Happy Turkey Day
Embed:
(wiki syntax)
Show/hide line numbers
GPSINT.h
00001 /* GPSINT.cpp 00002 * jbradshaw (20141101) 00003 * GPS functions are work of Tyler Weavers mbed gps library page 00004 * (http://mbed.org/users/tylerjw/code/GPS/file/39d75e44b214/GPS.cpp) 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 /* This GPSINT library class retrieves, parses, and updates variables using 00026 a serial receive interrupt. The library was written using the Trimble 00027 Copernicus II moduls bus should work on any NMEA 4800 baud output device. 00028 The relatively slow 4800 baud allows for plenty of processing time during 00029 byte receptions in the interrupt. Using the mbed LPC 100MHz unit, it takes 00030 about 340us to process, and parse the 2 default GPGGA and GPZTG strings.*/ 00031 00032 #include "mbed.h" 00033 00034 #ifndef GPSINT_H 00035 #define GPSINT_H 00036 00037 #define PI (3.14159265359) 00038 #define GPSBUFSIZE 256 // GPS buffer size 00039 00040 /** 00041 * GPSINT Class. 00042 */ 00043 00044 class GPSINT{ 00045 public: 00046 /** 00047 * Constructor. 00048 * @param gps - Serial port pins attached to the gps 00049 */ 00050 GPSINT(PinName tx, PinName rx); 00051 int nmea_validate(char *nmeastr); //runs the checksum calculation on the GPS NMEA string 00052 void parseGPSString(char *GPSstrParse); //uses scanf to parse NMEA string into variables 00053 void GPSSerialRecvInterrupt(void); //fills temprpary buffer for processing 00054 float nmea_to_dec(float deg_coord, char nsew); //convert nmea format to decimal format 00055 float calc_course_to(float pointLat, float pontLong); 00056 double calc_dist_to_mi(float pointLat, float pontLong); 00057 double calc_dist_to_ft(float pointLat, float pontLong); 00058 double calc_dist_to_km(float pointLat, float pontLong); 00059 double calc_dist_to_m(float pointLat, float pontLong); 00060 00061 // GPSINT variables 00062 char GPSbuf[GPSBUFSIZE]; // Receive buffer. 00063 char Temp_GPSbuf[GPSBUFSIZE];// Receive buffer. 00064 char GPSidx; // Read by background, written by Int Handler. 00065 int GPSstate; //set when successful GPS string is received 00066 00067 // calculated values 00068 float dec_longitude; 00069 float dec_latitude; 00070 float altitude_ft; 00071 00072 // GGA - Global Positioning System Fixed Data 00073 float nmea_longitude; 00074 float nmea_latitude; 00075 float utc_time; 00076 char ns, ew; 00077 int lock; 00078 char status; 00079 int satelites; 00080 float hdop; 00081 float msl_altitude; 00082 char msl_units; 00083 00084 // RMC - Recommended Minimmum Specific GNS Data 00085 char rmc_status; 00086 float speed_k; 00087 float course_d; 00088 int date; 00089 00090 // GLL 00091 char gll_status; 00092 00093 // VTG - Course over ground, ground speed 00094 float course_t; // ground speed true 00095 char course_t_unit; 00096 float course_m; // magnetic 00097 char course_m_unit; 00098 char speed_k_unit; 00099 float speed_km; // speek km/hr 00100 char speed_km_unit; 00101 private: 00102 Serial _gps; 00103 }; 00104 00105 #endif /* GPSINT_H */
Generated on Tue Jul 12 2022 17:50:07 by
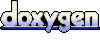