
class project main.cpp publish
Dependencies: mbed C12832_lcd USBHost USBHostPTP LCD_Menu
main.cpp
00001 /** 00002 * @file main.cpp 00003 * @brief Function to call USBHostPTP Library 00004 * @author Dwayne Dilbeck 00005 * @date 8/23/2013 00006 * 00007 * mbed USBHostPTP Library 00008 * 00009 * @par Copyright: 00010 * Copyright (c) 2013 Dwayne Dilbeck 00011 * @par License: 00012 * This software is distributed under the terms of the GNU Lesser General Public License 00013 */ 00014 #include "mbed.h" 00015 #include "C12832_lcd.h" 00016 #include "USBHostMSD.h" 00017 #include "USBHostPTP.h" 00018 00019 #include "PTPMenu.h" 00020 00021 00022 00023 /** 00024 * Initiate Global variables 00025 */ 00026 DigitalOut led(LED1); 00027 FILE * fp2; 00028 C12832_LCD lcd; 00029 USBHostPTP *ptpdev = NULL; 00030 USBHostMSD *msddev = NULL; 00031 char fname[256]; 00032 PTPMenu *menuobj = NULL; 00033 bool commandActive=false; 00034 void (*statusFunction)(void); 00035 00036 00037 bool CheckForCode(uint16_t code, char *buffer){ 00038 FILE *fp; 00039 struct { 00040 char str[60]; 00041 uint16_t code; 00042 } temp; 00043 bool found=false; 00044 00045 if( code < 0x9000) { 00046 fp = fopen("/usb2/PIMACODE.bin", "rb"); 00047 } else { 00048 fp = fopen("/usb2/canon.bin", "rb"); 00049 } 00050 if (fp != NULL) { 00051 while( !found && fread(&temp.code,sizeof(uint16_t),1,fp) >0 ) { 00052 fread(&temp.str,sizeof(char),60,fp); 00053 if (code == temp.code) { 00054 found=true; 00055 strcpy(buffer,temp.str); 00056 } 00057 } 00058 fclose(fp); 00059 } 00060 00061 return found; 00062 } 00063 /** 00064 * This function is used to handle the raw data recieved via the bulk pipes 00065 * 00066 * @param ptp Pointer to the PTP device 00067 * @param buffer Pointer to the data recieved 00068 * @param length Total data received to be processed 00069 * 00070 * @return Void 00071 */ 00072 void WriteObjectHandles(void *ptp,uint8_t *buffer,uint16_t length){ 00073 int writeResult,errorcode; 00074 uint16_t transferLength=length; 00075 uint8_t *dataPtr=buffer; 00076 00077 writeResult=fwrite(dataPtr,sizeof(uint8_t),transferLength,fp2); 00078 if( writeResult != transferLength) { 00079 errorcode=ferror(fp2); 00080 if( errorcode ) 00081 { 00082 printf("\r\nError in writing to file %d\n",errorcode); 00083 error("Yucky@!"); 00084 } 00085 } 00086 } 00087 00088 /** 00089 * Test function 0x01 00090 * 00091 * @param numberOfImages A pointer to where to write the images on the device 00092 * @param numberOfThumbs A pointer to where to write the images on the device 00093 * @return void 00094 */ 00095 void GetNumberOfThumbsAndImages(int *numberOfImages,int *numberOfThumbs){ 00096 uint32_t fileHandle=0,numImages=0; 00097 int internalNumberOfImages=0; 00098 int internalNumberOfThumbs=0; 00099 00100 FILE *fp = fopen("/usb2/objHandles.bin", "rb"); 00101 fread(&numImages,sizeof(uint32_t),1,fp); 00102 while(numImages>0){ 00103 fread(&fileHandle,sizeof(uint32_t),1,fp); 00104 ptpdev->GetObjectInfo(fileHandle); 00105 00106 if (ptpdev->objectInfo.thumbFormat == 0x3801 ) { 00107 internalNumberOfThumbs++; 00108 } 00109 if (ptpdev->objectInfo.objectFormat == 0x3801 ) { 00110 internalNumberOfImages++; 00111 } 00112 numImages--; 00113 } 00114 fclose(fp); 00115 *numberOfImages = internalNumberOfImages; 00116 *numberOfThumbs = internalNumberOfThumbs; 00117 00118 } 00119 00120 /** 00121 * Retrieve all thumbnail images and real images that are JPG files. 