096 bluetoothLE->addCharacteristic(new Characteristic(8 /* number of bytes */,pressureCharUUID,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_NOTIFY)); 00097 bluetoothLE->addCharacteristic(new Characteristic(32 /* number of bytes */,dataCharUUID,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE)); 00098 bluetoothLE->addCharacteristic(new Characteristic(1 /* number of bytes */,commandCharUUID,GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_READ | GattCharacteristic::BLE_GATT_CHAR_PROPERTIES_WRITE)); 00099 bluetoothLE->initService(serialNumberPtr, deviceName, sizeof(deviceName),envServiceUUID); 00100 00101 bluetoothLE->onDataWritten(&HspBLE::_onDataWritten); 00102 ticker.attach(callback(this, &HspBLE::tickerHandler), 1); 00103 } 00104 00105 void HspBLE::_onDataWritten(int index) { 00106 HspBLE::instance->onDataWritten(index); 00107 } 00108 00109 /** 00110 * @brief Callback for written characteristics 00111 * @param index Index of whose characteristic is written to 00112 */ 00113 void HspBLE::onDataWritten(int index) { 00114 int length; 00115 uint8_t *data; 00116 printf("onDataWritten "); 00117 if (index == CHARACTERISTIC_CMD) { 00118 data = bluetoothLE->getDataWritten(index, &length); 00119 if (length >= 1) { 00120 if (data[0] == 0x00) startDataLogging = false; 00121 if (data[0] == 0x01) startDataLogging = true; 00122 printf("onDataWritten index %d, data %02X, length %d ",index,data[0],length); fflush(stdout); 00123 00124 } 00125 } 00126 } 00127 00128 void HspBLE::pollSensor(int sensorId, uint8_t *data) { 00129 00130 switch (sensorId) { 00131 case CHARACTERISTIC_TEMP_TOP: { 00132 uint16_t uShort; 00133 Peripherals::max30205_top()->readTemperature(&uShort); 00134 data[0] = HIGH_BYTE(uShort); 00135 data[1] = LOW_BYTE(uShort); 00136 } break; 00137 case CHARACTERISTIC_TEMP_BOTTOM: { 00138 uint16_t uShort; 00139 Peripherals::max30205_bottom()->readTemperature(&uShort); 00140 data[0] = HIGH_BYTE(uShort); 00141 data[1] = LOW_BYTE(uShort); 00142 } break; 00143 case CHARACTERISTIC_ACCELEROMETER: { 00144 unsigned int i; 00145 uint8_t *bytePtr; 00146 int16_t acclPtr[3]; 00147 Peripherals::lis2dh()->get_motion_cached(&acclPtr[0], &acclPtr[1], 00148 &acclPtr[2]); 00149 bytePtr = reinterpret_cast<uint8_t *>(&acclPtr); 00150 for (i = 0; i < sizeof(acclPtr); i++) 00151 data[i] = bytePtr[i]; 00152 } break; 00153 case CHARACTERISTIC_PRESSURE: { 00154 unsigned int i; 00155 uint8_t *bytePtr; 00156 float temperature; 00157 float pressure; 00158 Peripherals::bmp280()->ReadCompData(&temperature, &pressure); 00159 bytePtr = reinterpret_cast<uint8_t *>(&temperature); 00160 for (i = 0; i < sizeof(float); i++) 00161 data[i] = bytePtr[i]; 00162 bytePtr = reinterpret_cast<uint8_t *>(&pressure); 00163 for (i = 0; i < sizeof(float); i++) 00164 data[i + sizeof(float)] = bytePtr[i]; 00165 } break; 00166 case CHARACTERISTIC_HEARTRATE: { 00167 unsigned int i; 00168 uint8_t *bytePtr; 00169 MAX30001::max30001_bledata_t heartrateData; 00170 Peripherals::max30001()->ReadHeartrateData(&heartrateData); 00171 bytePtr = reinterpret_cast<uint8_t *>(&heartrateData); 00172 for (i = 0; i < sizeof(MAX30001::max30001_bledata_t); i++) 00173 data[i] = bytePtr[i]; 00174 } break; 00175 } 00176 } 00177 00178 bool HspBLE::getStartDataLogging(void) { return startDataLogging; } 00179 00180 /** 00181 * @brief Timer Callback that updates all sensor characteristic notifications 00182 */ 00183 void HspBLE::tickerHandler(void) { 00184 uint8_t data[8]; 00185 if (bluetoothLE->isConnected()) { 00186 pollSensor(CHARACTERISTIC_TEMP_TOP, data); 00187 bluetoothLE->notifyCharacteristic(CHARACTERISTIC_TEMP_TOP, data); 00188 pollSensor(CHARACTERISTIC_TEMP_BOTTOM, data); 00189 bluetoothLE->notifyCharacteristic(CHARACTERISTIC_TEMP_BOTTOM, data); 00190 pollSensor(CHARACTERISTIC_ACCELEROMETER, data); 00191 bluetoothLE->notifyCharacteristic(CHARACTERISTIC_ACCELEROMETER, data); 00192 pollSensor(CHARACTERISTIC_HEARTRATE, data); 00193 bluetoothLE->notifyCharacteristic(CHARACTERISTIC_HEARTRATE, data); 00194 pollSensor(CHARACTERISTIC_PRESSURE, data); 00195 bluetoothLE->notifyCharacteristic(CHARACTERISTIC_PRESSURE, data); 00196 } 00197 }
Generated on Tue Jul 12 2022 22:52:23 by
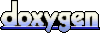