
FXOS8700CQ demo for mbed OS 5
Fork of fxos8700cq_example by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 #include "FXOS8700CQ.h" 00003 00004 #define DATA_RECORD_TIME_MS 1000 00005 00006 Serial pc(USBTX, USBRX); // Primary output to demonstrate library 00007 00008 // Pin names for FRDM-K64F 00009 FXOS8700CQ fxos(PTE25, PTE24, FXOS8700CQ_SLAVE_ADDR1); // SDA, SCL, (addr << 1) 00010 00011 DigitalOut green(LED_GREEN); // waiting light 00012 DigitalOut blue(LED_BLUE); // collection-in-progress light 00013 DigitalOut red(LED_RED); // completed/error ligt 00014 00015 Timer t; // Microsecond timer, 32 bit int, maximum count of ~30 minutes 00016 InterruptIn fxos_int1(PTC6); // unused, common with SW2 on FRDM-K64F 00017 InterruptIn fxos_int2(PTC13); // should just be the Data-Ready interrupt 00018 InterruptIn start_sw(PTA4); // switch SW3 00019 00020 // Interrupt status flags and data 00021 bool fxos_int1_triggered = false; 00022 bool fxos_int2_triggered = false; 00023 uint32_t us_ellapsed = 0; 00024 bool start_sw_triggered = false; 00025 00026 void trigger_fxos_int1(void) 00027 { 00028 fxos_int1_triggered = true; 00029 } 00030 00031 void trigger_fxos_int2(void) 00032 { 00033 fxos_int2_triggered = true; 00034 us_ellapsed = t.read_us(); 00035 } 00036 00037 void trigger_start_sw(void) 00038 { 00039 start_sw_triggered = true; 00040 } 00041 00042 void print_reading() 00043 { 00044 pc.printf("%d A X:%5d,Y:%5d,Z:%5d M X:%5d,Y:%5d,Z:%5d\r\n", 00045 us_ellapsed, 00046 fxos.getAccelX(), fxos.getAccelY(), fxos.getAccelZ(), 00047 fxos.getMagnetX(), fxos.getMagnetY(), fxos.getMagnetZ()); 00048 } 00049 00050 int main(void) 00051 { 00052 // Setup 00053 t.reset(); 00054 pc.baud(115200); // Print quickly! 200Hz x line of output data! 00055 00056 // Lights off (FRDM-K64F has active-low LEDs) 00057 green.write(1); 00058 red.write(1); 00059 blue.write(1); 00060 00061 // Diagnostic printing of the FXOS WHOAMI register value 00062 printf("\r\n\nFXOS8700Q Who Am I= %X\r\n", fxos.get_whoami()); 00063 00064 // Iterrupt for active-low interrupt line from FXOS 00065 // Configured with only one interrupt on INT2 signaling Data-Ready 00066 fxos_int2.fall(&trigger_fxos_int2); 00067 fxos.enable(); 00068 00069 // Interrupt for SW3 button-down state 00070 start_sw.mode(PullUp); // Since the FRDM-K64F doesn't have its SW2/SW3 pull-ups populated 00071 start_sw.fall(&trigger_start_sw); 00072 00073 green.write(0); // ready-green on 00074 00075 // Example data printing 00076 fxos.get_data(); 00077 print_reading(); 00078 00079 pc.printf("Waiting for data collection trigger on SW3\r\n"); 00080 00081 while(true) { 00082 if(start_sw_triggered) { 00083 break; 00084 } 00085 wait_ms(50); // just to slow the loop, fast enough for UX 00086 } 00087 00088 green.write(1); // ready-green off 00089 blue.write(0); // working-blue on 00090 00091 pc.printf("Started data collection. Accelerometer at max %dg.\r\n", 00092 fxos.get_accel_scale()); 00093 00094 fxos.get_data(); // clear interrupt from device 00095 fxos_int2_triggered = false; // un-trigger 00096 00097 t.start(); // start timer and enter collection loop 00098 while (t.read_ms() <= DATA_RECORD_TIME_MS) { 00099 00100 if(fxos_int2_triggered) { 00101 fxos_int2_triggered = false; // un-trigger 00102 fxos.get_data(); 00103 print_reading(); // outpouring of data !! 00104 } 00105 00106 // Continuous polling of interrupt status is not efficient, but... 00107 wait_us(500); // 1/10th the period of the 200Hz sample rate 00108 } 00109 00110 blue.write(1); // working-blue off 00111 red.write(0); // complete-red on 00112 00113 pc.printf("Done. Reset to repeat.\r\n"); 00114 00115 while(true) { 00116 pc.putc('.'); // idle dots 00117 wait(1.0); 00118 } 00119 }
Generated on Fri Jul 15 2022 10:57:01 by
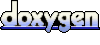