
Homework #1
Fork of BusOut_HelloWorld by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 //Load mbed basic function header 00002 #include "mbed.h" 00003 00004 //Define the LEDs as a BUS 00005 BusOut myleds(LED1, LED2, LED3, LED4); 00006 DigitalIn updown(p14); 00007 00008 //Define a global count variable 00009 int cnt=0; 00010 bool direction=false; 00011 00012 00013 //Define a generic increment by X function. The functions to increment is passed as a pointer. The increment amount is passed by value to the function 00014 void IncrementValueByX(int *value, int x) { 00015 *value+=x; 00016 } 00017 00018 //Define a generic wait function using the milliseconds, wait time is passed by value. 00019 void WaitForTimeInMilliSeconds(int mseconds) { 00020 wait_ms(mseconds); 00021 } 00022 00023 //Define a generic function to assign a nibble to a bus. The BUS is passed by reference and the value is passed 00024 // by value. 00025 void SetNibbleBusOut(BusOut *nibble,int value) { 00026 nibble->write(value & 0xF); 00027 } 00028 00029 //Determine if a button has been released 00030 bool CheckButtonRelease(int *buttonState,DigitalIn *inputPin) { 00031 int currentButton; 00032 bool retValue; 00033 00034 currentButton=inputPin->read(); 00035 if( *buttonState != currentButton && currentButton == 0) { 00036 retValue=true; 00037 } else { 00038 retValue=false; 00039 } 00040 *buttonState=currentButton; 00041 return retValue; 00042 } 00043 00044 //Invert a boolean based on a boolean value. 00045 void InvertBoolBasedonValue(bool *direction, int buttonRelease){ 00046 if(buttonRelease) 00047 *direction= !*direction; 00048 } 00049 00050 //Change a boolean value based on button release 00051 void ChangeDirectionOnButtonRelease(bool *direction, DigitalIn *inputPin){ 00052 bool buttonRelease=0; 00053 static int buttonState; 00054 00055 buttonRelease=CheckButtonRelease(&buttonState,inputPin); 00056 InvertBoolBasedonValue(direction,buttonRelease); 00057 } 00058 00059 00060 //Main loop 00061 int main() { 00062 while(true) { 00063 ChangeDirectionOnButtonRelease(&direction,&updown); 00064 if(direction) { 00065 IncrementValueByX(&cnt,1); 00066 } else { 00067 IncrementValueByX(&cnt,-1); 00068 } 00069 SetNibbleBusOut(&myleds,cnt); 00070 WaitForTimeInMilliSeconds(1000); 00071 } 00072 }
Generated on Sun Jul 31 2022 09:35:46 by
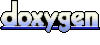