
FINAL VERSION
Gamepad Class Reference
#include <Gamepad.h>
Public Types | |
enum | GamepadEvent { A_PRESSED, B_PRESSED, X_PRESSED, Y_PRESSED, L_PRESSED, R_PRESSED, BACK_PRESSED, START_PRESSED, JOY_PRESSED, N_EVENTS } |
Gamepad events. More... | |
Public Member Functions | |
Gamepad () | |
Constructor. | |
~Gamepad () | |
Destructor. | |
void | init () |
Initialise all peripherals and configure interrupts. | |
void | leds_on () |
Turn all LEDs on. | |
void | leds_off () |
Turn all LEDs off. | |
void | leds (float val) const |
Set all LEDs to duty-cycle. | |
void | led (int n, float val) const |
Set LED to duty-cycle. | |
float | read_pot () const |
Read potentiometer. | |
void | tone (float frequency, float duration) |
Play tone on piezo. | |
bool | check_event (GamepadEvent const id) |
Check whether an event flag has been set and clear it. | |
void | reset_flags () |
Reset all button flags. | |
float | get_mag () |
Get magnitude of joystick movement. | |
float | get_angle () |
Get angle of joystick movement. | |
Direction | get_direction () |
Gets joystick direction. | |
Vector2D | get_coord () |
Gets raw cartesian co-ordinates of joystick. | |
Vector2D | get_mapped_coord () |
Gets cartesian coordinates mapped to circular grid. | |
Polar | get_polar () |
Gets polar coordinates of the joystick. |
Detailed Description
Gamepad Class.
Library for interfacing with ELEC2645 Gamepad PCB, University of Leeds
- Date:
- Febraury 2017
Definition at line 52 of file Gamepad.h.
Member Enumeration Documentation
enum GamepadEvent |
Gamepad events.
List of events that can be registered on the gamepad
- Enumerator:
Constructor & Destructor Documentation
Gamepad | ( | ) |
Constructor.
Definition at line 6 of file Gamepad.cpp.
~Gamepad | ( | ) |
Destructor.
Definition at line 39 of file Gamepad.cpp.
Member Function Documentation
bool check_event | ( | GamepadEvent const | id ) |
Check whether an event flag has been set and clear it.
- Parameters:
-
id[in] The ID of the event to test
- Returns:
- true if the event occurred
Definition at line 141 of file Gamepad.cpp.
float get_angle | ( | ) |
Get angle of joystick movement.
- Returns:
- value in range 0.0 to 359.9. 0.0 corresponds to N, 180.0 to S. -1.0 is central
Definition at line 173 of file Gamepad.cpp.
Vector2D get_coord | ( | ) |
Gets raw cartesian co-ordinates of joystick.
- Returns:
- a struct with x,y members, each in the range 0.0 to 1.0
Definition at line 289 of file Gamepad.cpp.
Direction get_direction | ( | ) |
Gets joystick direction.
- Returns:
- an enum: CENTRE, N, NE, E, SE, S, SW, W, NW,
Definition at line 179 of file Gamepad.cpp.
float get_mag | ( | ) |
Get magnitude of joystick movement.
- Returns:
- value in range 0.0 to 1.0
Definition at line 166 of file Gamepad.cpp.
Vector2D get_mapped_coord | ( | ) |
Gets cartesian coordinates mapped to circular grid.
- Returns:
- a struct with x,y members, each in the range 0.0 to 1.0
Definition at line 308 of file Gamepad.cpp.
Polar get_polar | ( | ) |
Gets polar coordinates of the joystick.
- Returns:
- a struct contains mag and angle
Definition at line 321 of file Gamepad.cpp.
void init | ( | ) |
Initialise all peripherals and configure interrupts.
Definition at line 49 of file Gamepad.cpp.
void led | ( | int | n, |
float | val | ||
) | const |
Set LED to duty-cycle.
- Parameters:
-
led number (0 to 5) value in range 0.0 to 1.0
Definition at line 93 of file Gamepad.cpp.
void leds | ( | float | val ) | const |
Set all LEDs to duty-cycle.
- Parameters:
-
value in range 0.0 to 1.0
Definition at line 72 of file Gamepad.cpp.
void leds_off | ( | ) |
Turn all LEDs off.
Definition at line 62 of file Gamepad.cpp.
void leds_on | ( | ) |
Turn all LEDs on.
Definition at line 67 of file Gamepad.cpp.
float read_pot | ( | ) | const |
Read potentiometer.
- Returns:
- potentiometer value in range 0.0 to 1.0
Definition at line 129 of file Gamepad.cpp.
void reset_flags | ( | ) |
Reset all button flags.
Definition at line 152 of file Gamepad.cpp.
void tone | ( | float | frequency, |
float | duration | ||
) |
Play tone on piezo.
- Parameters:
-
frequency in Hz duration of tone in seconds
Definition at line 134 of file Gamepad.cpp.
Generated on Thu Jul 14 2022 01:22:29 by
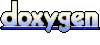