00122 */ 00123 void GetAllImagesAndThumbs(void) { 00124 FILE *fp; 00125 00126 uint32_t fileHandle=0,numImages=0; 00127 00128 printf("objectid,objectinfosize,imagetype,thumbtype\r\n"); 00129 fp = fopen("/usb2/objHandles.bin", "rb"); 00130 00131 fread(&numImages,sizeof(uint32_t),1,fp); 00132 while(numImages>0){ 00133 fread(&fileHandle,sizeof(uint32_t),1,fp); 00134 ptpdev->GetObjectInfo(fileHandle); 00135 printf("%ld,%x,%x,%x\r\n",numImages,fileHandle,ptpdev->objectInfo.objectFormat,ptpdev->objectInfo.thumbFormat); 00136 00137 if (ptpdev->objectInfo.thumbFormat == 0x3801 && numImages < 864 ) { 00138 sprintf(fname,"/usb2/thumb_%s",ptpdev->objectInfo.filename.getString()); 00139 fp2 = fopen(fname, "wb"); 00140 printf("Starting transfer of %s\r\n",fname); 00141 ptpdev->GetThumb(fileHandle,(void *)&WriteObjectHandles); 00142 fclose(fp2); 00143 printf("GetThumb Transaction Complete\r\n"); 00144 } 00145 00146 if (ptpdev->objectInfo.objectFormat == 0x3801 && numImages < 864 ) { 00147 sprintf(fname,"/usb2/%s",ptpdev->objectInfo.filename.getString()); 00148 printf("%s -Type: 0x%04x\r\n",fname,ptpdev->objectInfo.objectFormat); 00149 printf("Starting transfer of %s\r\n",fname); 00150 fp2 = fopen(fname, "wb"); 00151 ptpdev->GetObject(fileHandle,(void *)&WriteObjectHandles); 00152 fclose(fp2); 00153 printf("GetObject Transaction Complete\r\n"); 00154 } 00155 numImages--; 00156 } 00157 fclose(fp); 00158 } 00159 00160 /** 00161 * Thread to Watch for MSD image 00162 */ 00163 void msd_task(void const *) { 00164 USBHostMSD msd2("usb2"); 00165 00166 msddev=&msd2; 00167 while(true) { 00168 while(!msd2.connect()) { 00169 Thread::wait(500); 00170 } 00171 00172 Thread::wait(200); 00173 DIR *dp; 00174 struct dirent *ep; 00175 dp = opendir ("/usb2"); 00176 00177 if (dp != NULL) 00178 { 00179 ep = readdir (dp); 00180 while (ep!=NULL) { 00181 printf("%s\r\n",ep->d_name); 00182 ep = readdir (dp); 00183 } 00184 (void) closedir (dp); 00185 } 00186 00187 while(msd2.connect()) { 00188 Thread::wait(250); 00189 00190 } 00191 if(ptpdev!=NULL) 00192 ptpdev->CodeDecoderFunction=NULL; 00193 00194 } 00195 } 00196 00197 00198 void genericStatus(void) { 00199 led=!led; 00200 lcd.cls(); 00201 lcd.locate(10,0); 00202 lcd.printf("Please wait...."); 00203 } 00204 00205 void getAllObjectHandles(void) { 00206 fp2 = fopen("/usb2/objHandles.bin", "wb"); 00207 ptpdev->GetObjectHandles(0xffffffff,0x0000,0x0000,(void *)&WriteObjectHandles); 00208 fclose(fp2); 00209 printf("GetObjecthandles Transaction Complete\r\n"); 00210 } 00211 00212 00213 /** 00214 * Function to display status during image transfers 00215 */ 00216 void GetAllJPGStatus(void) { 00217 led=!led; 00218 lcd.cls(); 00219 if(ptpdev->dataLeftToTransfer>0) { 00220 lcd.locate(10,0); 00221 lcd.printf("%ld/%ld", ptpdev->dataLeftToTransfer, ptpdev->totalDataToTransfer); 00222 lcd.locate(0,10); 00223 lcd.printf("%s",fname); 00224 } 00225 } 00226 00227 /** 00228 * Thread to watch for the PTP device connected, and commands that need to be executed. 