
code for the receiver
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "XBeeLib.h" 00003 00004 using namespace XBeeLib; 00005 00006 Serial pc(USBTX, USBRX); 00007 00008 bool capt_1; 00009 bool capt_2; 00010 bool capt_3; 00011 bool capt_4; 00012 bool capt_5; 00013 bool capt_6; 00014 00015 /** Callback function, invoked at packet reception */ 00016 static void receive_cb(const RemoteXBeeZB& remote, bool broadcast, const uint8_t *const data, uint16_t len) 00017 { 00018 const uint64_t remote_addr64 = remote.get_addr64(); 00019 00020 pc.printf("\r\nGot packet, len %d\r\nData: ", len); 00021 00022 for (int i = 0; i < len; i++) 00023 { 00024 pc.printf("%02x ", data[i]); 00025 } 00026 00027 capt_1 = data[0] >> 7; 00028 capt_2 = data[0] >> 6; 00029 capt_3 = data[0] >> 5; 00030 capt_4 = data[0] >> 4; 00031 capt_5 = data[0] >> 3; 00032 capt_6 = data[0] >> 2; 00033 00034 pc.printf("\r\n"); 00035 } 00036 00037 int main() 00038 { 00039 XBeeZB xbee = XBeeZB(p13, p14, NC, NC, NC, 9600); 00040 00041 /* Register callbacks */ 00042 xbee.register_receive_cb(&receive_cb); 00043 00044 RadioStatus const radioStatus = xbee.init(); 00045 MBED_ASSERT(radioStatus == Success); 00046 00047 /* Wait until the device has joined the network */ 00048 pc.printf("Waiting for device to join the network: "); 00049 while (!xbee.is_joined()) { 00050 wait_ms(1000); 00051 } 00052 pc.printf("OK\r\n"); 00053 00054 while (true) { 00055 xbee.process_rx_frames(); 00056 wait_ms(100); 00057 } 00058 }
Generated on Sun Jul 17 2022 01:41:50 by
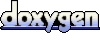