
A web server for monitoring and controlling a MakerBot Replicator over the USB host and ethernet.
Dependencies: IAP NTPClient RTC mbed-rtos mbed Socket lwip-sys lwip BurstSPI
Fork of LPC1768_Mini-DK by
makerbot.h
00001 // Copyright (c) 2013, jake (at) allaboutjake (dot) com 00002 // All rights reserved. 00003 // 00004 // Redistribution and use in source and binary forms, with or without 00005 // modification, are permitted provided that the following conditions are met: 00006 // * Redistributions of source code must retain the above copyright 00007 // notice, this list of conditions and the following disclaimer. 00008 // * Redistributions in binary form must reproduce the above copyright 00009 // notice, this list of conditions and the following disclaimer in the 00010 // documentation and/or other materials provided with the distribution. 00011 // * The name of the author and/or copyright holder nor the 00012 // names of its contributors may be used to endorse or promote products 00013 // derived from this software without specific prior written permission. 00014 // 00015 // THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 00016 // ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 00017 // WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00018 // DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER, AUTHOR, OR ANY CONTRIBUTORS 00019 // BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR 00020 // CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE 00021 // GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) 00022 // HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT 00023 // LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT 00024 // OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00025 00026 // DESCRIPTION OF FILE: 00027 // 00028 // This is the associated header file and contains the declaration of a C++ 00029 // object for communicating with a Makerbot over a USB host serial. 00030 // 00031 00032 #include "mbed.h" 00033 #include "USBHostSerial.h" 00034 #include "rtos.h" 00035 00036 #ifndef __MAKERBOT_H__ 00037 #define __MAKERBOT_H__ 00038 00039 typedef unsigned char uint8_t; 00040 00041 #define HEADER 0xD5 00042 #define MAXIMUM_PAYLOAD_LENGTH 32 00043 #define MAXIMUM_PACKET_LENGTH (MAXIMUM_PAYLOAD_LENGTH+3) 00044 00045 // Response Codes 00046 #define HEADER_NOT_FOUND 0xFF 00047 #define GENERIC_PACKET_ERROR 0x80 00048 #define SUCCESS 0x81 00049 #define ACTION_BUFFER_OVERFLOW 0x82 00050 #define CRC_MISMATCH 0x83 00051 #define PACKET_TOO_BIG 0x84 00052 #define COMMAND_NOT_SUPPORTED 0x85 00053 #define DOWNSTREAM_TIMEOUT 0x87 00054 #define TOOL_LOCK_TIMEOUT 0x88 00055 #define CANCEL_BUILD 0x89 00056 #define ACTIVE_LOCAL_BUILD 0x8A 00057 #define OVERHEAT_STATE 0x8B 00058 00059 // EEPROM Offsets 00060 #define MACHINE_NAME_OFFSET 0x0022 00061 #define TOOL_COUNT_OFFSET 0x0042 00062 #define HBP_PRESENT_OFFSET 0x004C 00063 00064 const uint8_t crctab[] = { 00065 0, 94, 188, 226, 97, 63, 221, 131, 194, 156, 126, 32, 163, 253, 31, 65, 00066 157, 195, 33, 127, 252, 162, 64, 30, 95, 1, 227, 189, 62, 96, 130, 220, 00067 35, 125, 159, 193, 66, 28, 254, 