
New project
Embed:
(wiki syntax)
Show/hide line numbers
MCP23017.h
00001 /* MCP23017 - drive the Microchip MCP23017 16-bit Port Extender using I2C 00002 * Copyright (c) 2010 Wim Huiskamp, Romilly Cocking (original version for SPI) 00003 * 00004 * Changed by Jacco van Splunter: 00005 * The _read and _write functions are moved from protected -> public 00006 * 00007 * Released under the MIT License: http://mbed.org/license/mit 00008 * 00009 * version 0.2 Initial Release 00010 * version 0.3 Cleaned up 00011 * version 0.4 Fixed problem with _read method 00012 * version 0.5 Added support for 'Banked' access to registers 00013 */ 00014 #include "mbed.h" 00015 00016 #ifndef MCP23017_H 00017 #define MCP23017_H 00018 00019 // All register addresses assume IOCON.BANK = 0 (POR default) 00020 #define IODIRA 0x00 00021 #define IODIRB 0x01 00022 #define IPOLA 0x02 00023 #define IPOLB 0x03 00024 #define GPINTENA 0x04 00025 #define GPINTENB 0x05 00026 #define DEFVALA 0x06 00027 #define DEFVALB 0x07 00028 #define INTCONA 0x08 00029 #define INTCONB 0x09 00030 #define IOCONA 0x0A 00031 #define IOCONB 0x0B 00032 #define GPPUA 0x0C 00033 #define GPPUB 0x0D 00034 #define INTFA 0x0E 00035 #define INTFB 0x0F 00036 #define INTCAPA 0x10 00037 #define INTCAPB 0x11 00038 #define GPIOA 0x12 00039 #define GPIOB 0x13 00040 #define OLATA 0x14 00041 #define OLATB 0x15 00042 00043 // The following register addresses assume IOCON.BANK = 1 00044 #define IODIRA_BNK 0x00 00045 #define IPOLA_BNK 0x01 00046 #define GPINTENA_BNK 0x02 00047 #define DEFVALA_BNK 0x03 00048 #define INTCONA_BNK 0x04 00049 #define IOCONA_BNK 0x05 00050 #define GPPUA_BNK 0x06 00051 #define INTFA_BNK 0x07 00052 #define INTCAPA_BNK 0x08 00053 #define GPIOA_BNK 0x09 00054 #define OLATA_BNK 0x0A 00055 00056 #define IODIRB_BNK 0x10 00057 #define IPOLB_BNK 0x11 00058 #define GPINTENB_BNK 0x12 00059 #define DEFVALB_BNK 0x13 00060 #define INTCONB_BNK 0x14 00061 #define IOCONB_BNK 0x15 00062 #define GPPUB_BNK 0x16 00063 #define INTFB_BNK 0x17 00064 #define INTCAPB_BNK 0x18 00065 #define GPIOB_BNK 0x19 00066 #define OLATB_BNK 0x1A 00067 00068 // This array allows structured access to Port_A and Port_B registers for both bankModes 00069 const int IODIR_AB[2][2] = {{IODIRA, IODIRB}, {IODIRA_BNK, IODIRB_BNK}}; 00070 const int IPOL_AB[2][2] = {{IPOLA, IPOLB}, {IPOLA_BNK, IPOLB_BNK}}; 00071 const int GPINTEN_AB[2][2] = {{GPINTENA, GPINTENB}, {GPINTENA_BNK, GPINTENB_BNK}}; 00072 const int DEFVAL_AB[2][2] = {{DEFVALA, DEFVALB}, {DEFVALA_BNK, DEFVALB_BNK}}; 00073 const int INTCON_AB[2][2] = {{INTCONA, INTCONB}, {INTCONA_BNK, INTCONB_BNK}}; 00074 const int IOCON_AB[2][2] = {{IOCONA, IOCONB}, {IOCONA_BNK, IOCONB_BNK}}; 00075 const int GPPU_AB[2][2] = {{GPPUA, GPPUB}, {GPPUA_BNK, GPPUB_BNK}}; 00076 const int INTF_AB[2][2] = {{INTFA, INTFB}, {INTFA_BNK, INTFB_BNK}}; 00077 const int INTCAP_AB[2][2] = {{INTCAPA, INTCAPB}, {INTCAPA_BNK, INTCAPB_BNK}}; 00078 const int GPIO_AB[2][2] = {{GPIOA, GPIOB}, {GPIOA_BNK, GPIOB_BNK}}; 00079 const int