A simple compass using the BBC Micro:Bit. Uses a point-line distance calculation to light the LEDs instead of commonly used pre-calculated LED Matrix which gives nicer representation of the direction.
main.cpp
00001 /* See 00002 * http://lancaster-university.github.io/microbit-docs/advanced/ 00003 * for docs about using the micro:bit library 00004 */ 00005 #ifndef M_PI 00006 #define M_PI 3.14159265358979323846 00007 #endif 00008 00009 #include "MicroBit.h" 00010 00011 // a,b line normal vector coordinates, should be normalized, a^2 + b^2 = 1 00012 // returns if distance of point at coordinates x, y and vector line does not 00013 // exceed given range 00014 bool inRange(float a, float b, float x, float y, float range = 0.5) 00015 { 00016 return abs(a*x + b*y) < range; 00017 } 00018 // answer if the LED in row, column should be lit or turned off 00019 bool isLit(float a, float b, int row, int column) 00020 { 00021 float x = column - 2; 00022 float y = 2 - row; 00023 return inRange(a,b,x,y); 00024 } 00025 00026 float deg2rad(int deg) 00027 { 00028 return (float) deg * M_PI / 180; 00029 } 00030 00031 MicroBit uBit; 00032 00033 int main() 00034 { 00035 00036 00037 uBit.init(); 00038 00039 while (true) { 00040 int heading = uBit.compass.heading(); 00041 //uBit.serial.printf("%d\r\n", heading); 00042 00043 float angle = M_PI / 2 - deg2rad(heading); //calculate angle of displayed line 00044 float a = sin(angle); //calculate line normal vector parameters a, b 00045 float b = cos(angle); 00046 00047 for (int row = 0; row < 5; row++) { 00048 for (int col = 0; col < 5; col++) { 00049 uBit.display.image.setPixelValue(col,row,isLit(a, b, row, col) ? 255 : 0); 00050 } 00051 } 00052 00053 uBit.sleep (100); 00054 } 00055 } 00056
Generated on Sat Jul 16 2022 02:39:22 by
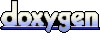