
control de velocidad progresivo de un motor de CC en ambas direcciones
Fork of pwm_example by
main.cpp
00001 /* PWM motor driving example on LPC1768 00002 * 00003 * @author: Baser Kandehir 00004 * @date: July 9, 2015 00005 * @license: MIT license 00006 * 00007 * Copyright (c) 2015, Baser Kandehir, baser.kandehir@ieee.metu.edu.tr 00008 * 00009 * Permission is hereby granted, free of charge, to any person obtaining a copy 00010 * of this software and associated documentation files (the "Software"), to deal 00011 * in the Software without restriction, including without limitation the rights 00012 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00013 * copies of the Software, and to permit persons to whom the Software is 00014 * furnished to do so, subject to the following conditions: 00015 * 00016 * The above copyright notice and this permission notice shall be included in 00017 * all copies or substantial portions of the Software. 00018 * 00019 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00020 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00021 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00022 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00023 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00024 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00025 * THE SOFTWARE. 00026 * 00027 * @description of the program: 00028 * 00029 * Program is written as an example for the PWM control on mbed LPC1768. 00030 * After making neccessary connections, this program can control speed 00031 * and direction of a motor. Example code will increase motor speed to 00032 * its max in 5 seconds and decrease the motor speed from max to zero 00033 * in 5 seconds and it will keep doing this. 00034 * 00035 * @connections: 00036 *-------------------------------------------------------------- 00037 * |LPC1768| |Peripherals| 00038 * Pin 21 --------> First input of the L293D 00039 * Pin 22 --------> Second input of the L293D 00040 * GND -----------> GND of any peripheral 00041 * VOUT (3.3 V) --> VCC of the the motor driver 00042 *--------------------------------------------------------------- 00043 * Note: L293D is a very simple and useful motor driver that mostly used in 00044 * robotics. For detailed info and neccessary connections for L293D, please 00045 * refer to its datasheet. 00046 */ 00047 00048 #include "mbed.h" 00049 00050 PwmOut PWM1(PF_8); // pwm outputs 00051 PwmOut PWM2(PF_7); 00052 DigitalOut led1(LED1); 00053 00054 /* Function prototype */ 00055 void motorControl(bool dir, float pwmVal); 00056 00057 int main() 00058 { 00059 00060 led1 = 1; 00061 wait(1); 00062 led1 = 0; 00063 PWM1.write(0); // Duty cycles are initially zero 00064 PWM2.write(0); 00065 PWM1.period(0.02f); // 1 kHz pwm frequency 00066 PWM2.period(0.02f); 00067 00068 while(1) 00069 { 00070 for(float i=0;i<1;i=i+0.01f) // increase speed from 0 to 1 in 5 seconds (50ms*100). 00071 { 00072 motorControl(1,i); 00073 wait(0.05f); 00074 } 00075 for(float i=1;i>0;i=i-0.01f) // decrease speed from 1 to 0 in 5 seconds (50ms*100). 00076 { 00077 motorControl(0,i); 00078 wait(0.05f); 00079 } 00080 led1 = 1; 00081 wait(0.5); 00082 led1 = 0; 00083 } 00084 } 00085 00086 // This is a motor control function which controls the speed and direction of 00087 // a motor. Motor drivers like L293D can be used. 00088 void motorControl(bool dir, float pwmVal) 00089 { 00090 if(dir==1) // forward direction 00091 { 00092 PWM2.write(0); 00093 PWM1.write(pwmVal); 00094 } 00095 else if(dir==0) // reverse direction 00096 { 00097 PWM1.write(0); 00098 PWM2.write(pwmVal); 00099 } 00100 }
Generated on Mon Jul 25 2022 23:57:29 by
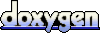