
New project
Embed:
(wiki syntax)
Show/hide line numbers
Track.cpp
00001 #include "Track.h" 00002 00003 Track::Track () : trackpin(p20), lcd(p22, p21, p23, p24, p25, p26), myled1(LED1) 00004 { 00005 //ctor 00006 address = 0; 00007 inst = 0; 00008 myled1 = 1; 00009 } 00010 00011 Track::~Track() 00012 { 00013 //dtor 00014 } 00015 00016 void Track::DCC_send_command(unsigned int address, unsigned int inst, unsigned int repeat_count) 00017 { 00018 unsigned __int64 command = 0x0000000000000000; // __int64 is the 64-bit integer type 00019 unsigned __int64 temp_command = 0x0000000000000000; 00020 unsigned __int64 prefix = 0x3FFF; // 14 "1" bits needed at start 00021 unsigned int error = 0x00; //error byte 00022 //calculate error detection byte with xor 00023 error = address ^ inst; 00024 //combine packet bits in basic DCC format 00025 command = (prefix<<28)|(address<<19)|(inst<<10)|((error)<<1)|0x01; 00026 //printf("\n\r %llx \n\r",command); 00027 int i=0; 00028 //repeat DCC command lots of times 00029 while(i < repeat_count) { 00030 temp_command = command; 00031 //loops through packet bits encoding and sending out digital pulses for a DCC command 00032 for (int j=0; j<64; j++) { 00033 if((temp_command&0x8000000000000000)==0) { //test packet bit 00034 //send data for a "0" bit 00035 trackpin=0; 00036 wait_us(100); 00037 trackpin=1; 00038 wait_us(100); 00039 } else { 00040 //send data for a "1"bit 00041 trackpin=0; 00042 wait_us(58); 00043 trackpin=1; 00044 wait_us(58); 00045 } 00046 // next bit in packet 00047 temp_command = temp_command<<1; 00048 } 00049 i++; 00050 } 00051 }
Generated on Fri Jul 22 2022 17:31:31 by
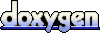