00229 */void ptp_task2(void const *) { 00230 00231 00232 USBHostPTP ptp; 00233 ptpdev=&ptp; 00234 00235 int numi,numt; 00236 uint32_t objCount=0; 00237 00238 while(true) { 00239 while(!ptpdev->connect()) { 00240 Thread::wait(500); 00241 } 00242 00243 while(!msddev->connected()){ 00244 Thread::wait(500); 00245 } 00246 00247 ptp.OpenSession(); 00248 printf("OpenSession Transaction Complete\r\n\r\n"); 00249 ptp.GetDeviceInfo(); 00250 printf("GetDeviceInfo Transaction Complete\r\n"); 00251 00252 while(ptpdev->connected()) { 00253 if(menuobj->command!=0x00) 00254 commandActive=true; 00255 switch(menuobj->command) { 00256 case GETALLJPG: 00257 statusFunction=&GetAllJPGStatus; 00258 getAllObjectHandles(); 00259 GetAllImagesAndThumbs(); 00260 break; 00261 case GETALLJPGTHUMB: 00262 break; 00263 case GETNUMJPG: 00264 getAllObjectHandles(); 00265 GetNumberOfThumbsAndImages(&numi,&numt); 00266 printf("images: %d, thumbs:%d\r\n",numi,numt); 00267 break; 00268 case GETNUMOBJ: 00269 ptp.GetNumObjects(&objCount); 00270 printf("GetNumObjects Transaction Complete - Count:%ld\r\n",objCount); 00271 break; 00272 case DUMPDEVICEINFO: 00273 statusFunction=&genericStatus; 00274 ptp.GetDeviceInfo(); 00275 ptp.DumpDeviceInfo(); 00276 break; 00277 case CAPTUREMODEON: 00278 statusFunction=&genericStatus; 00279 ptp.Operation(0x9008); 00280 ptp.GetDeviceInfo(); 00281 break; 00282 case CAPTUREMODEOFF: 00283 statusFunction=&genericStatus; 00284 ptp.Operation(0x9009); 00285 ptp.GetDeviceInfo(); 00286 break; 00287 case VFINDERON: 00288 ptp.Operation(0x900b); 00289 break; 00290 case VFINDEROFF: 00291 ptp.Operation(0x900c); 00292 break; 00293 case TAKEPHOTO: 00294 statusFunction=&genericStatus; 00295 printf("Set Prop: %x\r\n",ptp.SetDevicePropValue(0xd029,0xd)); 00296 printf("Focus Lock: %x\r\n",ptp.Operation(0x9014)); 00297 printf("Image Capture: %x\r\n",ptp.Operation(0x901a)); 00298 break; 00299 case CLOSESESSION: 00300 ptpdev->CloseSession(); 00301 printf("CloseSession Transaction Complete\r\n"); 00302 break; 00303 case ENABLEDECODER : 00304 if(msddev!=NULL) { 00305 ptpdev->CodeDecoderFunction=&CheckForCode; 00306 } 00307 printf("Decoder Enabled\r\n"); 00308 break; 00309 default: 00310 commandActive=false; 00311 break; 00312 } 00313 statusFunction=NULL; 00314 menuobj->command=0x00; 00315 Thread::wait(100); 00316 } 00317 } 00318 } 00319 00320 ///Main fuction to display the Status of the application. 00321 int main() { 00322 lcd.cls(); 00323 lcd.locate(10,3); 00324 lcd.printf("Initializing"); 00325 Thread ptpTask2(ptp_task2, NULL, osPriorityNormal, 1024 * 4); 00326 Thread msdTask2(msd_task, NULL, osPriorityNormal, 1024 * 4); 00327 menuobj = new PTPMenu(&lcd); 00328 00329 while(1) { 00330 if(ptpdev!=NULL) { 00331 if(ptpdev->connected()) { 00332 if(!commandActive) { 00333 menuobj->poll(); 00334 } else { 00335 if(statusFunction!=NULL) 00336 (*statusFunction)(); 00337 } 00338 } else { 00339 lcd.cls(); 00340 lcd.locate(10,3); 00341 lcd.printf("Please connect PTP device."); 00342 } 00343 } 00344 Thread::wait(20); 00345 } 00346 } 00347
Generated on Thu Jul 14 2022 07:15:49 by
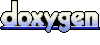