160, 225, 191, 93, 3, 128, 222, 60, 98, 00068 190, 224, 2, 92, 223, 129, 99, 61, 124, 34, 192, 158, 29, 67, 161, 255, 00069 70, 24, 250, 164, 39, 121, 155, 197, 132, 218, 56, 102, 229, 187, 89, 7, 00070 219, 133, 103, 57, 186, 228, 6, 88, 25, 71, 165, 251, 120, 38, 196, 154, 00071 101, 59, 217, 135, 4, 90, 184, 230, 167, 249, 27, 69, 198, 152, 122, 36, 00072 248, 166, 68, 26, 153, 199, 37, 123, 58, 100, 134, 216, 91, 5, 231, 185, 00073 140, 210, 48, 110, 237, 179, 81, 15, 78, 16, 242, 172, 47, 113, 147, 205, 00074 17, 79, 173, 243, 112, 46, 204, 146, 211, 141, 111, 49, 178, 236, 14, 80, 00075 175, 241, 19, 77, 206, 144, 114, 44, 109, 51, 209, 143, 12, 82, 176, 238, 00076 50, 108, 142, 208, 83, 13, 239, 177, 240, 174, 76, 18, 145, 207, 45, 115, 00077 202, 148, 118, 40, 171, 245, 23, 73, 8, 86, 180, 234, 105, 55, 213, 139, 00078 87, 9, 235, 181, 54, 104, 138, 212, 149, 203, 41, 119, 244, 170, 72, 22, 00079 233, 183, 85, 11, 136, 214, 52, 106, 43, 117, 151, 201, 74, 20, 246, 168, 00080 116, 42, 200, 150, 21, 75, 169, 247, 182, 232, 10, 84, 215, 137, 107, 53 00081 }; 00082 00083 00084 class Makerbot { 00085 00086 public: 00087 00088 enum LCDOptions { 00089 CLEAR_EXISTING_MESSAGE = 0x01, 00090 LAST_MESSAGE_IN_GROUP = 0x02, 00091 WAIT_FOR_BUTTON_PRESS = 0x04, 00092 }; 00093 00094 enum BuildState { 00095 NO_BUILD = 0, 00096 BUILD_RUNNING = 1, 00097 BUILD_FINISHED_NORMALLY = 2, 00098 BUILD_PAUSED = 3, 00099 BUILD_CANCELLED = 4, 00100 BUILD_SLEEPING = 5, 00101 BUILD_STATE_ERROR = 0xFF, 00102 }; 00103 00104 typedef struct { 00105 uint8_t build_state; 00106 uint8_t hours; 00107 uint8_t minutes; 00108 uint32_t line; 00109 uint32_t reserved; 00110 } __attribute__((packed, aligned(1))) MakerbotBuildState; 00111 00112 // Constructor / Destructor 00113 Makerbot(USBHostSerial* serial); 00114 ~Makerbot(); 00115 00116 int displayMessageToLCD(uint8_t options, uint8_t xPos, uint8_t yPos, uint8_t timeout, char* message); 00117 float getMakerbotVersion(); 00118 char* getMachineName(); 00119 int playbackCapture(char* filename); 00120 int pauseResume(); 00121 int abortImmediately(); 00122 int getToolCount(); 00123 int getToolTemperature(uint8_t toolIndex); 00124 int getToolSetPoint(uint8_t toolIndex); 00125 int getPlatformTemperature(uint8_t toolIndex); 00126 int getPlatformSetPoint(uint8_t toolIndex); 00127 char* getBuildName(); 00128 int hasPlatform(); 00129 MakerbotBuildState getBuildState(); 00130 00131 void flushInputChannel(); 00132 00133 static char* humanReadableBuildState(Makerbot::MakerbotBuildState state); 00134 00135 private: 00136 uint8_t CalculateCRC(uint8_t* data, int length); 00137 int getEEPROMByte(uint16_t offset); 00138 00139 int send_packet(uint8_t* payload, int length); 00140 int recv_packet(uint8_t* payload, int buffer_length); 00141 00142 USBHostSerial* serial; 00143 Mutex mutex; 00144 00145 char* machineName; 00146 float machineVersion; 00147 char* buildName; 00148 00149 }; 00150 00151 00152 #define DBG_INFO(x, ...) std::printf("[DBG_INFO: %s:%d]"x"\r\n", __FILE__, __LINE__, ##__VA_ARGS__); 00153 00154 #endif
Generated on Tue Jul 12 2022 17:52:03 by
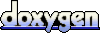