OLAT_AB[2][2] = {{OLATA, OLATB}, {OLATA_BNK, OLATB_BNK}}; 00080 00081 00082 // Control settings 00083 #define IOCON_BANK 0x80 // Banked registers for Port A and B 00084 #define IOCON_BYTE_MODE 0x20 // Disables sequential operation, Address Ptr does not increment 00085 // If Disabled and Bank = 0, operations toggle between Port A and B registers 00086 // If Disabled and Bank = 1, operations do not increment registeraddress 00087 #define IOCON_HAEN 0x08 // Hardware address enable 00088 00089 #define INTERRUPT_POLARITY_BIT 0x02 00090 #define INTERRUPT_MIRROR_BIT 0x40 00091 00092 #define PORT_DIR_OUT 0x00 00093 #define PORT_DIR_IN 0xFF 00094 00095 enum Polarity { ACTIVE_LOW , ACTIVE_HIGH }; 00096 enum Port { PORT_A=0, PORT_B=1 }; 00097 enum Bank { NOT_BNK=0, BNK=1 }; 00098 00099 class MCP23017 { 00100 public: 00101 /** Create an MCP23017 object connected to the specified I2C object and using the specified deviceAddress 00102 * 00103 * @param I2C &i2c the I2C port to connect to 00104 * @param char deviceAddress the address of the MCP23017 00105 */ 00106 MCP23017(I2C &i2c, char deviceAddress); 00107 00108 /** Set I/O direction of specified MCP23017 Port 00109 * 00110 * @param Port Port address (Port_A or Port_B) 00111 * @param char direction pin direction (0 = output, 1 = input) 00112 */ 00113 void direction(Port port, char direction); 00114 00115 /** Set Pull-Up Resistors on specified MCP23017 Port 00116 * 00117 * @param Port Port address (Port_A or Port_B) 00118 * @param char offOrOn per pin (0 = off, 1 = on) 00119 */ 00120 void configurePullUps(Port port, char offOrOn); 00121 00122 void configureBanked(Bank bankmode); 00123 void interruptEnable(Port port, char interruptsEnabledMask); 00124 void interruptPolarity(Polarity polarity); 00125 void mirrorInterrupts(bool mirror); 00126 void defaultValue(Port port, char valuesToCompare); 00127 void interruptControl(Port port, char interruptControlBits); 00128 00129 /** Read from specified MCP23017 Port 00130 * 00131 * @param Port Port address (Port_A or Port_B) 00132 * @returns data from Port 00133 */ 00134 char read(Port port); 00135 00136 /** Write to specified MCP23017 Port 00137 * 00138 * @param Port Port address (Port_A or Port_B) 00139 * @param char byte data to write 00140 */ 00141 void write(Port port, char byte); 00142 00143 /** Write to specified MCP23017 register 00144 * 00145 * @param char address the internal registeraddress of the MCP23017 00146 */ 00147 void _write(char address, char byte); 00148 00149 /** Read from specified MCP23017 register 00150 * 00151 * @param char address the internal registeraddress of the MCP23017 00152 * @returns data from register 00153 */ 00154 char _read(char address); 00155 00156 protected: 00157 I2C &_i2c; 00158 char _readOpcode; 00159 char _writeOpcode; 00160 Bank _bankMode; 00161 00162 /** Init MCP23017 00163 * 00164 * @param 00165 * @returns 00166 */ 00167 void _init(); 00168 }; 00169 00170 #endif
Generated on Fri Jul 22 2022 17:31:31 by
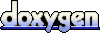